12.324 Django ORM常用字段
1 .id = models.AutoField(primary_key = True):int自增列,必須填入參數 primary_key = True。當model中如果沒有自增列,則自動會創建一個列名為id的列。 2 .IntegerField:一個整數類型,范圍在 - 2147483648 to 2147483647 3 .name = models.CharField(max_length = 32 ):varchar字符類型,必須提供max_length參數, max_length表示字符長度 4 .title = models.DateField( null = True):日期字段,日期格式 YYYY - MM - DD,相當于Python中的datetime.date()實例 5 .title = models.DateTimeField( null = True):日期時間字段,格式 YYYY - MM - DD HH:MM [ :ss[.uuuuuu ] ] [ TZ ] ,相當于Python中的datetime. datetime ()實例 6 .TimeField(DateTimeCheckMixin, Field): - 時間格式 HH:MM [ :ss[.uuuuuu ] ] 7 .DurationField(Field): - 長整數,時間間隔,數據庫中按照bigint存儲,ORM中獲取的值為datetime.timedelta類型 8 .TextField(Field): - 文本類型 9 .FilePathField(Field): - 字符串,Django Admin以及ModelForm中提供讀取文件夾下文件的功能 - 參數: path, 文件夾路徑 match = None, 正則匹配 recursive = False, 遞歸下面的文件夾 allow_files = True, 允許文件 allow_folders = False, 允許文件夾 10 .FileField(Field): - 字符串,路徑保存在數據庫,文件上傳到指定目錄 - 參數: upload_to = "" 上傳文件的保存路徑 storage = None 存儲組件,默認django.core.files.storage.FileSystemStorage 11 .ImageField(FileField): - 字符串,路徑保存在數據庫,文件上傳到指定目錄 - 參數: upload_to = "" 上傳文件的保存路徑 storage = None 存儲組件,默認django.core.files.storage.FileSystemStorage width_field = None, 上傳圖片的高度保存的數據庫字段名(字符串) height_field = None 上傳圖片的寬度保存的數據庫字段名(字符串) 12 .FloatField(Field): - 浮點型 13 .DecimalField(Field): - 10進制小數 - 參數:max_digits,總長度 decimal_places,小數位長度 14 .BinaryField(Field): - 二進制類型
自定義字段:
自定義char類型字段:
class FixedCharField(models.Field): def __init__ (self, max_length, *args, ** kwargs): super(). __init__ (max_length=max_length, *args, ** kwargs) self.length = max_length ? def db_type(self, connection): """ 限定生成數據庫表的字段類型為char,長度為length指定的值 """ return ' char(%s) ' % self.length ? class Class(models.Model): id = models.AutoField(primary_key= True) title = models.CharField(max_length=25 ) # 使用上面自定義的char類型的字段 cname = FixedCharField(max_length=25)
自定義無符號的int類型:
class UnsignedIntegerField(models.IntegerField): def db_type(self, connection): return ' integer UNSIGNED ' class Class(models.Model): id = models.AutoField(primary_key= True) title = models.CharField(max_length=25 ) # 使用上面自定義的無符號的int類型的字段 cid = UnsignedIntegerField()
12.325 字段參數
title = models.DateField( null = True):用于表示某個字段可以為空 title = models.DateField( unique = True):如果設置為unique = True,則該字段在此表中必須是唯一的 title = models.DateField(db_index = True):如果db_index = True,則代表著為此字段設置數據庫索引 price = models.DecimalField(max_digits = 5 , decimal_places = 2 , default = 9.9 ):為該字段設置默認值 ? DatetimeField、DateField、TimeField: publisher_date = models.DateField(auto_now_add): 配置auto_now_add = True,創建數據記錄的時候會把當前時間添加到數據庫 publisher_date = models.DateField(auto_now): 配置上auto_now = True,每次更新數據記錄的時候會更新該字段
12.326關系字段(ForeignKey)
外鍵類型在ORM中用來表示外鍵關聯關系,一般把ForeignKey字段設置在 '一對多'中'多'的一方。ForeignKey可以和其他表做關聯關系同時也可以和自身做關聯關系。
ForeignKey字段參數:
publisher = models.ForeignKey( to = ' Publisher ' , tofield = ' id ' on_delete = models. CASCADE , db_constraint = False) 1 . to :設置要關聯的表 2 .to_field:設置要關聯的表的字段、 3 .related_name:反向操作時,使用的字段名,用于代替原反向查詢時的 ' 表名_set ' 4 .related_query_name:反向查詢操作時,使用的連接前綴,用于替換表名 5 .on_delete = models. CASCADE :當刪除關聯表中的數據時,當前表與其關聯的行的行為,models.CASCADE刪除關聯數據,與之關聯也刪除( 1 .1版本默認級聯刪除, 2 .0版本后必須手動添加) 6 .on_delete = models. SET ()刪除關聯數據, a. 與之關聯的值設置為指定值,設置:models. SET (值) b. 與之關聯的值設置為可執行對象的返回值,設置:models. SET (可執行對象) def func(): return 10 class MyModel(models.Model): user = models.ForeignKey( to = " User ", to_field = "id", on_delete = models. SET (func) ) 7 .db_constraint:是否在數據庫中設置外鍵約束關系,默認為True,設置成False,數據庫中不體現約束關系,相當于普通字段
示例:
class Classes(models.Model): name = models.CharField(max_length=32 ) ? class Student(models.Model): name = models.CharField(max_length=32 ) theclass = models.ForeignKey(to= " Classes " )
當我們要查詢某個班級關聯的所有學生(反向查詢)時,我們會這么寫:
models.Classes.objects.first().student_set.all()
當我們在ForeignKey字段中添加了參數 related_name 后:
