# -*- coding: utf-8 -*-
import requests
import os
from biplist import *
from mod_pbxproj import XcodeProject
?
def CleanDirectory(dirPath):
? ? isexits = os.path.exists(dirPath)
? ? if isexits:
? ? ? ? for root, dirs, files in os.walk(dirPath):
? ? ? ? ? ? for fileName in files:
? ? ? ? ? ? ? ? del_file = os.path.join(root, fileName)
? ? ? ? ? ? ? ? os.remove(del_file)
? ? else :
? ? ? ? os.mkdir(dirPath)
?
class Server():
? ? dev ? ? = 0
? ? test? ? = 1
? ? online? = 2
? ? staging = 3
?
def CodeUpdate():
? ? gotoRootDir = os.system("cd %s"%(os.getcwd()))
? ? gitFetch = os.system("git fetch --all")
? ? gitResetLocal = os.system("git reset --hard origin/master")
? ? gitPull = os.system("git pull")
?
def ModifyInfoPlist(app_name,app_version,build_version="1.0"):
? ? infoPlist = readPlist('./Pocket7Games/Info.plist')
? ? infoPlist["CFBundleVersion"] = build_version
? ? infoPlist["CFBundleShortVersionString"] = app_version
? ? infoPlist["CFBundleDisplayName"] = app_name
? ? writePlist(infoPlist,"./Pocket7Games/Info.plist")
?
def ModifyExportOptionsPlist(team_id,method,profile_id,profile_name):
? ? exportPlist = readPlist('./ExportOptions.plist')
? ? exportPlist["teamID"] = team_id
? ? exportPlist["method"] = method
? ? exportPlist["provisioningProfiles"] = {profile_id:profile_name}
? ? writePlist(exportPlist,"./ExportOptions.plist")
?
def ModifyXcodeProject(bundle_id,profile_name,codesign_id,team_id):
? ? project = XcodeProject.Load('./Pocket7Games.xcodeproj/project.pbxproj')
? ? rootObject = project["rootObject"]
? ? projectObject = project["objects"][rootObject]["targets"]
? ? buildConfigurationLists = []
? ? for id in projectObject:
? ? ? ? buildConfigurationList = project["objects"][id]["buildConfigurationList"]
? ? ? ? buildConfigurationLists.append(buildConfigurationList)
? ? buildListsArr = []
? ? for key in buildConfigurationLists:
? ? ? ? buildListsArr = buildListsArr + project["objects"][key]["buildConfigurations"]
? ? for key in buildListsArr:
? ? ? ? version =? project["objects"][key]["name"].lower()
? ? ? ? if project["objects"][key]["buildSettings"]:
? ? ? ? ? ? buildSettingsArr = project["objects"][key]["buildSettings"]
? ? ? ? ? ? buildSettingsArr['PRODUCT_BUNDLE_IDENTIFIER'] = bundle_id
? ? ? ? ? ? buildSettingsArr['PROVISIONING_PROFILE_SPECIFIER'] = profile_name
? ? ? ? ? ? buildSettingsArr['CODE_SIGN_IDENTITY'] = codesign_id
? ? ? ? ? ? buildSettingsArr['CODE_SIGN_STYLE'] = "Manual"
? ? ? ? ? ? buildSettingsArr['DEVELOPMENT_TEAM'] = team_id
? ? project.save()
?
def ModifyLinkedServer(server):
? ? networkDefinesPath = './Pocket7Games/NetWork/NCNetWorkDefines.h'
? ? networkDefinesPath_New = './Pocket7Games/NetWork/NCNetWorkDefines_New.h'
? ? networkDefinesPath_Bak = './Pocket7Games/NetWork/NCNetWorkDefines_Bak.h'
? ? with open(networkDefinesPath, 'r') as read_file, open(networkDefinesPath_New, 'w') as write_file:
? ? ? ? for r_line in read_file:
? ? ? ? ? ? if "#define HostFlag" in r_line :
? ? ? ? ? ? ? ? serverString = "#define HostFlag %d\n"%(server)
? ? ? ? ? ? ? ? write_file.write(serverString)
? ? ? ? ? ? else :
? ? ? ? ? ? ? ? write_file.write(r_line)
? ? os.rename(networkDefinesPath, networkDefinesPath_Bak)
? ? os.rename(networkDefinesPath_New, networkDefinesPath)
? ? os.remove(networkDefinesPath_Bak)
?
