合成模式有時又叫做部分-整體模式(Part-Whole)。合成模式將對象組織到樹結構中,可以用來描述整體與部分的關系。合成模式可以使客戶端將單純元素與復合元素同等看待。
合成模式的實現根據所實現接口的區別分為兩種形式,分別稱為安全模式和透明模式。
透明方式
作為第一種選擇,在Component里面聲明所有的用來管理子類對象的方法,包括add()、remove(),以及getChild()方法。這樣做的好處是所有的構件類都有相同的接口。在客戶端看來,樹葉類對象與合成類對象的區別起碼在接口層次上消失了,客戶端可以同等同的對待所有的對象。這就是透明形式的合成模式。
這個選擇的缺點是不夠安全,因為樹葉類對象和合成類對象在本質上是有區別的。樹葉類對象不可能有下一個層次的對象,因此add()、remove()以及getChild()方法沒有意義,是在編譯時期不會出錯,而只會在運行時期才會出錯。
安全方式
第二種選擇是在Composite類里面聲明所有的用來管理子類對象的方法。這樣的做法是安全的做法,因為樹葉類型的對象根本就沒有管理子類對象的方法,因此,如果客戶端對樹葉類對象使用這些方法時,程序會在編譯時期出錯。
這個選擇的缺點是不夠透明,因為樹葉類和合成類將具有不同的接口。
這兩個形式各有優缺點,需要根據軟件的具體情況做出取舍決定。
?
一,安全式結構
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
抽象構件(Component)角色: 這是一個抽象角色,它給參加組合的對象定義出公共的接口及其默認行為,可以用來管理所有的子對象。在安全式的合成模式里,構件角色并不是定義出管理子對象的方法,這一定義由樹枝構件對象給出。
樹葉構件(Leaf)角色: 樹葉對象是沒有下級子對象的對象,定義出參加組合的原始對象的行為。
樹枝構件(Composite)角色: 代表參加組合的有下級子對象的對象。樹枝對象給出所有的管理子對象的方法,如add()、remove()、getChild()等。
二,安全式示例代碼
import java.util.ArrayList; /** * 抽象構件 * @author Salmon * */ public abstract class Component { protected String name; public Component(String name) { this.name = name; } public abstract void display(int depth); } /** * 樹枝構件 * @author Salmon * */ public class Composite extends Component { private ArrayList<Component> children = new ArrayList<Component>(); public Composite(String name) { super(name); } public void add(Component component) { children.add(component); } public void remove(Component component) { children.remove(component); } public void display(int depth) { for (Component component : children) { component.display(depth + 2); } } } /** * 樹葉構件 * @author Salmon * */ public class Leaf extends Component { public Leaf(String name) { super(name); } public void display(int depth) { System.out.println(""); } } /** * 客戶端代碼 * @author Salmon * */ public class Client { public void main() { Composite root = new Composite("root"); root.add(new Leaf("Leaf A")); root.add(new Leaf("Leaf B")); Composite comp = new Composite("Composite X"); comp.add(new Leaf("Leaf XA")); comp.add(new Leaf("Leaf XB")); root.add(comp); root.add(new Leaf("Leaf C")); // Add and remove a leaf Leaf l = new Leaf("Leaf D"); root.add(l); root.remove(l); // Recursively display nodes root.display(1); } }
?
三,透明式結構
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
抽象構件(Component)角色: 這是一個抽象角色,它給參加組合的對象規定一個接口,規范共有的接口及默認行為。
樹葉構件(Leaf)角色: 代表參加組合的樹葉對象,定義出參加組合的原始對象的行為。樹葉類會給出add()、remove()以及getChild()之類的用來管理子類對對象的方法的平庸實現。
樹枝構件(Composite)角色: 代表參加組合的有子對象的對象,定義出這樣的對象的行為。
四,透明式示例代碼
import java.util.ArrayList; /** * 抽象構件 * @author Salmon * */ public abstract class Component { protected String name; public Component(String name) { this.name = name; } public abstract void add(Component c); public abstract void remove(Component c); public abstract void display(int depth); } /** * 樹枝構件 * @author Salmon * */ public class Composite extends Component { private ArrayList<Component> children = new ArrayList<Component>(); public Composite(String name) { super(name); } public void add(Component component) { children.add(component); } public void remove(Component component) { children.remove(component); } public void display(int depth) { System.out.println(""); ; // Display each of the node's children for (Component component : children) component.display(depth + 2); } } /** * 樹葉構件 * @author Salmon * */ class Leaf extends Component { public Leaf(String name) { super(name); } public void add(Component c) { System.out.println("Cannot add to a leaf"); } public void remove(Component c) { System.out.println("Cannot remove from a leaf"); } public void display(int depth) { System.out.println(""); } } /** * 客戶端代碼 * @author Salmon * */ public class Client { public static void main(String[] args) { // Create a tree structure Composite root = new Composite("root"); root.add(new Leaf("Leaf A")); root.add(new Leaf("Leaf B")); Composite comp = new Composite("Composite X"); comp.add(new Leaf("Leaf XA")); comp.add(new Leaf("Leaf XB")); root.add(comp); root.add(new Leaf("Leaf C")); Leaf l = new Leaf("Leaf D"); root.add(l); root.remove(l); root.display(1); } }
?
?
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
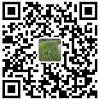
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
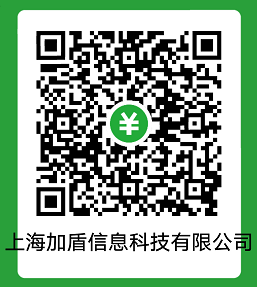