decimal 模塊為快速正確舍入的十進制浮點運算提供支持。
模塊設計以三個概念為中心:十進制數,算術上下文和信號。
-
十進制數是不可變的。 它有一個符號,系數數字和一個指數。 為了保持重要性,系數數字不會截斷尾隨零。十進制數也包括特殊值,例如 Infinity ,-Infinity ,和 NaN 。 該標準還區分 -0 和 +0 。
-
算術的上下文是指定精度、舍入規則、指數限制、指示操作結果的標志以及確定符號是否被視為異常的陷阱啟用器的環境。 舍入選項包括 ROUND_CEILING 、 ROUND_DOWN 、 ROUND_FLOOR 、 ROUND_HALF_DOWN, ROUND_HALF_EVEN 、 ROUND_HALF_UP 、 ROUND_UP 以及 ROUND_05UP.
-
信號是在計算過程中出現的異常條件組。 根據應用程序的需要,信號可能會被忽略,被視為信息,或被視為異常。 十進制模塊中的信號有:Clamped 、 InvalidOperation 、 DivisionByZero 、 Inexact 、 Rounded 、 Subnormal 、 Overflow 、 Underflow 以及 FloatOperation 。
-
對于每個信號,都有一個標志和一個陷阱啟動器。 遇到信號時,其標志設置為 1 ,然后,如果陷阱啟用器設置為 1 ,則引發異常。 標志是粘性的,因此用戶需要在監控計算之前重置它們。
快速入門教程
通常使用小數的開始是導入模塊,使用
getcontext()
查看當前上下文,并在必要時為精度、舍入或啟用的陷阱設置新值:
>>
>
from
decimal
import
*
>>
>
getcontext
(
)
Context
(
prec
=
28
,
rounding
=
ROUND_HALF_EVEN
,
Emin
=
-
999999
,
Emax
=
999999
,
capitals
=
1
,
clamp
=
0
,
flags
=
[
]
,
traps
=
[
Overflow
,
DivisionByZero
,
InvalidOperation
]
)
>>
>
getcontext
(
)
.
prec
=
7
# Set a new precision
可以從整數、字符串、浮點數或元組構造十進制實例。 從整數或浮點構造將執行該整數或浮點值的精確轉換。 十進制數包括特殊值,例如 NaN 代表“非數字”,正的和負的 Infinity,和 -0
>>
>
getcontext
(
)
.
prec
=
28
>>
>
Decimal
(
10
)
Decimal
(
'10'
)
>>
>
Decimal
(
'3.14'
)
Decimal
(
'3.14'
)
>>
>
Decimal
(
3.14
)
Decimal
(
'3.140000000000000124344978758017532527446746826171875'
)
>>
>
Decimal
(
(
0
,
(
3
,
1
,
4
)
,
-
2
)
)
Decimal
(
'3.14'
)
>>
>
Decimal
(
str
(
2.0
**
0.5
)
)
Decimal
(
'1.4142135623730951'
)
>>
>
Decimal
(
2
)
**
Decimal
(
'0.5'
)
Decimal
(
'1.414213562373095048801688724'
)
>>
>
Decimal
(
'NaN'
)
Decimal
(
'NaN'
)
>>
>
Decimal
(
'-Infinity'
)
Decimal
(
'-Infinity'
)
新 Decimal 的重要性僅由輸入的位數決定。 上下文精度和舍入僅在算術運算期間發揮作用。
>>
>
getcontext
(
)
.
prec
=
6
>>
>
Decimal
(
'3.0'
)
Decimal
(
'3.0'
)
>>
>
Decimal
(
'3.1415926535'
)
Decimal
(
'3.1415926535'
)
>>
>
Decimal
(
'3.1415926535'
)
+
Decimal
(
'2.7182818285'
)
Decimal
(
'5.85987'
)
>>
>
getcontext
(
)
.
rounding
=
ROUND_UP
>>
>
Decimal
(
'3.1415926535'
)
+
Decimal
(
'2.7182818285'
)
Decimal
(
'5.85988'
)
Decimal 也可以使用一些數學函數
>>
>
getcontext
(
)
.
prec
=
28
>>
>
Decimal
(
2
)
.
sqrt
(
)
Decimal
(
'1.414213562373095048801688724'
)
>>
>
Decimal
(
1
)
.
exp
(
)
Decimal
(
'2.718281828459045235360287471'
)
>>
>
Decimal
(
'10'
)
.
ln
(
)
Decimal
(
'2.302585092994045684017991455'
)
>>
>
Decimal
(
'10'
)
.
log10
(
)
Decimal
(
'1'
)
設定有效數字的方法
from
decimal
import
*
getcontext
(
)
.
prec
=
6
Decimal
(
1
)
/
Decimal
(
7
)
# 結果為Decimal('0.142857'),六個有效數字
quantize() 方法
quantize() 方法將數字四舍五入為固定指數。 此方法對于 將結果舍入到固定的位置 的貨幣應用程序非常有用:
bb
=
decimal
.
Decimal
(
'7.325'
)
.
quantize
(
decimal
.
Decimal
(
'.01'
)
,
decimal
.
ROUND_UP
)
print
(
bb
)
b
=
decimal
.
Decimal
(
'7.325'
)
.
quantize
(
decimal
.
Decimal
(
'.01'
)
,
decimal
.
ROUND_DOWN
)
print
(
b
)
輸出:
7.33
7.32
Rounding modes
-
decimal.ROUND_CEILING
Round towards Infinity. -
decimal.ROUND_DOWN
Round towards zero. -
decimal.ROUND_FLOOR
Round towards -Infinity. -
decimal.ROUND_HALF_DOWN
Round to nearest with ties going towards zero. -
decimal.ROUND_HALF_EVEN
Round to nearest with ties going to nearest even integer. -
decimal.ROUND_HALF_UP
Round to nearest with ties going away from zero. -
decimal.ROUND_UP
Round away from zero. -
decimal.ROUND_05UP
Round away from zero if last digit after rounding towards zero would have been 0 or 5; otherwise round towards zero.
Context objects
decimal.getcontext()
Return the current context for the active thread.
decimal.setcontext(c)
Set the current context for the active thread to c.
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
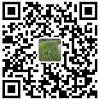
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
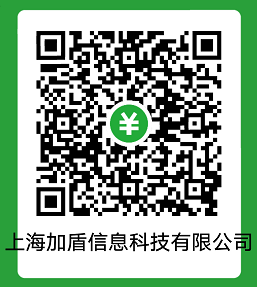