def ModifyDebugBall(show_debugball):
? ? debugBallPath = './Pocket7Games/AviaAppDelegate.mm'
? ? debugBallPath_New = './Pocket7Games/AviaAppDelegate_New.mm'
? ? debugBallPath_Bak = './Pocket7Games/AviaAppDelegate_Bak.mm'
? ? with open(debugBallPath, 'r') as read_file, open(debugBallPath_New, 'w') as write_file:
? ? ? ? for r_line in read_file:
? ? ? ? ? ? if "[[AviaDebug sharedAviaDebug] addDebugButton]" in r_line :
? ? ? ? ? ? ? ? if show_debugball:
? ? ? ? ? ? ? ? ? ? write_file.write("? ? [[AviaDebug sharedAviaDebug] addDebugButton];\n")
? ? ? ? ? ? ? ? else :
? ? ? ? ? ? ? ? ? ? write_file.write("http://? ? [[AviaDebug sharedAviaDebug] addDebugButton];\n")
? ? ? ? ? ? else :
? ? ? ? ? ? ? ? write_file.write(r_line)
? ? os.rename(debugBallPath, debugBallPath_Bak)
? ? os.rename(debugBallPath_New, debugBallPath)
? ? os.remove(debugBallPath_Bak)
?
def OutputIPA():
? ? BuildCommandPath = "/Applications/Xcode.app/Contents/Developer/usr/bin/xcodebuild"
? ? archiveDir = "{0}/NC_iOSArchive".format(os.path.join(os.path.expanduser("~"), 'Desktop'))
? ? archivePath = "{0}/NC_iOSArchive/Pocket7Games".format(os.path.join(os.path.expanduser("~"), 'Desktop'))
? ? exportOptionsPath = os.path.abspath('ExportOptions.plist')
? ? CleanDirectory(archiveDir)
? ? cleanResult = os.system("{0} -workspace Pocket7Games.xcworkspace -scheme Pocket7Games clean".format(BuildCommandPath))
? ? buildResult = os.system("{0} -workspace Pocket7Games.xcworkspace -scheme Pocket7Games build".format(BuildCommandPath))
? ? archiveResult = os.system("{0} archive -workspace Pocket7Games.xcworkspace -scheme Pocket7Games -archivePath {1}".format(BuildCommandPath,archivePath))
? ? outputIPAResult = os.system("{0} -exportArchive -archivePath {1}.xcarchive -exportPath {2} -exportOptionsPlist {3}".format(BuildCommandPath,archivePath,archiveDir,exportOptionsPath))
? ? ipaPath = "%s.ipa"%(archivePath)
? ? print ("ipa文件路徑:{0}".format(ipaPath))
? ? return ipaPath
?
def UploadToFir(ipaPath):
? ? firApiToken = "XXXXX"
? ? publishResult = os.system("fir publish {0} -T {1}".format(ipaPath,firApiToken))
?
def NewCodeBuild(app_name,version,bundle_id,profile_name,team_id,enviroment,debugFlag,server):
? ? CodeUpdate()
? ? ModifyInfoPlist(app_name,version)
? ? ModifyExportOptionsPlist(team_id,enviroment,bundle_id,profile_name)
? ? ModifyXcodeProject(bundle_id,profile_name,"iPhone Developer",team_id)
? ? ModifyLinkedServer(server)
? ? ModifyDebugBall(debugFlag)
? ? ipaPath = OutputIPA()
? ? UploadToFir(ipaPath)
?
#-----------------------這是分割線,下面是入口函數-----------------------
if __name__ == '__main__':
?? ?
#? ? App的名字
? ? app_name = "Pocket7Games"
#? ? App的版本
? ? version = "1.3.1"
#? ? App的標識符
? ? bundle_id = ""
#? ? 開發者賬號的配置文件名
? ? profile_name = "Pocket7Test"
#? ? 開發者賬號的ID,常用第一個(1) ? (2)
? ? team_id = "XXXX"
#? ? 打包設置,常用development? (1)app-store ? (2)ad-hoc ? (3)enterprise ? (4)development
? ? enviroment = "development"
#? ? 調試模式的小籃球開關 True False
? ? debugFlag = True
#? ? 服務端的服務器設置,參照腳本的18~21行去修改
? ? server = Server().test
?? ?
# ? **下面是執行體,不要動!!!**
? ? NewCodeBuild(app_name,version,bundle_id,profile_name,team_id,enviroment,debugFlag,server)
?? ?
注:
fir安裝命令
sudo gem install fir-cli
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
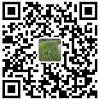
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
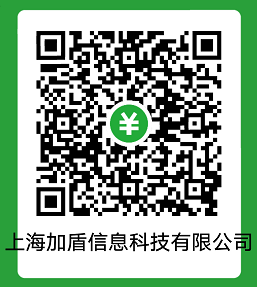