Pythond 的函數是由一個新的語句編寫,即def,def是可執行的語句--函數并不存在,直到Python運行了def后才存在。
函數是通過賦值傳遞的,參數通過賦值傳遞給函數
def語句將創建一個函數對象并將其賦值給一個變量名,def語句的一般格式如下:
def function_name(arg1,arg2[,...]):
??? statement
[return value]?
返回值不是必須的,如果沒有return語句,則Python默認返回值None。
函數名的命名規則:
函數名必須以下劃線或字母開頭,可以包含任意字母、數字或下劃線的組合。不能使用任何的標點符號;
函數名是區分大小寫的。
函數名不能是保留字。
Python使用名稱空間的概念存儲對象,這個名稱空間就是對象作用的區域, 不同對象存在于不同的作用域。下面是不同對象的作用域規則:
每個模塊都有自已的全局作用域。
函數定義的對象屬局部作用域,只在函數內有效,不會影響全局作用域中的對象。
賦值對象屬局部作用域,除非使用global關鍵字進行聲明。
LGB規則是Python查找名字的規則,下面是LGB規則:
1.大多數名字引用在三個作用域中查找:先局部(Local),次之全局(Global),再次之內置(Build-in)。
>>> a=2
>>> b=2
>>> def test(b):
...???? test=a*b
...???? return test
>>>print test(10)
20
b在局部作用域中找到,a在全局作用域中找到。
2.如想在局部作用域中改變全局作用域的對象,必須使用global關鍵字。
#沒用global時的情況
>>> name="Jims"
>>> def set():
...???? name="ringkee"
...
>>> set()
>>> print name
Jims
#使用global后的情況
>>> name="Jims"
>>> def set1():
...???? global name
...???? name="ringkee"
...
>>> set1()
>>> print name
ringkee
3.'global'聲明把賦值的名字映射到一個包含它的模塊的作用域中。
函數的參數是函數與外部溝通的橋梁,它可接收外部傳遞過來的值。參數傳遞的規則如下:
4.在一個函數中對參數名賦值不影響調用者。
>>> a=1
>>> def test(a):
...???? a=a+1
...???? print a
...
>>> test(a)
2
>>> a
1???????????? # a值不變
5.在一個函數中改變一個可變的對象參數會影響調用者。
>>> a=1
>>> b=[1,2]
>>> def test(a,b):
...???? a=5
...???? b[0]=4
...???? print a,b
...
>>> test(a,b)
5 [4, 2]
>>> a
1
>>> b
[4, 2]??? # b值已被更改
參數是對象指針,無需定義傳遞的對象類型。如:
>>> def test(a,b):
...???? return a+b
...
>>> test(1,2)?? #數值型
3
>>> test("a","b")?? #字符型
'ab'
>>> test([12],[11])?? #列表
[12, 11]
函數中的參數接收傳遞的值,參數可分默認參數,如:
def function(ARG=VALUE)
元組(Tuples)參數:
def function(*ARG)
字典(dictionary)參數:
def function(**ARG)
一些函數規則:
默認值必須在非默認參數之后;
在單個函數定義中,只能使用一個tuple參數(*ARG)和一個字典參數(**ARG)。
tuple參數必須在連接參數和默認參數之后。
字典參數必須在最后定義。
1.常用函數
1.abs(x)
abs()返回一個數字的絕對值。如果給出復數,返回值就是該復數的模。
>>>print abs(-100)
100
>>>print abs(1+2j)
2.2360679775
2.callable(object)
callable()函數用于測試對象是否可調用,如果可以則返回1(真);否則返回0(假)。可調用對象包括函數、方法、代碼對象、類和已經定義了“調用”方法的類實例。
>>> a="123"
>>> print callable(a)
0
>>> print callable(chr)
1
3.cmp(x,y)
cmp()函數比較x和y兩個對象,并根據比較結果返回一個整數,如果x
>>>a=1
>>>b=2
>>>c=2
>>> print cmp(a,b)
-1
>>> print cmp(b,a)
1
>>> print cmp(b,c)
0
4.divmod(x,y)
divmod(x,y)函數完成除法運算,返回商和余數。
>>> divmod(10,3)
(3, 1)
>>> divmod(9,3)
(3, 0)
5.isinstance(object,class-or-type-or-tuple) -> bool
測試對象類型
>>> a='isinstance test'
>>> b=1234
>>> isinstance(a,str)
True
>>> isinstance(a,int)
False
>>> isinstance(b,str)
False
>>> isinstance(b,int)
True
下面的程序展示了isinstance函數的使用:
def displayNumType(num):
??? print num, 'is',
??? if isinstance(num, (int, long, float, complex)):
??????? print 'a number of type:', type(num).__name__
??? else:
??????? print 'not a number at all!!!'
