jQuery css模塊用于css屬性的修改操作。
?
jQuery.fn.css
jQuery.fn.css = function ( name, value ) { // 又是用access來操作 return jQuery.access( this , function ( elem, name, value ) { var styles, len, map = {}, i = 0 ; // 如果name是數(shù)組 if ( jQuery.isArray( name ) ) { // 通過getStyles方法返回elem的styles styles = getStyles( elem ); len = name.length; // 創(chuàng)建對(duì)應(yīng)styles的處理函數(shù)map for ( ; i < len; i++ ) { map[ name[ i ] ] = jQuery.css( elem, name[ i ], false , styles ); } return map; } // 如果不是數(shù)組,則返回jQuery.style或jQuery.css return value !== undefined ? jQuery.style( elem, name, value ) : jQuery.css( elem, name ); }, name, value, arguments.length > 1 ); };
又是通過jQuery.access來遍歷和操作屬性。
根據(jù)value值來判斷是返回一個(gè)函數(shù)數(shù)組,還是返回一個(gè)函數(shù)傳入jQuery.access。
主要用到j(luò)Query.css和jQuery.style兩個(gè)方法。
?
getStyles
function getStyles( elem ) { return window.getComputedStyle( elem, null ); }
這是一個(gè)獲取實(shí)際css style的方法。
可是……getComputedStyle是啥東西……
getComputedStyle
是一個(gè)可以獲取當(dāng)前元素所有最終使用的CSS屬性值。返回的是一個(gè)CSS樣式聲明對(duì)象([object CSSStyleDeclaration]),只讀。
語法:
var
styles = window.getComputedStyle("元素", "偽類");
如果沒有偽類,則傳null。
實(shí)際上就是獲取最終瀏覽器繪制時(shí)的css值,因?yàn)閟tyle不會(huì)返回所有css值,只會(huì)返回設(shè)置的css值,所以需要用該方法來獲得所有css值。
限于篇幅本文就不詳細(xì)解釋了,有興趣的朋友請(qǐng)參見: 獲取元素CSS值之getComputedStyle方法熟悉
?
jQuery.style
jQuery.style = function ( elem, name, value, extra ) { // 不處理text和comment節(jié)點(diǎn) if ( !elem || elem.nodeType === 3 || elem.nodeType === 8 || ! elem.style ) { return ; } var ret, type, hooks, // 修正css屬性名 origName = jQuery.camelCase( name ), style = elem.style; // jQuery.cssProps是css緩存,如果有則取出值,否則通過vendorPropName函數(shù)來得到實(shí)際的css名字 name = jQuery.cssProps[ origName ] || ( jQuery.cssProps[ origName ] = vendorPropName( style, origName ) ); // 獲取必要的鉤子 hooks = jQuery.cssHooks[ name ] || jQuery.cssHooks[ origName ]; // 如果value已定義 if ( value !== undefined ) { type = typeof value; // 如果value是+=或則-=一個(gè)數(shù),則轉(zhuǎn)成對(duì)應(yīng)的數(shù)字 if ( type === "string" && (ret = rrelNum.exec( value )) ) { value = ( ret[1] + 1 ) * ret[2] + parseFloat( jQuery.css( elem, name ) ); // 將其類型改成number type = "number" ; } // 確保NaN和null不被設(shè)置 if ( value == null || type === "number" && isNaN( value ) ) { return ; } // 如果value是數(shù)字則加上px if ( type === "number" && ! jQuery.cssNumber[ origName ] ) { value += "px" ; } // 修復(fù)#8908,IE9的問題,對(duì)于克隆的元素清除掉其background時(shí),其原型的background也會(huì)被清除 if ( !jQuery.support.clearCloneStyle && value === "" && name.indexOf("background") === 0 ) { style[ name ] = "inherit" ; } // 如果鉤子存在,則使用鉤子設(shè)置值,否則用style[ name ]來設(shè)置值 if ( !hooks || !("set" in hooks) || (value = hooks.set( elem, value, extra )) !== undefined ) { style[ name ] = value; } } else { // 如果鉤子存在,則使用鉤子返回值 if ( hooks && "get" in hooks && (ret = hooks.get( elem, false , extra )) !== undefined ) { return ret; } // 否則用style[ name ]來返回值 return style[ name ]; } };
這里面有一個(gè)挺有趣的問題。下面兩個(gè)代碼最后結(jié)果是多少呢?
alert(("-" + 5) + 6);alert(("-" + 5) * 6);
?
