使用正則表達式在 System.TextRegularExpression
?
?
using?System;
using?System.Text.RegularExpressions;
class?chapter8
{
????static?void?Main()
????{
????????Regex?reg?=?new?Regex("the");
????????string?str1?=?"the?quick?brown?fox?jumped?over?the?lazy?dog";
????????Match?matchSet;
????????int?matchPos;
????????matchSet?=?reg.Match(str1);
????????if?(matchSet.Success)
????????{?
????????????matchPos?=?matchSet.Index;
????????????Console.WriteLine("found?match?at?position:"?+?matchPos);
????????}
????}
}
?
if?(Regex.IsMatch(str1,?"the"))
{
????Match?aMatch;
aMatch?=?reg.Match(str1);
?
using?System;
using?System.Text.RegularExpressions;
class?chapter8
{
????static?void?Main()
????{
????????Regex?reg?=?new?Regex("the");
????????string?str1?=?"the?quick?brown?fox?jumped?over?the?lazy?dog";
????????MatchCollection?matchSet;
????????matchSet?=?reg.Matches(str1);
????????if?(matchSet.Count?>?0)
????????????foreach?(Match?aMatch?in?matchSet)
????????????????Console.WriteLine("found?a?match?at:?"?+?aMatch.Index);
????????Console.Read();
????}
}
?
數量詞
(+) 這個數量詞說明正則表達式應該匹配一個或者多次緊接其前的字符。
(*) 這個數量詞說明正則表達式應該匹配零個或者多次緊接其前的字符。 // 實踐中非常難用,會導致匹配太多
(?) 這個數量詞說明正則表達式應該匹配零次或者多次緊接其前的字符。
{N}? 這個數量詞指定要匹配的數量。
{m,n} 這個數量詞指定最小,做大匹配數量。也可以 {m,},{,n} 只指定最大和最小。
?
?
using?System;
using?System.Text.RegularExpressions;
class?chapter8
{
????static?void?Main()
????{
????????string[]?words?=?new?string[]{"Part",?"of",?"this","<b>string</b>",?"is",?"bold"};
????????string?regExp?=?"<.*>";?//? 應該修改成? <.+?>??+ 僅使用這個是不行的。 .?(.) 句點表示與任意字符匹配
????????MatchCollection?aMatch;
????????foreach?(string?word?in?words)
????????{
????????????if?(Regex.IsMatch(word,?regExp))
????????????{
????????????????aMatch?=?Regex.Matches(word,?regExp);
????????????????for?(int?i?=?0;?i?<?aMatch.Count;?i++)
????????????????????Console.WriteLine(aMatch[i].Value);
????????????}
????????}
????}
}
原本期望這個程序就返回兩個標簽: <b> 和 </b>?? 但由于貪心,正則返回了 <b>string</b>? 。利用惰性量詞 (?)? 可以解決? <.+?>? 僅適用 +? 是不行的,必須加惰性量詞 ?
使用字符類
句點 (.) 的通常是用它在字符內部定義字符范圍,也就是用來限定字符串的開始 / 結束字符。
句點匹配任意字符。
using?System;
using?System.Text.RegularExpressions;
class?chapter8
{
????static?void?Main()
????{
????????string?str1?=?"the?quick?brown?fox?jumped?over?the?lazy?dog?one?time";
????????MatchCollection?matchSet;
????????matchSet?=?Regex.Matches(str1,?"t.e");
????????foreach?(Match?aMatch?in?matchSet)
????????????Console.WriteLine("Matches?at:?"?+?aMatch.Index);
????}
}
?
?
檢查字符組的模式?, ([]) 。在方括號內的字符稱為“字符類”
using?System;
using?System.Text.RegularExpressions;
class?chapter8
{
????static?void?Main()
????{
????????string?str1?=?"THE?quick?BROWN?fox?JUMPED?over?THE?lazy?DOG";
????????MatchCollection?matchSet;
????????matchSet?=?Regex.Matches(str1,?"[a-z]");
????????foreach?(Match?aMatch?in?matchSet)
????????????Console.WriteLine("Matches?at:?"?+?aMatch.Index);
????}
}
[]A-Za-z]? 所有英文字母大小寫
字符類前面放 (^)? 表示字符類的反或者否定如 [aeiou]? 表示元音,那么 [^aeiou] 表示非元音。
[]A-Za-z0-9?] 表示單詞,也可以用 \w 表示 , 用 \W 表示 \w 的反向即非單詞。
[0-9] 可以用 \d? 表示
[^0-9]? 表示 \D?
\s? 表示空格? \S 表示非空格。
?