displayNumType(-69)
displayNumType(9999999999999999999999999L)
displayNumType(565.8)
displayNumType(-344.3+34.4j)
displayNumType('xxx')
代碼運行結果如下:
-69 is a number of type: int
9999999999999999999999999 is a number of type: long
565.8 is a number of type: float
(-344.3+34.4j) is a number of type: complex
xxx is not a number at all!!!
6.len(object) -> integer
len()函數返回字符串和序列的長度。
>>> len("aa")
2
>>> len([1,2])
2
7.pow(x,y[,z])
pow()函數返回以x為底,y為指數的冪。如果給出z值,該函數就計算x的y次冪值被z取模的值。
>>> print pow(2,4)
16
>>> print pow(2,4,2)
0
>>> print pow(2.4,3)
13.824
8.range([lower,]stop[,step])
range()函數可按參數生成連續的有序整數列表。
>>> range(10)
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> range(1,10)
[1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> range(1,10,2)
[1, 3, 5, 7, 9]
9.round(x[,n])
round()函數返回浮點數x的四舍五入值,如給出n值,則代表舍入到小數點后的位數。
>>> round(3.333)
3.0
>>> round(3)
3.0
>>> round(5.9)
6.0
10.type(obj)
type()函數可返回對象的數據類型。
>>> type(a)
>>> type(copy)
>>> type(1)
11.xrange([lower,]stop[,step])
xrange()函數與range()類似,但xrnage()并不創建列表,而是返回一個xrange對象,它的行為與列表相似,但是只在需要時才計算列表值,當列表很大時,這個特性能為我們節省內存。
>>> a=xrange(10)
>>> print a[0]
0
>>> print a[1]
1
>>> print a[2]
2
2.內置類型轉換函數
1.chr(i)
chr()函數返回ASCII碼對應的字符串。
>>> print chr(65)
A
>>> print chr(66)
B
>>> print chr(65)+chr(66)
AB
2.complex(real[,imaginary])
complex()函數可把字符串或數字轉換為復數。
>>> complex("2+1j")
(2+1j)
>>> complex("2")
(2+0j)
>>> complex(2,1)
(2+1j)
>>> complex(2L,1)
(2+1j)
3.float(x)
float()函數把一個數字或字符串轉換成浮點數。
>>> float("12")
12.0
>>> float(12L)
12.0
>>> float(12.2)
12.199999999999999
4.hex(x)
hex()函數可把整數轉換成十六進制數。
>>> hex(16)
'0x10'
>>> hex(123)
'0x7b'
5.long(x[,base])
long()函數把數字和字符串轉換成長整數,base為可選的基數。
>>> long("123")
123L
>>> long(11)
11L
6.list(x)
list()函數可將序列對象轉換成列表。如:
>>> list("hello world")
['h', 'e', 'l', 'l', 'o', ' ', 'w', 'o', 'r', 'l', 'd']
>>> list((1,2,3,4))
[1, 2, 3, 4]
7.int(x[,base])
int()函數把數字和字符串轉換成一個整數,base為可選的基數。
>>> int(3.3)
3
>>> int(3L)
3
>>> int("13")
13
>>> int("14",15)
19
8.min(x[,y,z...])
min()函數返回給定參數的最小值,參數可以為序列。
>>> min(1,2,3,4)
1
>>> min((1,2,3),(2,3,4))
(1, 2, 3)
9.max(x[,y,z...])
