- 圖像的幾何變換
imgobj = cv2.imread('pho.png') #讀取圖像
cv2.namedWindow("image") #創(chuàng)建窗口并顯示的是圖像類型
cv2.imshow("image",imgobj)
cv2.waitKey(0)??????? #等待事件觸發(fā),參數(shù)0表示永久等待
cv2.destroyAllWindows()?? #釋放窗口
# resize
res = cv2.resize(imgobj,None,fx=0.5, fy=0.5, interpolation = cv2.INTER_CUBIC)
cv2.imshow("image",res)
#warpAffine?? 仿射
rows,cols = res.shape[0:2]
M = np.float32([[1,0,100],[0,1,50]])
dst = cv2.warpAffine(res,M,(cols,rows))
cv2.imshow('img',dst)
#getRotationMatrix2D? 旋轉(zhuǎn)
M = cv2.getRotationMatrix2D((cols/2,rows/2),90,1)#長寬減半 逆時針90度 縮放1倍
dst2 = cv2.warpAffine(res,M,(cols,rows))
cv2.imshow('img',dst2)
#flip 翻轉(zhuǎn)
dst3=cv2.flip(res,1)#0延x軸翻轉(zhuǎn)? 任意正數(shù)延y軸翻轉(zhuǎn)? 任意負數(shù)延x/y軸同時翻轉(zhuǎn)
cv2.imshow('img',dst3)
#二值化
gray = cv2.cvtColor(res, cv2.COLOR_BGR2GRAY)
ret, binary = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV | cv2.THRESH_OTSU)
cv2.imshow('img',binary)
cv2.waitKey(0)
cv2.destroyAllWindows()
?
?
- 霍夫變換? 圓檢測
def circle_detect(img):
??? gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
??? trans = cv2.GaussianBlur(gray, (9, 9), 0)
??? ret_1, thresh_1 = cv2.threshold(trans, 40, 255, cv2.THRESH_BINARY)
?? circles_2 = cv2.HoughCircles(thresh_1, cv2.HOUGH_GRADIENT, 1, 10, param1=1000, ?????? param2=20)
??? local_2 = circles_2[0][0]
??? x_2 = int(local_2[0])
??? y_2 = int(local_2[1])
??? r_2 = int(local_2[2])
??? img = cv2.circle(img, (x_2, y_2), r_2, (0, 255, 0), 2)
??? n = 10
??? img = cv2.rectangle(img, (x_2 - r_2 - n, y_2 - r_2 - n), (x_2 + r_2 + n, y_2 + r_2 + n), (255, ?? ???????? 255, 0), 3)
??? return img
?
if __name__ == '__main__':
??? img = cv2.imread('rec_cir.jpg')
??? # print(img.shape)
??? circle_detect(img)
??? # cv2.namedWindow('addImage')
??? cv2.imshow('img_add', img)
??? cv2.waitKey(0)
cv2.destroyAllWindows()
?
- 輪廓檢測?? 形狀檢測
需要進一步預處理? 獲取更好的特征
import cv2 as cv
import numpy as np
class ShapeAnalysis:
??? def __init__(self):
??????? self.shapes = {'triangle': 0, 'rectangle': 0, 'polygons': 0, 'circles': 0}
??? def analysis(self, frame):
??????? h, w, ch = frame.shape
??????? result = np.zeros((h, w, ch), dtype=np.uint8)
??????? # 二值化圖像
??????? print("start to detect lines...\n")
??????? gray = cv.cvtColor(frame, cv.COLOR_BGR2GRAY)
??????? trans = cv.GaussianBlur(gray, (9, 9), 0)
??????? ret_1, thresh_1 = cv.threshold(trans, 40, 255, cv.THRESH_BINARY)
??????? # ret, binary = cv.threshold(gray, 0, 255, cv.THRESH_BINARY_INV | cv.THRESH_OTSU)
??????? cv.imshow("input image", frame)
??????? contours, hierarchy = cv. findContours (thresh_1, cv.RETR_EXTERNAL, cv.CHAIN_APPROX_SIMPLE)
??????? for cnt in range(len(contours)):
??????????? # 提取與繪制輪廓
??????????? cv. drawContours (result, contours, cnt, (0, 255, 0), 2)
??????????? # 輪廓逼近
??????????? epsilon = 0.01 * cv.arcLength(contours[cnt], True)
??????????? approx = cv. approxPolyDP (contours[cnt], epsilon, True)
?