斷言
(^)? 在開始處匹配
($)? 在結束處匹配
\b? 在開始結束匹配
using?System;
using?System.Text.RegularExpressions;
class?chapter8
{
????static?void?Main()
????{
????????string[]?words?=?new?string[]?{?"heal",?"heel",?"noah",?"techno"?};
????????string?regExp?=?"^h";
????????Match?aMatch;
????????foreach?(string?word?in?words)
????????????if?(Regex.IsMatch(word,?regExp))
????????????{
????????????????aMatch?=?Regex.Match(word,?regExp);
????????????????Console.WriteLine("Matched:?"?+?word?+?"?at?position:?"?+?aMatch.Index);
????????????}
????}
}
?
string?regExp?=?"h$";
string?words?=?"hark,?what?doth?thou?say,?Harold??";
string?regExp?=?"\\bh";
?
?使用分組構造?
?1? 匿名分組
通過括號內圍繞的正則表達式就可以組成組
using?System;
using?System.Text.RegularExpressions;
class?chapter8
{
????static?void?Main()
????{
????????string?words?=?"08/14/57?46?02/25/59?45?06/05/85?18"?+?"03/12/88?16?09/09/90?13";
????????string?regExp1?=?"(\\s\\d{2}\\s)";
????????MatchCollection?matchSet?=?Regex.Matches(words,regExp1);
????????foreach?(Match?aMatch?in?matchSet)
????????????Console.WriteLine(aMatch.Groups[0].Captures[0]);
????}
}
?2? 命名組
命名組通過在正則表達式前綴的問號和一對尖括號擴著的名字組成。
例如? "ages?" 中的組名
正則如下 (?<ages>\\s\\d{2}\\s)
using?System;
using?System.Text.RegularExpressions;
class?chapter8
{
????static?void?Main()
????{
????????string?words?=?"08/14/57?46?02/25/59?45?06/05/85?18?"?+?"03/12/88?16?09/09/90?13";
????????string?regExp1?=?"(?<dates>(\\d{2}/\\d{2}/\\d{2}))\\s";
????????MatchCollection?matchSet?=?Regex.Matches(words,regExp1);
????????foreach?(Match?aMatch?in?matchSet)
????????????Console.WriteLine("Date:?{0}",?aMatch.Groups["dates"]);
????}
}
?
?
零寬度正向預搜索斷言和零寬度反向預搜索斷言
斷言還可以用來確定正則表達式向前或者向后匹配程度,這些斷言可能是正(?匹配模式),也能是負的 ( 非匹配模式 ) 。
(?=reg-exp-char)
string?words?=?"lions?lion?tigers?tiger?bears,bear";
string?regExp1?=?"\\w+(?=\\s)";?\\?? 只匹配當前子表達式在指定位置右側,那么匹配就繼續。
?
負的正向預搜索斷言?,只要搜索到不匹配的當前表達式的指定位置右側,那么斷言就繼續。
string?words?=?"subroutine?routine?subprocedure?procedure";
string?regExp1?=?"\\b(?!sub)\\w+\\b";
?
反向預搜索斷言
只要字表達式不匹配在位置左側,那么負的反向與搜索斷言就繼續。
string?words?=?"subroutines?routine?subprocedures
procedure";
string?regExp1?=?"\\b\\w+(?<=s)\\b";
?
?
string?regExp1?=?"\\b\\w+(?<!s)\\b";
?
?
CaptureCollection? 類
using?System;
using?System.Text.RegularExpressions;
class?chapter8
{
????static?void?Main()
????{
????????string?dates?=?"08/14/57?46?02/25/59?45?06/05/85?18?"?+?"03/12/88?16?09/09/90?13";
????????string?regExp?=?"(?<dates>(\\d{2}/\\d{2}/\\d{2}))\\s(?<ages>(\\d{2}))\\s";
????????MatchCollection?matchSet;
????????matchSet?=?Regex.Matches(dates,?regExp);
????????Console.WriteLine();
????????foreach?(Match?aMatch?in?matchSet)
????????{
????????????foreach?(Capture?aCapture?in?aMatch.Groups["dates"].Captures)
????????????????Console.WriteLine("date?capture:?"?+?aCapture.ToString());
????????????foreach?(Capture?aCapture?in?aMatch.Groups["ages"].Captures)
????????????????Console.WriteLine("age?capture:?"?+?aCapture.ToString());
????????}
????}
}
?
正則表達式選項
matchSet?=?Regex.Matches(dates,?regexp,?RegexOptions.Multiline);
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
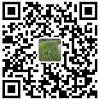
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
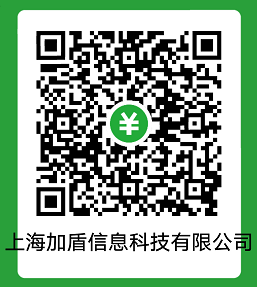