max()函數返回給定參數的最大值,參數可以為序列。
>>> max(1,2,3,4)
4
>>> max((1,2,3),(2,3,4))
(2, 3, 4)
10.oct(x)
oct()函數可把給出的整數轉換成八進制數。
>>> oct(8)
'010'
>>> oct(123)
'0173'
11.ord(x)
ord()函數返回一個字符串參數的ASCII碼或Unicode值。
>>> ord("a")
97
>>> ord(u"a")
97
12.str(obj)
str()函數把對象轉換成可打印字符串。
>>> str("4")
'4'
>>> str(4)
'4'
>>> str(3+2j)
'(3+2j)'
13.tuple(x)
tuple()函數把序列對象轉換成tuple。
>>> tuple("hello world")
('h', 'e', 'l', 'l', 'o', ' ', 'w', 'o', 'r', 'l', 'd')
>>> tuple([1,2,3,4])
(1, 2, 3, 4)
3.序列處理函數
1.常用函數中的len()、max()和min()同樣可用于序列。
2.filter(function,list)
調用filter()時,它會把一個函數應用于序列中的每個項,并返回該函數返回真值時的所有項,從而過濾掉返回假值的所有項。
>>> def nobad(s):
...???? return s.find("bad") == -1
...
>>> s = ["bad","good","bade","we"]
>>> filter(nobad,s)
['good', 'we']
這個例子通過把nobad()函數應用于s序列中所有項,過濾掉所有包含“bad”的項。
3.map(function,list[,list])
map()函數把一個函數應用于序列中所有項,并返回一個列表。
>>> import string
>>> s=["python","zope","linux"]
>>> map(string.capitalize,s)
['Python', 'Zope', 'Linux']
map()還可同時應用于多個列表。如:
>>> import operator
>>> s=[1,2,3]; t=[3,2,1]
>>> map(operator.mul,s,t)?? # s[i]*t[j]
[3, 4, 3]
如果傳遞一個None值,而不是一個函數,則map()會把每個序列中的相應元素合并起來,并返回該元組。如:
>>> a=[1,2];b=[3,4];c=[5,6]
>>> map(None,a,b,c)
[(1, 3, 5), (2, 4, 6)]
4.reduce(function,seq[,init])
reduce()函數獲得序列中前兩個項,并把它傳遞給提供的函數,獲得結果后再取序列中的下一項,連同結果再傳遞給函數,以此類推,直到處理完所有項為止。
>>> import operator
>>> reduce(operator.mul,[2,3,4,5])? # ((2*3)*4)*5
120
>>> reduce(operator.mul,[2,3,4,5],1) # (((1*2)*3)*4)*5
120
>>> reduce(operator.mul,[2,3,4,5],2)? # (((2*2)*3)*4)*5
240
5.zip(seq[,seq,...])
zip()函數可把兩個或多個序列中的相應項合并在一起,并以元組的格式返回它們,在處理完最短序列中的所有項后就停止。
>>> zip([1,2,3],[4,5],[7,8,9])
[(1, 4, 7), (2, 5, 8)]
如果參數是一個序列,則zip()會以一元組的格式返回每個項,如:
>>> zip((1,2,3,4,5))
[(1,), (2,), (3,), (4,), (5,)]
>>> zip([1,2,3,4,5])
[(1,), (2,), (3,), (4,), (5,)]
4.其他
def語句是實時執行的,當它運行的時候,它創建并將一個新的函數對象賦值給一個變量名,Python所有的語句都是實時執行的,沒有像獨立的編譯時間這樣的流程
由于是語句,def可以出現在任一語句可以出現的地方--甚至是嵌套在其他語句中:
if test:
??? def fun():
??????? ...
else:
??? def func():
??????? ...
...
func()
可以將函數賦值給一個不同的變量名,并通過新的變量名進行調用:
othername=func()
othername()
創建函數
內建的callable函數可以用來判斷函數是否可調用:
>>> import math
>>> x=1
>>> y=math.sqrt
>>> callable(x)
False
>>> callable(y)
True
使用del語句定義函數:
>>> def hello(name):
? ? return 'Hello, '+name+'!'
>>> print hello('world')
Hello, world!
>>> print hello('Gumby')
Hello, Gumby!
