官方解釋:
Apply function of two arguments cumulatively to the items of iterable, from left to right, so as to reduce the iterable to a single value. For example, reduce(lambda x, y: x+y, [1, 2, 3, 4, 5]) calculates ((((1+2)+3)+4)+5). The left argument, x, is the accumulated value and the right argument, y, is the update value from the iterable. If the optional initializer is present, it is placed before the items of the iterable in the calculation, and serves as a default when the iterable is empty. If initializer is not given and iterable contains only one item, the first item is returned. Roughly equivalent to:
意思就是說:將一個可迭代的對象應用到一個帶有兩個參數的方法上,我們稱之為appFun,遍歷這個可迭代對象,將其中的元素依次作為appFun的參數,但這個函數有兩個參數,作為哪個參數呢?有這樣的規則,看一下下面reduce方法的實現,有三個參數,第一個參數就是上面說的appFun,第二個參數就是那個可迭代的對象,而第三個呢?當調用reduce方法的時候給出了initializer這個參數,那么第一次調用appFun的時候這個參數值就作為第一個參數,而可迭代對象的元素依次作為appFun的第二個參數;如果調用reduce的時候沒有給出initializer這個參數,那么第一次調用appFun的時候,可迭代對象的第一個元素就作為appFun的第一個元素,而可迭代器的從第二個元素到最后依次作為appFun的第二個參數,除第一次調用之外,appFun的第一個參數就是appFun的返回值了。例如reduce(lambda x, y: x+y, [1, 2, 3, 4, 5]),計算1到5的和,因為沒有給定initializer參數,所以第一次調用x+y時,x=1,即列表的第一個元素,y=2,即列表的第二個元素,之后返回的1+2的結果作為第二次調用x+y中的x,即上一次的結果,y=2,即第二個元素,依次類推,知道得到1+2+3+4+5的結果。
這樣看來,其實下面的代碼定義是有一點問題,我們在程序中調用這段代碼reduce(lambda x, y: x+y, [1, 2, 3, 4, 5]),得到的結果為16,而正確的結果為15,問題在于如果集合不是以0開始,那么按照如下代碼,第一次調用x=1,即第一個元素,y也是等于1,也是第一個元素,而正確的y應該是2。所以真正的reduce方法應該和下面的例子是有差別的。
def reduce(function, iterable, initializer=None): it = iter(iterable) if initializer is None: try: initializer = next(it) except StopIteration: raise TypeError('reduce() of empty sequence with no initial value') accum_value = initializer for x in iterable: accum_value = function(accum_value, x) return accum_value
那么reduce函數能做什么,什么情況下要用reduce呢,看下面的例子:
例如上面的例子,實現一個整形集合的累加。假設lst = [1,2,3,4,5],實現累加的方式有很多:
第一種:用sum函數 。
sum(lst)
?
第二種:循環方式。
def customer_sum(lst): result = 0 for x in lst: result+=x return result #或者 def customer_sum(lst): result = 0 while lst: temp = lst.pop(0) result+=temp return result if __name__=="__main__": lst = [1,2,3,4,5] print customer_sum(lst)
第三種:遞推求和
def add(lst,result): if lst: temp = lst.pop(0) temp+=result return add(lst,temp) else: return result if __name__=="__main__": lst = [1,2,3,4,5] print add(lst,0)
第四種:reduce方式
lst = [1,2,3,4,5] print reduce(lambda x,y:x+y,lst) #這種方式用lambda表示當做參數,因為沒有提供reduce的第三個參數,所以第一次執行時x=1,y=2,第二次x=1+2,y=3,即列表的第三個元素 #或者 lst = [1,2,3,4,5] print reduce(lambda x,y:x+y,lst,0) #這種方式用lambda表示當做參數,因為指定了reduce的第三個參數為0,所以第一次執行時x=0,y=1,第二次x=0+1,y=2,即列表的第二個元素, 假定指定reduce的第三個參數為100,那么第一次執行x=100,y仍然是遍歷列表的元素,最后得到的結果為115 #或者 def add(x,y): return x+y print reduce(add, lst) #與方式1相同,只不過把lambda表達式換成了自定義函數 #或者 def add(x,y): return x+y print reduce(add, lst,0) #與方式2相同,只不過把lambda表達式換成了自定義函數
?
再舉一個例子:有一個序列集合,例如[1,1,2,3,2,3,3,5,6,7,7,6,5,5,5],統計這個集合所有鍵的重復個數,例如1出現了兩次,2出現了兩次等。大致的思路就是用字典存儲,元素就是字典的key,出現的次數就是字典的value。方法依然很多
第一種:for循環判斷
def statistics(lst): dic = {} for k in lst: if not k in dic: dic[k] = 1 else: dic[k] +=1 return dic lst = [1,1,2,3,2,3,3,5,6,7,7,6,5,5,5] print(statistics(lst))
第二種:比較取巧的,先把列表用set方式去重,然后用列表的count方法
def statistics2(lst): m = set(lst) dic = {} for x in m: dic[x] = lst.count(x) return dic lst = [1,1,2,3,2,3,3,5,6,7,7,6,5,5,5] print statistics2(lst)
第三種:用reduce方式
def statistics(dic,k): if not k in dic: dic[k] = 1 else: dic[k] +=1 return dic lst = [1,1,2,3,2,3,3,5,6,7,7,6,5,5,5] print reduce(statistics,lst,{}) #提供第三個參數,第一次,初始字典為空,作為statistics的第一個參數,然后遍歷lst,作為第二個參數,然后將返回的字典集合作為下一次的第一個參數 或者 d = {} d.extend(lst) print reduce(statistics,d) #不提供第三個參數,但是要在保證集合的第一個元素是一個字典對象,作為statistics的第一個參數,遍歷集合依次作為第二個參數
通過上面的例子發現,凡是要對一個集合進行操作的,并且要有一個統計結果的,能夠用循環或者遞歸方式解決的問題,一般情況下都可以用reduce方式實現。
reduce函數真是“一位好同志啊”!
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
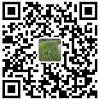
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
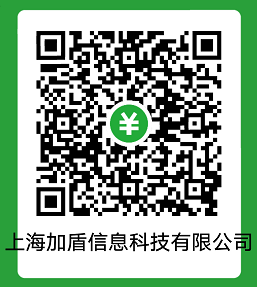