一 什么是Service
二 如何使用Service
三
Service
的生命周期
一 什么是 Service
Service
,看名字就知道跟正常理解的“服務(wù)”差不多,后臺運行,可交互這樣的一個東西。它跟Activity的級別差不多,也需要在配置文件里注冊,但是他不能自己運行,需要通過某一個Activity或者其他Context對象來調(diào)用, Context.startService() 和 Context.bindService()。
兩種啟動
Service
的方式有所不同。這里要說明一下的是如果你在
Service
的onCreate或者onStart做一些很耗時間的事情,最好在
Service
里啟動一個線程來完成,因為
Service
是跑在主線程中,會影響到你的UI操作或者阻塞主線程中的其他事情。
什么時候需要
Service
呢?比如播放多媒體的時候用戶啟動了其他Activity這個時候程序要在后臺繼續(xù)播放,比如檢測SD卡上文件的變化,再或者在后臺記錄你地理信息位置的改變等等,總之服務(wù)嘛,總是藏在后頭的。
二 如何使用 Service
那接下來用代碼來說明一下怎么使用
Service
,這里我們要講的是Local
Service
也就是你自己的一個
Service
, 你也可以操作別的應(yīng)用程序的
service
如果它允許你那么去做的話,這就設(shè)計到一個比較麻煩的東西interprocess communication (IPC),在不同的進程中通信的機制,這個我自己也還沒有用過,等用了以后再跟大伙說說,通常情況下Local的就夠用啦。
跟Activity一樣首先你要寫一個類繼承自
android
.app.
Service
,在這里我叫他TestService
代碼如下:
package jason.tutorial; import android.app.Notification; import android.app.NotificationManager; import android.app.PendingIntent; import android.app.Service; import android.content.Intent; import android.os.Binder; import android.os.IBinder; import android.util.Log; public class TestService extends Service { private static final String TAG = "TestService"; private NotificationManager nm; @Override public IBinder onBind(Intent i) { Log.e(TAG, "============> TestService.onBind"); return null; } public class LocalBinder extends Binder { TestService getService() { return TestService.this; } } @Override public boolean onUnbind(Intent i) { Log.e(TAG, "============> TestService.onUnbind"); return false; } @Override public void onRebind(Intent i) { Log.e(TAG, "============> TestService.onRebind"); } @Override public void onCreate() { Log.e(TAG, "============> TestService.onCreate"); nm = (NotificationManager) getSystemService(NOTIFICATION_SERVICE); showNotification(); } @Override public void onStart(Intent intent, int startId) { Log.e(TAG, "============> TestService.onStart"); } @Override public void onDestroy() { nm.cancel(R.string.service_started); Log.e(TAG, "============> TestService.onDestroy"); } private void showNotification() { Notification notification = new Notification(R.drawable.face_1, "Service started", System.currentTimeMillis()); PendingIntent contentIntent = PendingIntent.getActivity(this, 0, new Intent(this, TestServiceHolder.class), 0); // must set this for content view, or will throw a exception notification.setLatestEventInfo(this, "Test Service", "Service started", contentIntent); nm.notify(R.string.service_started, notification); } }
其中用到Notification是為了明顯地表明 Service 存活的狀態(tài),這樣看上去直觀一點,更多關(guān)于Notification的內(nèi)容請參考前面的文章.