編寫一個fibnacci數列函數:
>>> def fibs(num):
?? result=[0,1]
??? for i in range(num-2):
?????? result.append(result[-2]+result[-1])
?? return result
>>> fibs(10)
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
>>> fibs(15)
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377]
在函數內為參數賦值不會改變外部任何變量的值:
>>> def try_to_change(n):
? ? n='Mr.Gumby'
>>> name='Mrs.Entity'
>>> try_to_change(name)
>>> name
'Mrs.Entity'
由于字符串(以及元組和數字)是不可改變的,故做參數的時候也就不會改變,但是如果將可變的數據結構如列表用作參數的時候會發生什么:
>>> name='Mrs.Entity'
>>> try_to_change(name)
>>> name
'Mrs.Entity'
>>> def change(n):
?? n[0]='Mr.Gumby'
>>> name=['Mrs.Entity','Mrs.Thing']
>>> change(name)
>>> name
['Mr.Gumby', 'Mrs.Thing']
參數發生了改變,這就是和前面例子的重要區別
以下不用函數再做一次:
>>> name=['Mrs.Entity','Mrs.Thing']
>>> n=name? #再來一次,模擬傳參行為
>>> n[0]='Mr.Gumby' #改變列表
>>> name
['Mr.Gumby', 'Mrs.Thing']
當2個變量同時引用一個列表的時候,它們的確是同時引用一個列表,想避免這種情況,可以復制一個列表的副本,當在序列中做切片的時候,返回的切片總是一個副本,所以復制了整個列表的切片,將會得到一個副本:
>>> names=['Mrs.Entity','Mrs.Thing']
>>> n=names[:]
>>> n is names
False
>>> n==names
True
此時改變n不會影響到names:
>>> n[0]='Mr.Gumby'
>>> n
['Mr.Gumby', 'Mrs.Thing']
>>> names
['Mrs.Entity', 'Mrs.Thing']
>>> change(names[:])
>>> names
['Mrs.Entity', 'Mrs.Thing']
關鍵字參數和默認值
參數的順序可以通過給參數提供參數的名字(但是參數名和值一定要對應):
>>> def hello(greeting, name):
??????? print '%s,%s!'%(greeting, name)
>>> hello(greeting='hello',name='world!')
hello,world!!
關鍵字參數最厲害的地方在于可以在參數中給參數提供默認值:
>>> def hello_1(greeting='hello',name='world!'):
??? print '%s,%s!'%(greeting,name)
>>> hello_1()
hello,world!!
>>> hello_1('Greetings')
Greetings,world!!
>>> hello_1('Greeting','universe')
Greeting,universe!
若想讓greeting使用默認值:
>>> hello_1(name='Gumby')
hello,Gumby!
可以給函數提供任意多的參數,實現起來也不難:
>>> def print_params(*params):
?? print params
>>> print_params('Testing')
('Testing',)
>>> print_params(1,2,3)
(1, 2, 3)
混合普通參數:
>>> def print_params_2(title,*params):
?? print title
?? print params
>>> print_params_2('params:',1,2,3)
params:
(1, 2, 3)
>>> print_params_2('Nothing:')
Nothing:
()
?星號的意思就是“收集其余的位置參數”,如果不提供任何供收集的元素,params就是個空元組
但是不能處理關鍵字參數:
>>> print_params_2('Hmm...',something=42)
Traceback (most recent call last):
? File "
??? print_params_2('Hmm...',something=42)
TypeError: print_params_2() got an unexpected keyword argument 'something'
試試使用“**”:
>>> def print_params(**params):
?? print params
>>> print_params(x=1,y=2,z=3)
{'y': 2, 'x': 1, 'z': 3}
>>> def parames(x,y,z=3,*pospar,**keypar):
?? print x,y,z
?? print pospar
?? print keypar
>>> parames(1,2,3,5,6,7,foo=1,bar=2)
1 2 3
(5, 6, 7)
{'foo': 1, 'bar': 2}
>>> parames(1,2)
1 2 3
()
{}
>>> def print_params_3(**params):
?? print params
>>> print_params_3(x=1,y=2,z=3)
{'y': 2, 'x': 1, 'z': 3}
>>> #返回的是字典而不是元組
>>> #組合‘#'與'##'
>>> def print_params_4(x,y,z=3,*pospar,**keypar):
?? print x,y,z
?? print pospar
?? print keypar
>>> print_params_4(1,2,3,5,6,7,foo=1,bar=2)
1 2 3
(5, 6, 7)
{'foo': 1, 'bar': 2}
>>> print_params_4(1,2)
1 2 3
()
{}
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
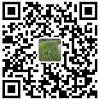
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
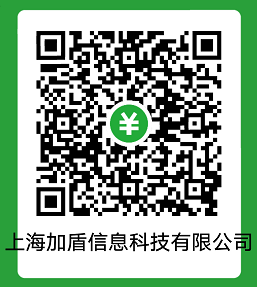