前言
因為工作需要有時候要畫雷達圖,但是數據好多組怎么辦?不能一個一個點excel去畫吧,那么可以利用python進行批量制作,得到樣式如下:
首先制作一個演示的excel,評分為excel隨機數生成:
1 =INT((RAND()+4)*10)/10
加入標簽等得到的excel樣式如下(部分,共計32行):
那么接下來就是打開python寫碼了,本文是基于
pycharm
進行編寫
?
wb = load_workbook(filename=r'C:\Users\Administrator\Desktop\數據指標.xlsx') ##讀取路徑 ws = wb.get_sheet_by_name("Sheet1") ##讀取名字為Sheet1的sheet表 info_id = [] info_first = [] for row_A in range(2, 32): ## 遍歷第2行到32行 id = ws.cell(row=row_A, column=1).value ## 遍歷第2行到32行,第1列 info_id.append(id) for col in range(2, 9): ##讀取第1到9列 first = ws.cell(row=1, column=col).value info_first.append(first) ##得到1到8列的標簽 info_data = [] for row_num_BtoU in range(2, len(info_id) + 2): ## 遍歷第2行到32行 row_empty = [] ##建立一個空數組作為臨時儲存地,每次換行就被清空 for i in range(2, 9): ## 遍歷第2行到32行,第2到9列 data_excel = ws.cell(row=row_num_BtoU, column=i).value if data_excel == None: pass else: row_empty.append(data_excel) ##將單元格信息儲存進去 info_data.append(row_empty)
分步講解:
讀取excel表格:
wb = load_workbook(filename=r'C:\Users\Administrator\Desktop\數據指標.xlsx') ##讀取路徑 ws = wb.get_sheet_by_name("Sheet1") ##讀取名字為Sheet1的sheet表
需要用到庫:
?import xlsxwriter
?from openpyxl import load_workbook
在命令指示符下輸入:
?pip install xlsxwriter
等待安裝即可,后面的庫也是如此:
將第一列ID儲存,以及第一行的標簽,標簽下面的數值分別儲存在:
info_id = [] info_first = [] info_data = []
讀取數據后接下來需要設置寫入的格式:
workbook = xlsxwriter.Workbook('C:\\Users\\Administrator\\Desktop\\result.xlsx') worksheet = workbook.add_worksheet() # 創建一個工作表對象 #字體格式 font = workbook.add_format( {'border': 1, 'align': 'center', 'font_size': 11, 'font_name': '微軟雅黑'}) ##字體居中,11號,微軟雅黑,給一般的信息用的 #寫下第一行第一列的標簽 worksheet.write(0, 0, '商品貨號', font) ##設置圖片的那一列寬度 worksheet.set_column(0, len(info_first) + 1, 11) # 設定第len(info_first) + 1列的寬度為11
將標簽數據等寫入新的excel表格中:
#新建一個excel保存結果 workbook = xlsxwriter.Workbook('C:\\Users\\Administrator\\Desktop\\result.xlsx') worksheet = workbook.add_worksheet() # 創建一個工作表對象 #字體格式 font = workbook.add_format( {'border': 1, 'align': 'center', 'font_size': 11, 'font_name': '微軟雅黑'}) ##字體居中,11號,微軟雅黑,給一般的信息用的 #寫下第一行第一列的標簽 worksheet.write(0, 0, '商品貨號', font) ##設置圖片的那一列寬度 worksheet.set_column(0, len(info_first) + 1, 11) # 設定第len(info_first) + 1列的寬度為11 ##寫入標簽 for k in range(0,7): worksheet.write(0, k + 1, info_first[k], font) #寫入最后一列標簽 worksheet.write(0, len(info_first) + 1, '雷達圖', font)
制作雷達圖:
#設置雷達各個頂點的名稱 labels = np.array(info_first) #數據個數 data_len = len(info_first) for i in range(0,len(info_id)): data = np.array(info_data[i]) angles = np.linspace(0, 2*np.pi, data_len, endpoint=False) data = np.concatenate((data, [data[0]])) # 閉合 angles = np.concatenate((angles, [angles[0]])) # 閉合 fig = plt.figure() ax = fig.add_subplot(111, polar=True)# polar參數!! ax.plot(angles, data, 'bo-', linewidth=2)# 畫線 ax.fill(angles, data, facecolor='r', alpha=0.25)# 填充 ax.set_thetagrids(angles * 180/np.pi, labels, fontproperties="SimHei") ax.set_title("商品貨號:" + str(info_id[i]), va='bottom', fontproperties="SimHei") ax.set_rlim(3.8,5)# 設置雷達圖的范圍 ax.grid(True) plt.savefig("C:\\Users\\Administrator\\Desktop\\result\\商品貨號:" + str(info_id[i]) + ".png", dpi=120)