jQuery.css
jQuery.css = function ( elem, name, extra, styles ) { var val, num, hooks, origName = jQuery.camelCase( name ); // 修正名字name name = jQuery.cssProps[ origName ] || ( jQuery.cssProps[ origName ] = vendorPropName( elem.style, origName ) ); // 得到必要的鉤子 hooks = jQuery.cssHooks[ name ] || jQuery.cssHooks[ origName ]; // 如果有鉤子則使用get來獲取值 if ( hooks && "get" in hooks ) { val = hooks.get( elem, true , extra ); } // 沒有鉤子則用curCSS函數(shù)獲取 if ( val === undefined ) { val = curCSS( elem, name, styles ); } // 將"normal"轉(zhuǎn)成特定的值 if ( val === "normal" && name in cssNormalTransform ) { val = cssNormalTransform[ name ]; } // 是否需要強(qiáng)行轉(zhuǎn)成數(shù)字或者true if ( extra === "" || extra ) { num = parseFloat( val ); return extra === true || jQuery.isNumeric( num ) ? num || 0 : val; } return val; };
?
curCSS函數(shù)
var curCSS = function ( elem, name, _computed ) { var width, minWidth, maxWidth, computed = _computed || getStyles( elem ), // 解決IE9的問題,getPropertyValue用IE9中用.css('filter') ret = computed ? computed.getPropertyValue( name ) || computed[ name ] : undefined, style = elem.style; // 如果實(shí)際styles數(shù)組存在 if ( computed ) { // 如果ret為"",且elem不是子文檔(沒有style) if ( ret === "" && ! jQuery.contains( elem.ownerDocument, elem ) ) { ret = jQuery.style( elem, name ); } // 支持:Chrome < 17,Safari 5.1 // 來自Dean Edwards帥呆的hack // 將百分比轉(zhuǎn)成更加有用的px if ( rnumnonpx.test( ret ) && rmargin.test( name ) ) { // 記住原始值 width = style.width; minWidth = style.minWidth; maxWidth = style.maxWidth; // 通過改變minWidth、maxWidth、width得到相應(yīng)的px值 style.minWidth = style.maxWidth = style.width = ret; ret = computed.width; // 再將原來的值賦回去 style.width = width; style.minWidth = minWidth; style.maxWidth = maxWidth; } } return ret; };
?Dean Edwards的hack主要利用的是部分瀏覽器會(huì)使用計(jì)算值來表示元素寬度,而非使用值。
?
show & hide
jQuery.fn.show = function () { return showHide( this , true ); };
jQuery.fn.show直接引用showHide函數(shù),jQuery.fn.hide也是一樣:
jQuery.fn.hide = function () { return showHide( this ); };
而jQuery.fn. toggle則可接受state或者通過判斷當(dāng)前元素是否hidden,再調(diào)用jQuery.fn.show或者jQuery.fn.hide。
jQuery.fn.toggle = function ( state ) { var bool = typeof state === "boolean" ; return this .each( function () { if ( bool ? state : isHidden( this ) ) { jQuery( this ).show(); } else { jQuery( this ).hide(); } }); };
?
showHide函數(shù)
function showHide( elements, show ) { var elem, values = [], index = 0 , length = elements.length; // 遍歷所有元素 for ( ; index < length; index++ ) { elem = elements[ index ]; // 如果元素沒有style屬性,則跳過 if ( ! elem.style ) { continue ; } // 取出保存在緩存的olddisplay值 values[ index ] = jQuery._data( elem, "olddisplay" ); // 如果要顯示 if ( show ) { // 通過將display設(shè)置成"",來判斷""元素是否會(huì)顯示 if ( !values[ index ] && elem.style.display === "none" ) { elem.style.display = "" ; } // 如果display被設(shè)成了"",并且元素隱藏了,則通過css_defaultDisplay設(shè)置默認(rèn)顯示方法 if ( elem.style.display === "" && isHidden( elem ) ) { values[ index ] = jQuery._data( elem, "olddisplay" , css_defaultDisplay(elem.nodeName) ); } // 如果緩存沒有值,且元素沒有隱藏 } else if ( !values[ index ] && ! isHidden( elem ) ) { // 將目前的display值保存入緩存 jQuery._data( elem, "olddisplay", jQuery.css( elem, "display" ) ); } } // 在第二次循環(huán)設(shè)置display屬性,避免不斷回流 for ( index = 0; index < length; index++ ) { elem = elements[ index ]; if ( ! elem.style ) { continue ; } if ( !show || elem.style.display === "none" || elem.style.display === "" ) { // 是否要西那是,要顯示則設(shè)置成緩存值或者"",否則設(shè)置為"none" elem.style.display = show ? values[ index ] || "" : "none" ; } } return elements; }
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
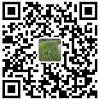
微信掃一掃加我為好友
QQ號(hào)聯(lián)系: 360901061
您的支持是博主寫作最大的動(dòng)力,如果您喜歡我的文章,感覺我的文章對(duì)您有幫助,請(qǐng)用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長非常感激您!手機(jī)微信長按不能支付解決辦法:請(qǐng)將微信支付二維碼保存到相冊,切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對(duì)您有幫助就好】元
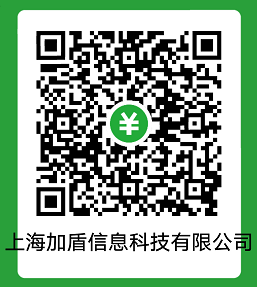