一、目的
1、畫一個立方體并自動旋轉。
二、程序運行結果
三、畫立方體
??畫一個立方體,需要八個頂點的數據。一個正方體如何畫出來,需要一個面一個面的畫,那么正方體有6個面,而每個面呢?是一個正方形,我們把正方形劃分為兩個三角形,這個三角形是opengl中最小的片元了。
??立方體有六個面,每個面兩個三角形,也就是12個三角形,每個三角形3個頂點,于是要定義36個頂點。
??使用語句glDrawArrays(GL_TRIANGLES, 0, 36)畫出36個點。
四、glVertexAttribPointer解析
??glVertexAttribPointer(0,3,GL_FLOAT,GL_FALSE,3
sizeof(float),(void
)0);
??第1個參數:0,因為這里只有一個屬性,所以從0開始。
??第2個參數:3,表示某個屬性包含的分量的個數。這里有3個頂點,但是每個頂點包含3個分量,也就是x、y、z。
??第3個參數:頂點屬性中每個分量的數據類型是什么,這里是float類型。
??第4個參數:表示是否將數據標準化。
??第5個參數:是每個屬性的長度,也就是步長,什么是步長,也就是第一個屬性從多少道多少,第2個屬性從多少到多少,每當我們要新的屬性的時候,一步要走多少,這里每個屬性包含3個float長度的數據,所以步長為3。
??第6個參數:表示屬性的偏移,因為我們這里的屬性只有一個也就是頂點位置屬性,沒有其他的屬性,所以這里的偏移為0。
??接著使用glEnableVertexAttribArray(0)將這個屬性的狀態變為使能狀態,也就是可用的狀態。
??這個是C++的語句解析,Python沒辦法表示偏移,所以是None
五、沿任意矢量軸的旋轉
??為了看看立方體的效果,對立方體沿矢量(0.7071,0.7071,0)軸進行旋轉,旋轉矩陣計算方法見參考文獻2,旋轉矩陣為:
六、源代碼
"""
glfw_cube01.py
Author: dalong10
Description: Draw a Cube, learning OPENGL
"""
import glutils #Common OpenGL utilities,see glutils.py
import sys, random, math
import OpenGL
from OpenGL.GL import *
from OpenGL.GL.shaders import *
import numpy
import numpy as np
import glfw
strVS = """
#version 330 core
layout(location = 0) in vec3 position;
uniform float theta;
void main(){
mat4 rot=mat4( vec4(0.5+0.5*cos(theta), 0.5-0.5*cos(theta), -0.707106781*sin(theta), 0),
vec4(0.5-0.5*cos(theta),0.5+0.5*cos(theta), 0.707106781*sin(theta),0),
vec4(0.707106781*sin(theta), -0.707106781*sin(theta),cos(theta), 0.0),
vec4(0.0, 0.0,0.0, 1.0));
gl_Position=rot *vec4(position.x, position.y, position.z, 1.0);
}
"""
strFS = """
#version 330 core
out vec3 color;
void main(){
color = vec3(1,1,0);
}
"""
class FirstCube:
def __init__(self, side):
self.side = side
# load shaders
self.program = glutils.loadShaders(strVS, strFS)
glUseProgram(self.program)
s = side/2.0
cube_vertices = [
-s, s, -s,
-s, -s, -s,
s, s, -s,
s, -s, -s,
s, s, -s,
-s, -s, -s,
-s, s, s,
-s, -s, s,
s, s, s,
s, -s, s,
s, s, s,
-s, -s, s,
-s, -s, s,
-s, -s, -s,
s, -s, s,
s, -s, -s,
s, -s, s,
-s, -s, -s,
-s, s, s,
-s, s, -s,
s, s, s,
s, s, -s,
s, s, s,
-s, s, -s,
-s, -s, s,
-s, -s, -s,
-s, s, s,
-s, s, -s,
-s, s, s,
-s, -s, -s,
s, -s, s,
s, -s,-s,
s, s, s,
s, s, -s,
s, s, s,
s, -s,-s
]
# set up VBOs
vertexData = numpy.array(cube_vertices, numpy.float32)
self.vertexBuffer = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, self.vertexBuffer)
glBufferData(GL_ARRAY_BUFFER, 4*len(vertexData), vertexData, GL_STATIC_DRAW)
# set up vertex array object (VAO)
self.vao = glGenVertexArrays(1)
glBindVertexArray(self.vao)
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 0, None)
glEnableVertexAttribArray(0)
# unbind VAO
glBindVertexArray(0)
glBindBuffer(GL_ARRAY_BUFFER, 0)
def render(self):
# use shader
glUseProgram(self.program)
theta = i*PI/180.0
glUniform1f(glGetUniformLocation(self.program, "theta"), theta)
# bind VAO
glBindVertexArray(self.vao)
# draw
glDrawArrays(GL_TRIANGLES, 0, 36)
# unbind VAO
glBindVertexArray(0)
if __name__ == '__main__':
import sys
import glfw
import OpenGL.GL as gl
def on_key(window, key, scancode, action, mods):
if key == glfw.KEY_ESCAPE and action == glfw.PRESS:
glfw.set_window_should_close(window,1)
# Initialize the library
if not glfw.init():
sys.exit()
# Create a windowed mode window and its OpenGL context
window = glfw.create_window(300, 300, "draw Cube ", None, None)
if not window:
glfw.terminate()
sys.exit()
# Make the window's context current
glfw.make_context_current(window)
# Install a key handler
glfw.set_key_callback(window, on_key)
PI = 3.14159265358979323846264
# Loop until the user closes the window
a=0
while not glfw.window_should_close(window):
# Render here
width, height = glfw.get_framebuffer_size(window)
ratio = width / float(height)
gl.glViewport(0, 0, width, height)
gl.glClear(gl.GL_COLOR_BUFFER_BIT)
gl.glClearColor(0.0,0.0,4.0,0.0)
firstCube0 = FirstCube(1.0)
i=a
firstCube0.render()
a=a+1
if a>360:
a=0
# Swap front and back buffers
glfw.swap_buffers(window)
# Poll for and process events
glfw.poll_events()
glfw.terminate()
七、參考文獻
1、wodownload2博客https://blog.csdn.net/wodownload2/article/details/78139273
2、csxiaoshui博客https://blog.csdn.net/csxiaoshui/article/details/65446125
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
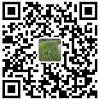
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
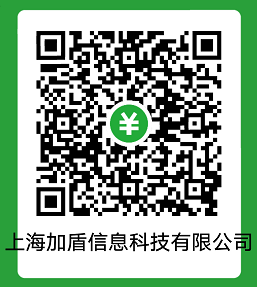