圖片太大怎么辦? 用庫改變大小即可 :
import Image ##更改圖片大小 infile = “C:\\Users\\Administrator\\Desktop\\result\\商品貨號:" + str(info_id[i]) + ".png“ outfile = ”C:\\Users\\Administrator\\Desktop\\result1\\商品貨號:" + str(info_id[i]) + ".png” im = Image.open(infile) (x, y) = im.size x_s = 80 ## 設置長 y_s = 100 ## 設置寬 out = im.resize((x_s, y_s), Image.ANTIALIAS) out.save(outfile,'png',quality = 95)
將大圖片和小圖片放在了 result和result1 兩個不同的文件夾,需要再前邊創建這兩個文件夾:
if os.path.exists(r'C:\\Users\\Administrator\\Desktop\\result'): # 建立一個文件夾在桌面,文件夾為result print('result文件夾已經在桌面存在,繼續運行程序……') else: print('result文件夾不在桌面,新建文件夾result') os.mkdir(r'C:\\Users\\Administrator\\Desktop\\result') print('文件夾建立成功,繼續運行程序') if os.path.exists(r'C:\\Users\\Administrator\\Desktop\\result1'): # 建立一個文件夾在C盤,文件夾為result1 print('result1文件夾已經在桌面存在,繼續運行程序……') else: print('result1文件夾不在桌面,新建文件夾result1') os.mkdir(r'C:\\Users\\Administrator\\Desktop\\result1') print('文件夾建立成功,繼續運行程序')
最后插入圖片到excel中:
worksheet.insert_image(i + 1, len(info_first) + 1, 'C:\\Users\\Administrator\\Desktop\\result1\\' + "商品貨號:" + str(info_id[i]) + '.png') ##寫入圖片 time.sleep(1)##防止寫入太快電腦死機 plt.close() # 一定要關掉圖片,不然python打開圖片20個后會崩潰 workbook.close()#最后關閉excel
得到的效果如下:
附上完整代碼:
import numpy as np import matplotlib.pyplot as plt import xlsxwriter from openpyxl import load_workbook import os import time from PIL import Image if __name__ == '__main__': if os.path.exists(r'C:\\Users\\Administrator\\Desktop\\result'): # 建立一個文件夾在桌面,文件夾為result print('result文件夾已經在桌面存在,繼續運行程序……') else: print('result文件夾不在桌面,新建文件夾result') os.mkdir(r'C:\\Users\\Administrator\\Desktop\\result') print('文件夾建立成功,繼續運行程序') if os.path.exists(r'C:\\Users\\Administrator\\Desktop\\result1'): # 建立一個文件夾在C盤,文件夾為result1 print('result1文件夾已經在桌面存在,繼續運行程序……') else: print('result1文件夾不在桌面,新建文件夾result1') os.mkdir(r'C:\\Users\\Administrator\\Desktop\\result1') print('文件夾建立成功,繼續運行程序') wb = load_workbook(filename=r'C:\Users\Administrator\Desktop\數據指標.xlsx') ##讀取路徑 ws = wb.get_sheet_by_name("Sheet1") ##讀取名字為Sheet1的sheet表 info_id = [] info_first = [] for row_A in range(2, 32): ## 遍歷第2行到32行 id = ws.cell(row=row_A, column=1).value ## 遍歷第2行到32行,第1列 info_id.append(id) for col in range(2, 9): ##讀取第1到9列 first = ws.cell(row=1, column=col).value info_first.append(first) ##得到1到8列的標簽 print(info_id) print(info_first) info_data = [] for row_num_BtoU in range(2, len(info_id) + 2): ## 遍歷第2行到32行 