??????????? # 分析幾何形狀
??????????? corners = len(approx)
??????????? shape_type = ""
??????????? if corners == 3:
??????????????? count = self.shapes['triangle']
??????????????? count = count+1
??????????????? self.shapes['triangle'] = count
??????????????? shape_type = "三角形"
??????????? if corners == 4:
??????????????? count = self.shapes['rectangle']
??????????????? count = count + 1
??????????????? self.shapes['rectangle'] = count
?????? ?????????shape_type = "矩形"
??????????? if corners >= 10:
??????????????? count = self.shapes['circles']
??????????????? count = count + 1
??????????????? self.shapes['circles'] = count
??????????????? shape_type = "圓形"
??????????? if 4 < corners < 10:
??????????????? count = self.shapes['polygons']
??????????????? count = count + 1
??????????????? self.shapes['polygons'] = count
??????????????? shape_type = "多邊形"
??????????? # 求解中心位置
??????????? mm = cv.moments(contours[cnt])
??????????? cx = int(mm['m10'] / mm['m00'])
??????????? cy = int(mm['m01'] / mm['m00'])
??????????? cv.circle(result, (cx, cy), 3, (0, 0, 255), -1)
??????????? # 顏色分析
??????????? color = frame[cy][cx]
??????????? color_str = "(" + str(color[0]) + ", " + str(color[1]) + ", " + str(color[2]) + ")"
??????????? # 計算面積與周長
??????????? p = cv.arcLength(contours[cnt], True)
??????????? area = cv.contourArea(contours[cnt])
??????????? print("周長: %.3f, 面積: %.3f 顏色: %s 形狀: %s "% (p, area, color_str, shape_type))
??????? cv.imshow("Analysis Result", self.draw_text_info(result))
??????? cv.imwrite("test-result.png", self.draw_text_info(result))
??????? return self.shapes
?
??? def draw_text_info(self, image):
??????? c1 = self.shapes['triangle']
??????? c2 = self.shapes['rectangle']
??????? c3 = self.shapes['polygons']
??????? c4 = self.shapes['circles']
??????? cv.putText(image, "triangle: "+str(c1), (10, 20), cv.FONT_HERSHEY_PLAIN, 1.2, (255, 0, 0), 1)
??????? cv.putText(image, "rectangle: " + str(c2), (10, 40), cv.FONT_HERSHEY_PLAIN, 1.2, (255, 0, 0), 1)
??????? cv.putText(image, "polygons: " + str(c3), (10, 60), cv.FONT_HERSHEY_PLAIN, 1.2, (255, 0, 0), 1)
??????? cv.putText(image, "circles: " + str(c4), (10, 80), cv.FONT_HERSHEY_PLAIN, 1.2, (255, 0, 0), 1)
??????? return image
?
if __name__ == "__main__":
??? src = cv.imread("rec_cir.jpg")
??? ld = ShapeAnalysis()
??? ld.analysis(src)
??? cv.waitKey(0)
cv.destroyAllWindows()
?
- 圖像分割
#去噪求梯度
??? gray = cv2.cvtColor(img_cv2, cv2.COLOR_BGR2GRAY)
??? trans = cv2.GaussianBlur(gray, (9, 9), 0)
??? gradX = cv2.Sobel(gray, ddepth=cv2.CV_32F, dx=1, dy=0)
??? gradY = cv2.Sobel(gray, ddepth=cv2.CV_32F, dx=0, dy=1)
??? gradient = cv2.subtract(gradX, gradY)
??? gradient = cv2.convertScaleAbs(gradient)
?
??? #去噪 二值化
??? blurred = cv2.GaussianBlur(gradient, (9, 9), 0)
??? (_, thresh) = cv2.threshold(blurred, 90, 255, cv2.THRESH_BINARY)
??? #形態(tài) 腐蝕膨脹
??? kernel = cv2.getStructuringElement(cv2.MORPH_ELLIPSE, (25, 25))
??? closed = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, kernel)
??? closed = cv2.erode(closed, None, iterations=4)
??? closed = cv2.dilate(closed, None, iterations=4)
?
?
??? ret_1, binary = cv2.threshold(thresh, 90, 255, cv2.THRESH_BINARY)
??? # ret, binary = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV | cv2.THRESH_OTSU)
??? contours, hierarchy= cv2.findContours(binary, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
?
??? c = sorted(contours, key=cv2.contourArea, reverse=True)[0]
??? # compute the rotated bounding box of the largest contour
??? rect = cv2.minAreaRect(c)
??? box = np.int0(cv2.boxPoints(rect))
??? # draw a bounding box arounded the detected barcode and display the image
??? # draw_img = cv2.drawContours(img_cv2, [box], -1, (0, 0, 255), 3)
??? # cv2.imshow("draw_img", draw_img)
?????????? cv2.drawContours(img_cv2, [box], -1, (0, 0, 255), 3)
??? for cnt in range(len(contours)):
??????? # 提取與繪制輪廓
??????? cv2.drawContours(img_cv2, contours, cnt, (0, 255, 0), 3)
但目標提取效果很好,當圖片出現(xiàn)多目標分割且背景復雜或有噪聲情況下,無法準確分割出目標圖像
?
- createBackgroundSubtractorMOG2背景去除
import numpy as np
import cv2
cap = cv2.VideoCapture(0)
fgbg = cv2.createBackgroundSubtractorMOG2(detectShadows = False)
# fgbg = cv2.createBackgroundSubtractorKNN()
while(cap.isOpened()):
??? ret, frame = cap.read()
??? fgmask = fgbg.apply(frame)
??? cv2.imshow('frame',fgmask)
??? k = cv2.waitKey(30) & 0xff
??? if k == 27:
??????? break
cap.release()
cv2.destroyAllWindows()
更多文章、技術交流、商務合作、聯(lián)系博主
微信掃碼或搜索:z360901061
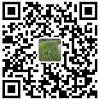
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
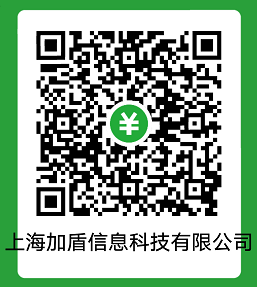