?這個教程,我們將展示如何用python創建一個井字游戲。 其中我們將使用函數、數組、if條件語句、while循環語句和錯誤捕獲等。
首先我們需要創建兩個函數,第一個函數用來顯示游戲板:
?
def print_board(): for i in range(0,3): for j in range(0,3): print map[2-i][j], if j != 2: print "|", print ""
這我們使用兩個for循環來遍歷map,該map是一個包含了位置信息的二維數組。
游戲板看起來是這樣的:
?
| | | | | | X | X | O | X | O | O | X X | X | X X | X | X X | X | X
?
下面我們需要一個函數check_done()來檢查游戲是否結束。如果結束,則返回True并打印消息。
?
def check_done(): for i in range(0,3): if map[i][0] == map[i][1] == map[i][2] != " " \ or map[0][i] == map[1][i] == map[2][i] != " ": print turn, "won!!!" return True if map[0][0] == map[1][1] == map[2][2] != " " \ or map[0][2] == map[1][1] == map[2][0] != " ": print turn, "won!!!" return True if " " not in map[0] and " " not in map[1] and " " not in map[2]: print "Draw" return True return False
有幾個地方需要檢查,首先檢查水平和垂直方向,是否有一行或一列不為空且包含有三個相同的符號,然后我們再檢查斜方向。如果上面有一個方向滿足,游戲結束并打印“Won!!!”。請注意檢查變量改變,它用來標記當前是哪一位玩家。
同時我們需要檢查當前游戲板是否被填滿且沒有人獲勝,游戲平局。
有了上面的兩個函數,下面我們創建3個變量:
?
turn = "X" map = [[" "," "," "], [" "," "," "], [" "," "," "]] done = False
??? turn : 輪到誰
??? map : 游戲板
??? done : 游戲是否結束
現在啟動游戲:
?
while done != True: print_board() print turn, "'s turn" print moved = False while moved != True:
這里使用了while循環直到游戲結束并返回true.在這個循環里面,使用了另外一個while循環來檢查玩家是否移動,如果玩家沒有移動,則程序會跳到下一次循環。
下一步告訴玩家怎么玩:
?
print "Please select position by typing in a number between 1 and 9, see below for which number that is which position..." print "7|8|9" print "4|5|6" print "1|2|3" print try: pos = input("Select: ") if pos <=9 and pos >=1:
我們期望玩家輸入一個數字,檢查該數字是否是在1到9之間。另外,我們這里需要一段錯誤處理邏輯,我們還需要需要檢查玩家是否能移動到一個位置:
?
Y = pos/3 X = pos%3 if X != 0: X -=1 else: X = 2 Y -=1
以下是全部的代碼:
?
def print_board(): for i in range(0,3): for j in range(0,3): print map[2-i][j], if j != 2: print "|", print "" def check_done(): for i in range(0,3): if map[i][0] == map[i][1] == map[i][2] != " " \ or map[0][i] == map[1][i] == map[2][i] != " ": print turn, "won!!!" return True if map[0][0] == map[1][1] == map[2][2] != " " \ or map[0][2] == map[1][1] == map[2][0] != " ": print turn, "won!!!" return True if " " not in map[0] and " " not in map[1] and " " not in map[2]: print "Draw" return True return False turn = "X" map = [[" "," "," "], [" "," "," "], [" "," "," "]] done = False while done != True: print_board() print turn, "'s turn" print moved = False while moved != True: print "Please select position by typing in a number between 1 and 9,\ see below for which number that is which position..." print "7|8|9" print "4|5|6" print "1|2|3" print try: pos = input("Select: ") if pos <=9 and pos >=1: Y = pos/3 X = pos%3 if X != 0: X -=1 else: X = 2 Y -=1 if map[Y][X] == " ": map[Y][X] = turn moved = True done = check_done() if done == False: if turn == "X": turn = "O" else: turn = "X" except: print "You need to add a numeric value"
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
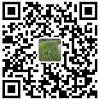
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
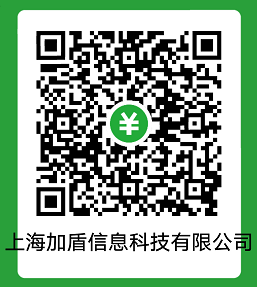