row_empty = [] ##建立一個空數組作為臨時儲存地,每次換行就被清空 for i in range(2, 9): ## 遍歷第2行到32行,第2到9列 data_excel = ws.cell(row=row_num_BtoU, column=i).value if data_excel == None: pass else: row_empty.append(data_excel) ##將單元格信息儲存進去 info_data.append(row_empty) print(info_data) print(len(info_data)) # 設置雷達各個頂點的名稱 labels = np.array(info_first) # 數據個數 data_len = len(info_first) # 新建一個excel保存結果 workbook = xlsxwriter.Workbook('C:\\Users\\Administrator\\Desktop\\result.xlsx') worksheet = workbook.add_worksheet() # 創建一個工作表對象 # 字體格式 font = workbook.add_format( {'border': 1, 'align': 'center', 'font_size': 11, 'font_name': '微軟雅黑'}) ##字體居中,11號,微軟雅黑,給一般的信息用的 # 寫下第一行第一列的標簽 worksheet.write(0, 0, '商品貨號', font) ##設置圖片的那一列寬度 worksheet.set_column(0, len(info_first) + 1, 11) # 設定第len(info_first) + 1列的寬度為11 ##寫入標簽 for k in range(0, 7): worksheet.write(0, k + 1, info_first[k], font) # 寫入最后一列標簽 worksheet.write(0, len(info_first) + 1, '雷達圖', font) # 將其他參數寫入excel中 for j in range(0, len(info_id)): worksheet.write(j + 1, 0, info_id[j], font) # 寫入商品貨號 worksheet.set_row(j, 76) ##設置行寬 for x in range(0, len(info_first)): worksheet.write(j + 1, x + 1, info_data[j][x], font) # 寫入商品的其他參數 for i in range(0, len(info_id)): data = np.array(info_data[i]) angles = np.linspace(0, 2 * np.pi, data_len, endpoint=False) data = np.concatenate((data, [data[0]])) # 閉合 angles = np.concatenate((angles, [angles[0]])) # 閉合 fig = plt.figure() ax = fig.add_subplot(111, polar=True) # polar參數!! ax.plot(angles, data, 'bo-', linewidth=2) # 畫線 ax.fill(angles, data, facecolor='r', alpha=0.25) # 填充 ax.set_thetagrids(angles * 180 / np.pi, labels, fontproperties="SimHei") ax.set_title("商品貨號:" + str(info_id[i]), va='bottom', fontproperties="SimHei") ax.set_rlim(3.8, 5) # 設置雷達圖的范圍 ax.grid(True) plt.savefig("C:\\Users\\Administrator\\Desktop\\result\\商品貨號:" + str(info_id[i]) + ".png", dpi=120) # plt.show()在python中顯示 ##更改圖片大小 infile = "C:\\Users\\Administrator\\Desktop\\result\\商品貨號:" + str(info_id[i]) + ".png" outfile = "C:\\Users\\Administrator\\Desktop\\result1\\商品貨號:" + str(info_id[i]) + ".png" im = Image.open(infile) (x, y) = im.size x_s = 80 ## 設置長 y_s = 100 ## 設置寬 out = im.resize((x_s, y_s), Image.ANTIALIAS) out.save(outfile, 'png', quality=95) worksheet.insert_image(i + 1, len(info_first) + 1, 'C:\\Users\\Administrator\\Desktop\\result1\\' + "商品貨號:" + str( info_id[i]) + '.png') ##寫入圖片 time.sleep(1) ##防止寫入太快電腦死機 plt.close() # 一定要關掉圖片,不然python打開圖片20個后會崩潰 workbook.close() # 最后關閉excel
以上就是本文介紹利用python批量制作雷達圖的實現方法,希望給學習python的大家有所幫助
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
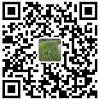
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
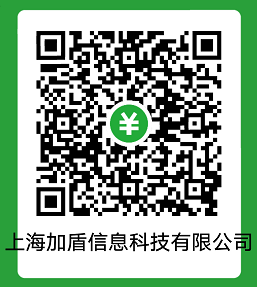