class Student(models.Model): name = models.CharField(max_length=32 ) theclass = models.ForeignKey(to= " Classes " , related_name= " students " )
當我們要查詢某個班級關聯的所有學生(反向查詢)時,我們會這么寫:
models.Classes.objects.first().students.all()
12.327OneToOneField
一對一字段:通常一對一字段用來擴展已有字段。
一對一的關聯關系多用在當一張表的不同字段查詢頻次差距過大的情況下,將本可以存儲在一張表的字段拆開放置在兩張表中,然后將兩張表建立一對一的關聯關系。
class Author(models.Model): name = models.CharField(max_length=32 ) gender_choice = ((1, " 男 " ), (2, " 女 " ), (3, " 保密 " )) gender = models.SmallIntegerField(choices=gender_choice, default=3 ) info = models.OneToOneField(to= ' AuthorInfo ' ) class AuthorInfo(models.Model): phone = models.CharField(max_length=11 ) email = models.EmailField(unique=True, db_index= True) 1 .to:設置要關聯的表 2 .to_field:設置要關聯的字段 3.on_delete:同ForeignKey字段
12.328ManyToManyField
用于表示多對多的關聯關系,在數據庫中通過第三張表來建立關聯關系
多對多關聯關系的三種方式:
方式一:自行創建第三張表
class Book(models.Model): title = models.CharField(max_length=32,verbose_name= " 書名 " ) ? class Author(models.Model): name = models.CharField(max_length=32,verbose_name= " 作者姓名 " ) ? # 自己創建第三張表 class Book2Author(models.Model): id = models.AutoField(primary_key= True) book = models.ForeignKey(to= " Book " ) author = models.ForeignKey(to= " Author " ) # first_blood = models.DateField() 自行創建第三章表,便于增加新字段 class Meta: unique_together = ( " author " , " book " ) 1 .unique_together:聯合唯一索引 2 .index_together:聯合索引 3.ordering:指定默認按什么字段排序。只有設置了該屬性,查詢到的結果才可以被reverse()
方式二:通過ManyToManyField自動創建第三張表
class Book(models.Model): title = models.CharField(max_length=32, verbose_name= " 書名 " ) # 通過ORM自帶的ManyToManyField自動創建第三張表 class Author(models.Model): name = models.CharField(max_length=32, verbose_name= " 作者姓名 " ) books = models.ManyToManyField(to= " Book " , db_table= " author2book " ,related_name= " authors " ) class Meta: db_table = " author " 1 .to:設置要關聯的表 2.db_table= " author2book " :指定自動創建的第三張表的表名為author2book,而不是app01_author_book 3.related_name= " authors " :反向操作時,使用的字段名,用于代替原反向查詢時的 ' 表名_set ' ,同ForeignKey字段 4.Meta:db_table,創建author表時指定表名為author,而不是app01_author
方式三:設置ManyTomanyField并指定自行創建的第三張表
class Book(models.Model): title = models.CharField(max_length=32,verbose_name= " 書名 " ) ? class Author(models.Model): name = models.CharField(max_length=32,verbose_name= " 作者姓名 " ) books = models.ManyToManyField( to = " Book " , through = " Book2Author " , through_fields =( ' author ' , ' book ' ) ) # 自己創建第三張表 class Book2Author(models.Model): id = models.AutoField(primary_key= True) book = models.ForeignKey(to= " Book " ) author = models.ForeignKey(to= " Author " ) # first_blood = models.DateField() 自行創建第三章表,便于增加新字段 class Meta: unique_together = ( " author " , " book " ) 1 .to:設置要關聯的表 2.through_fields:設置關聯的字段,接受一個2元組( ' field1 ' , ' field2 ' ):其中field1是定義ManyToManyField的模型外鍵的名(author),field2是關聯目標模型(book)的外鍵名,表示第三張表author,book字段是描述多對多關系的,其他的字段的ForeignKey是單純的多對一外鍵。 3.through:在使用ManyToManyField字段時,Django將自動生成一張表來管理多對多的關聯關系。但我們也可以手動創建第三張表來管理多對多關系,此時就需要通過through來指定第三張表的表名。
注意:
但是當我們使用第三種方式創建多對多關聯關系時,就 無法使用set、add、remove、clear 方法來管理多對多的關系了,只能使用all方法拿到對象,需要通過第三張表的 model 來管理多對多關系。
12.3281使用方式一或方式三的查詢和其他操作
查詢操作:
# 找到第一本書 ret = models.Book.objects.first().author2book_set.all().values( " author__name " ) ret = models.Book.objects.filter(id=1).values( " author2book__author__name " )
增刪改操作:
first_book = models.Book.objects.first() # 改:first_book.author.add(1): models.Author2Book.objects.create(book=first_book, author_id=1 ) # 清空:first_book.author.clear() models.Author2Book.objects.filter(book= first_book).delete() # 刪除:first_book.author.remove(3) models.Author2Book.objects.filter(book=first_book, author_id=3 ).delete() # 查:first_book.author.all() 方式三可用 models.Author2Book.objects.filter(book=first_book)
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
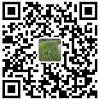
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
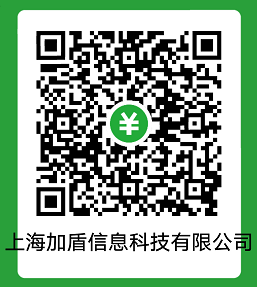