public class LocalBinder extends Binder { TestService getService() { return TestService.this; } }
這個方法是為了讓調(diào)用者得到這個
Service
并操作它。
Service
本身就這樣簡單了,你需要做什么就在onCreate和onStart里做好了,起個線程什么的。
再看一下它的調(diào)用者,TestServiceHolder
package jason.tutorial; import android.app.Activity; import android.content.ComponentName; import android.content.Context; import android.content.Intent; import android.content.ServiceConnection; import android.os.Bundle; import android.os.IBinder; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.Toast; public class TestServiceHolder extends Activity { private boolean isBound; private TestService boundService; public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.test_service_holder); setTitle("Service Test"); initButtons(); } private ServiceConnection connection = new ServiceConnection() { public void onServiceConnected(ComponentName className, IBinder service) { boundService = ((TestService.LocalBinder)service).getService(); Toast.makeText(TestServiceHolder.this, "Service connected", Toast.LENGTH_SHORT).show(); } public void onServiceDisconnected(ComponentName className) { // unexpectedly disconnected,we should never see this happen. boundService = null; Toast.makeText(TestServiceHolder.this, "Service disconnected", Toast.LENGTH_SHORT).show(); } }; private void initButtons() { Button buttonStart = (Button) findViewById(R.id.start_service); buttonStart.setOnClickListener(new OnClickListener() { public void onClick(View arg0) { startService(); } }); Button buttonStop = (Button) findViewById(R.id.stop_service); buttonStop.setOnClickListener(new OnClickListener() { public void onClick(View arg0) { stopService(); } }); Button buttonBind = (Button) findViewById(R.id.bind_service); buttonBind.setOnClickListener(new OnClickListener() { public void onClick(View arg0) { bindService(); } }); Button buttonUnbind = (Button) findViewById(R.id.unbind_service); buttonUnbind.setOnClickListener(new OnClickListener() { public void onClick(View arg0) { unbindService(); } }); } private void startService() { Intent i = new Intent(this, TestService.class); this.startService(i); } private void stopService() { Intent i = new Intent(this, TestService.class); this.stopService(i); } private void bindService() { Intent i = new Intent(this, TestService.class); bindService(i, connection, Context.BIND_AUTO_CREATE); isBound = true; } private void unbindService() { if (isBound) { unbindService(_connection); isBound = false; } } }這里可以看到兩種啟動方法,start和bind,當然都是通過intent調(diào)用的,在intent中指明要啟動的 Service 的名字,stop也一樣
private void startService() { Intent i = new Intent(this, TestService.class); this.startService(i); } private void stopService() { Intent i = new Intent(this, TestService.class); this.stopService(i); }
對于bind的話,需要一個ServiceConnection對象
private ServiceConnection connection = new ServiceConnection() { public void onServiceConnected(ComponentName className, IBinder service) { boundService = ((TestService.LocalBinder)service).getService(); Toast.makeText(TestServiceHolder.this, "Service connected", Toast.LENGTH_SHORT).show(); } public void onServiceDisconnected(ComponentName className) { // unexpectedly disconnected,we should never see this happen. boundService = null; Toast.makeText(TestServiceHolder.this, "Service disconnected", Toast.LENGTH_SHORT).show(); } };
用來把Activity和特定的
Service
連接在一起,共同存亡,具體的生命周期細節(jié)下一段來講。
三 Service的生命周期
Service
的生命周期方法比Activity少一些,只有onCreate, onStart, onDestroy
我們有兩種方式啟動一個
Service
,他們對
Service
生命周期的影響是不一樣的。
1 通過startService
Service
會經(jīng)歷 onCreate -> onStart, stopService的時候直接onDestroy
如果是調(diào)用者(TestServiceHolder)自己直接退出而沒有調(diào)用stopService的話,
Service
會一直在后臺運行。
下次TestServiceHolder再起來可以stopService。
2 通過bindService
Service
只會運行onCreate, 然后會有onBind,這個時候 TestServiceHolder 和TestService綁定在一起
TestServiceHolder 退出了,Srevice就會調(diào)用onUnbind->onDestroyed ,所謂綁定在一起就共存亡了。
那有同學(xué)問了,要是這幾個方法交織在一起的話,會出現(xiàn)什么情況呢?
一個原則是
Service
的onCreate的方法只會被調(diào)用一次,就是你無論多少次的startService又 bindService,
Service
只被創(chuàng)建一次。如果先是bind了,那么start的時候就直接運行
Service
的onStart方法,如果先 是start,那么bind的時候就直接運行onBind方法。如果你先bind上了,就stop不掉了,對啊,就是stopService不好使了,只 能先UnbindService, 再StopService,所以是先start還是先bind行為是有區(qū)別的。
大家有興趣可以回去點點按鈕看看log,多看幾遍log就知道了。
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
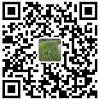
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
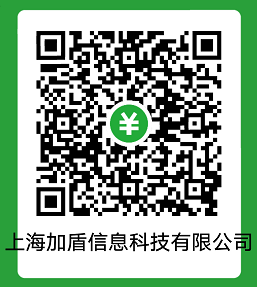