第十二章 Django框架
12.1 服務(wù)器程序和應(yīng)用程序
服務(wù)器程序負(fù)責(zé)對socket服務(wù)器進(jìn)行封裝,并在請求到來時(shí),對請求的各種數(shù)據(jù)進(jìn)行整理。應(yīng)用程序則負(fù)責(zé)具體的邏輯處理。為了方便應(yīng)用程序的開發(fā),就出現(xiàn)了眾多的Web框架,例如:Django、Flask、web.py 等。不同的框架有不同的開發(fā)方式,但是無論如何,開發(fā)出的應(yīng)用程序都要和服務(wù)器程序配合,才能為用戶提供服務(wù)。
WSGI(Web Server Gateway Interface)就是一種規(guī)范,它定義了使用Python編寫的web應(yīng)用程序與web服務(wù)器程序之間的接口格式,實(shí)現(xiàn)web應(yīng)用程序與web服務(wù)器程序間的解耦。
常用的WSGI服務(wù)器有uwsgi、Gunicorn,而Python標(biāo)準(zhǔn)庫提供的獨(dú)立WSGI服務(wù)器叫 wsgiref ,Django開發(fā)環(huán)境用的就是這個(gè)模塊來做服務(wù)器。
12.11 用wsgiref代替socket server
import time from wsgiref.simple_server import make_server def home(url): # 將返回不同的內(nèi)容部分封裝成函數(shù) s = " this is {} page! " .format(url) return bytes(s, encoding= " utf8 " ) def index(url): return b 'index page
' def user(url): # 不同的用戶得到的頁面上顯示不同的時(shí)間 c_time = str(time.time()) with open( " user.html " , " r " ) as f: data_s = f.read() data_s = data_s.replace( " @@xx@@ " , c_time) return bytes(data_s, encoding= " utf8 " ) ? url2func = [ # url和實(shí)際要執(zhí)行的函數(shù)的對應(yīng)關(guān)系 ( " /index/ " , index), ( " /home/ " , home), ( " /user/ " , user),] ? def run_server(environ, start_response): # 按照wsgiref的要求定義一個(gè)run_server函數(shù) start_response( ' 200 OK ' ,[( ' Content-Type ' , ' text/html;charset=utf8 ' ),]) # 設(shè)置HTTP響應(yīng)的狀態(tài)碼和頭信息 url = environ[ ' PATH_INFO ' ] # 取到用戶輸入的url func = None for i in url2func: if url == i[0]: func = i[1] # 拿到將要執(zhí)行的函數(shù) break if func: msg = func(url) # 執(zhí)行對應(yīng)的函數(shù) else : msg = b '404
' # 找不到要執(zhí)行的函數(shù)就返回404 return [msg, ] # 發(fā)送消息到客戶端 ? if __name__ == ' __main__ ' : httpd = make_server( ' 127.0.0.1 ' , 8090 , run_server) print ( " 我在8090等你哦... " ) httpd.serve_forever()
12.12 HTML模板渲染工具jinja2
user.html:
< body > < table border ="1" > < thead > < tr > < th > id th > < th > 姓名 th > < th > 愛好 th > tr > thead > < tbody > {% for user in user_list %} < tr > < td > {{user.id}} td > < td > {{user.name}} td > < td > {{user.hobby}} td > tr > {% endfor %} tbody > table > body >
使用jinjia2替換HTML中的數(shù)據(jù):
使用pymysql模塊查詢數(shù)據(jù)庫獲取數(shù)據(jù),使用jinjia2替換HTML中的數(shù)據(jù)
模板的原理就是字符串替換,我們只要在HTML頁面中遵循jinja2的語法規(guī)則,其內(nèi)部就會按照指定的語法進(jìn)行相應(yīng)的替換,從而達(dá)到 動(dòng)態(tài) 的返回內(nèi)容
import time from wsgiref.simple_server import make_server from jinja2 import Template import pymysql def user(url): conn = pymysql.connect( # 從數(shù)據(jù)庫里面去到所有的用戶信息, host= ' 127.0.0.1 ' , port =3306 , user = ' root ' , password = ' 123 ' , database = ' db6 ' , charset = ' utf8 ' ) cursor = conn.cursor(cursor= pymysql.cursors.DictCursor) cursor.execute( ' select * from user ' ) ret = cursor.fetchall() # print(ret) with open( " user.html " , " r " , encoding= " utf8 " ) as f: data_s = f.read() template = Template(data_s) # 生成一個(gè)模板文件實(shí)例 msg = template.render({ " user_list " : ret}) # 把數(shù)據(jù)填充到模板里面 return bytes(msg, encoding= " utf8 " ) url2func = [ # url和將要執(zhí)行的函數(shù)的對應(yīng)關(guān)系 ( " /index/ " , index), ( " /home/ " , home), ( " /user/ " , user),] def run_server(environ, start_response): # 按照wsgiref的要求定義一個(gè)run_server函數(shù) start_response( ' 200 OK ' ,[( ' Content-Type ' , ' text/html;charset=utf8 ' ),]) # 設(shè)置HTTP響應(yīng)的狀態(tài)碼和頭信息 url = environ[ ' PATH_INFO ' ] # 取到用戶輸入的url ? func = None for i in url2func: if url == i[0]: func = i[1] # 拿到將要執(zhí)行的函數(shù) break if func: msg = func(url) # 執(zhí)行對應(yīng)的函數(shù) else : msg = b '404
' # 找不到要執(zhí)行的函數(shù)就返回404 return [msg, ] # 發(fā)送消息到客戶端 ? if __name__ == ' __main__ ' : httpd = make_server( ' 127.0.0.1 ' , 8090 , run_server) print ( " 我在8090等你哦... " ) httpd.serve_forever()
12.2 Django基礎(chǔ)必備三件套
用命令創(chuàng)建了一個(gè)名為"mysite"的Django 項(xiàng)目
django-admin startproject mysite
Django目錄:
mysite/ ├── manage.py # 管理文件 └── mysite # 項(xiàng)目目錄 ├── __init__ .py ├── settings.py # 配置 ├── urls.py # 路由 --> URL和函數(shù)的對應(yīng)關(guān)系 └── wsgi.py # runserver命令就使用wsgiref模塊做簡單的 web server
用命令運(yùn)行Django項(xiàng)目
python3 manage.py runserver 127.0.0.1:8000 # 默認(rèn)使用8000端口
模板文件配置: settings
TEMPLATES = [ { ' BACKEND ' : ' django.template.backends.django.DjangoTemplates ' , ' DIRS ' : [os.path.join(BASE_DIR, " template " )], # template文件夾位置 ' APP_DIRS ' : True, ' OPTIONS ' : { ' context_processors ' : [ ' django.template.context_processors.debug ' , ' django.template.context_processors.request ' , ' django.contrib.auth.context_processors.auth ' , ' django.contrib.messages.context_processors.messages ' , ], }, }, ]
靜態(tài)文件配置: settings
STATIC_URL = ' /static/ ' # HTML中使用的靜態(tài)文件夾前綴 STATICFILES_DIRS = [ os.path.join(BASE_DIR, " static " ), # 靜態(tài)文件存放位置 ]
12.21 HttpResponse
內(nèi)部傳入一個(gè)字符串參數(shù),返回給瀏覽器
from django.conf.urls import url from django.contrib import admin from django.shortcuts import HttpResponse, render, redirect def index(request): # 所有跟請求相關(guān)的數(shù)據(jù)都封裝到了request這個(gè)參數(shù)里面 # 業(yè)務(wù)邏輯代碼 return HttpResponse( " 這是index頁面! " )
12.22 render
除request參數(shù)外還接受一個(gè)待渲染的模板文件和一個(gè)保存具體數(shù)據(jù)的字典參數(shù),將數(shù)據(jù)填充進(jìn)模板文件,最后把結(jié)果返回給瀏覽器(類似于上面用到的jinja2)
def login(request): # 自己去找HTML文件 # with open("templates/login.html", "r", encoding="utf8") as f: # data = f.read() # return HttpResponse(data) # Django去找login.html文件,讀取出來內(nèi)容,返回給瀏覽器 return render(request, " login.html " , { " name " : " alex " , " hobby " : [ " 燙頭 " , " 泡吧 " ]})
12.23 redirect
接受一個(gè)URL參數(shù),表示跳轉(zhuǎn)到指定的URL
def index(request): # 業(yè)務(wù)邏輯代碼 return redirect( " /home/ " )
12.24 設(shè)置URL和函數(shù)的對應(yīng)關(guān)系
urlpatterns = [ url(r ' ^admin/ ' , admin.site.urls), url(r ' ^home/ ' , home), url(r ' ^index/ ' , index), url(r ' ^login/ ' , login), ]
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
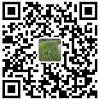
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動(dòng)力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長非常感激您!手機(jī)微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
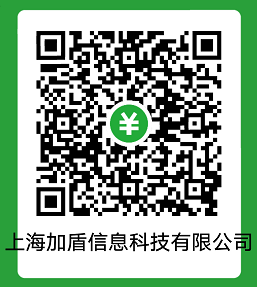