這是在Linux下面最常用的一個統一的鏈表結構,Linux就是用這個結構將所有的Driver、Device什么的都分別串在一起。我覺得寫得非常好,大家來看一看。
-----------------------------------------------------------------------------------------------------
#ifndef _LINUX_LIST_H
#define _LINUX_LIST_H
#ifdef __KERNEL__
struct list_head {
struct list_head *next, *prev;
};
#define LIST_HEAD_INIT(name) { &(name), &(name) }
#define LIST_HEAD(name) \
struct list_head name = LIST_HEAD_INIT(name)
#define INIT_LIST_HEAD(ptr) do { \
(ptr)->next = (ptr); (ptr)->prev = (ptr); \
} while (0)
/*
?* Insert a new entry between two known consecutive entries.?
?* This is only for internal list manipulation where we know the prev/next entries already!
?*/
static __inline__ void __list_add(struct list_head * new,?struct list_head * prev,?struct list_head * next)
{
next->prev = new;
new->next = next;
new->prev = prev;
prev->next = new;
}
/*
?* list_add - add a new entry
?* Insert a new entry after the specified head.
?* This is good for implementing stacks.
?*/
static __inline__ void list_add(struct list_head *new, struct list_head *head)
{
__list_add(new, head, head->next);
}
/*
?* list_add_tail - add a new entry
?* Insert a new entry before the specified head.
?* This is useful for implementing queues.
?*/
static __inline__ void list_add_tail(struct list_head *new, struct list_head *head)
{
__list_add(new, head->prev, head);
}
/*
?* Delete a list entry by making the prev/next entries point to each other.
?* This is only for internal list manipulation where we know the prev/next entries already!
?*/
static __inline__ void __list_del(struct list_head * prev, struct list_head * next)
{
next->prev = prev;
prev->next = next;
}
/*
?* list_del - deletes entry from list.
?* Note: list_empty on entry does not return true after this, the entry is in an undefined state.
?*/
static __inline__ void list_del(struct list_head *entry)
{
__list_del(entry->prev, entry->next);
}
/**
?* list_del_init - deletes entry from list and reinitialize it.
?*/
static __inline__ void list_del_init(struct list_head *entry)
{
__list_del(entry->prev, entry->next);
INIT_LIST_HEAD(entry);
}
/*
?* list_empty - tests whether a list is empty
?*/
static __inline__ int list_empty(struct list_head *head)
{
return head->next == head;
}
/**
?* list_splice - join two lists
?* @list: the new list to add.
?* @head: the place to add it in the first list.
?*/
static __inline__ void list_splice(struct list_head *list, struct list_head *head)
{
struct list_head *first = list->next;
?if (first != list) {
struct list_head *last = list->prev;
struct list_head *at = head->next;
first->prev = head;
head->next = first;
last->next = at;
at->prev = last;
}
}
/**
?* list_entry - get the struct for this entry
?* @ptr:?the &struct list_head pointer.
?* @type:?the type of the struct this is embedded in.
?* @member:?the name of the list_struct within the struct.
?*/
#define list_entry(ptr, type, member) \
((type *)((char *)(ptr)-(unsigned long)(&((type *)0)->member)))
/**
?* list_for_each?-?iterate over a list
?* @pos:?the &struct list_head to use as a loop counter.
?* @head:?the head for your list.
?*/
#define list_for_each(pos, head) \
for (pos = (head)->next; pos != (head); pos = pos->next)
#endif /* __KERNEL__ */
#endif
補充一下——給看不懂的同志。
它是個雙向的循環鏈表,這個鏈表可以用到任何結構里,比如:
struct kk {
type1 data1;
type2 data2;
......
struct list_head list;
typen datan;
}
再有一個鏈表頭:LIST_HEAD(kk_list)
然后調用鏈表函數的時候是這樣的,比如要把一個新結點kk_node加到鏈表尾
list_add_tail ( &(kk_node.list), &kk_list );
只是把結點中的list成員加進去,要獲得某個包含list_head節點的結構指針
struct kk *p = list_entry( p, kk, list );
當調用delete操作時,僅僅是把結點從鏈表中去掉,并不真正釋放結點所占用的空間,因為這是個通用結構嘛,分配釋放由用戶自己管理。
?
Trackback: http://tb.blog.csdn.net/TrackBack.aspx?PostId=3274
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
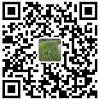
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
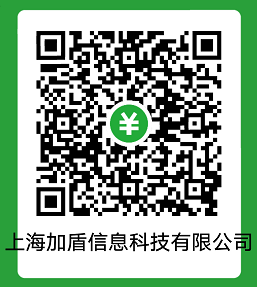