便攜文檔格式 (PDF) 是由 Adobe 開發的格式,主要用于呈現可打印的文檔,其中包含有 pixel-perfect 格式,嵌入字體以及2D矢量圖像。 You can think of a PDF document as the digital equivalent of a printed document; indeed, PDFs are often used in distributing documents for the purpose of printing them.
可以方便的使用 Python 和 Django 生成 PDF 文檔需要歸功于一個出色的開源庫, ReportLab (http://www.reportlab.org/rl_toolkit.html) 。動態生成 PDF 文件的好處是在不同的情況下,如不同的用戶或者不同的內容,可以按需生成不同的 PDF 文件。 The advantage of generating PDF files dynamically is that you can create customized PDFs for different purposes say, for different users or different pieces of content.
下面的例子是使用 Django 和 ReportLab 在 KUSports.com 上生成個性化的可打印的 NCAA 賽程表 (tournament brackets) 。
安裝 ReportLab
在生成 PDF 文件之前,需要安裝 ReportLab 庫。這通常是個很簡單的過程: Its usually simple: just download and install the library from http://www.reportlab.org/downloads.html.
Note
如果使用的是一些新的 Linux 發行版,則在安裝前可以先檢查包管理軟件。 多數軟件包倉庫中都加入了 ReportLab 。
比如,如果使用(杰出的) Ubuntu 發行版,只需要簡單的 apt-get install python-reportlab 一行命令即可完成安裝。
使用手冊(原始的只有 PDF 格式)可以從 http://www.reportlab.org/rsrc/userguide.pdf 下載,其中包含有一些其它的安裝指南。
在 Python 交互環境中導入這個軟件包以檢查安裝是否成功。
>>> import reportlab
如果剛才那條命令沒有出現任何錯誤,則表明安裝成功。
編寫視圖
和 CSV 類似,由 Django 動態生成 PDF 文件很簡單,因為 ReportLab API 同樣可以使用類似文件對象。
下面是一個 Hello World 的示例:
from reportlab.pdfgen import canvas from django.http import HttpResponse def hello_pdf(request): # Create the HttpResponse object with the appropriate PDF headers. response = HttpResponse(mimetype='application/pdf') response['Content-Disposition'] = 'attachment; filename=hello.pdf' # Create the PDF object, using the response object as its "file." p = canvas.Canvas(response) # Draw things on the PDF. Here's where the PDF generation happens. # See the ReportLab documentation for the full list of functionality. p.drawString(100, 100, "Hello world.") # Close the PDF object cleanly, and we're done. p.showPage() p.save() return response
需要注意以下幾點:
??? 這里我們使用的 MIME 類型是 application/pdf 。這會告訴瀏覽器這個文檔是一個 PDF 文檔,而不是 HTML 文檔。 如果忽略了這個參數,瀏覽器可能會把這個文件看成 HTML 文檔,這會使瀏覽器的窗口中出現很奇怪的文字。 If you leave off this information, browsers will probably interpret the response as HTML, which will result in scary gobbledygook in the browser window.
??? 使用 ReportLab 的 API 很簡單: 只需要將 response 對象作為 canvas.Canvas 的第一個參數傳入。
??? 所有后續的 PDF 生成方法需要由 PDF 對象調用(在本例中是 p ),而不是 response 對象。
??? 最后需要對 PDF 文件調用 showPage() 和 save() 方法(否則你會得到一個損壞的 PDF 文件)。
復雜的 PDF 文件
如果您在創建一個復雜的 PDF 文檔(或者任何較大的數據塊),請使用 cStringIO 庫存放臨時生成的 PDF 文件。 cStringIO 提供了一個用 C 編寫的類似文件對象的接口,從而可以使系統的效率最高。
下面是使用 cStringIO 重寫的 Hello World 例子:
from cStringIO import StringIO from reportlab.pdfgen import canvas from django.http import HttpResponse def hello_pdf(request): # Create the HttpResponse object with the appropriate PDF headers. response = HttpResponse(mimetype='application/pdf') response['Content-Disposition'] = 'attachment; filename=hello.pdf' temp = StringIO() # Create the PDF object, using the StringIO object as its "file." p = canvas.Canvas(temp) # Draw things on the PDF. Here's where the PDF generation happens. # See the ReportLab documentation for the full list of functionality. p.drawString(100, 100, "Hello world.") # Close the PDF object cleanly. p.showPage() p.save() # Get the value of the StringIO buffer and write it to the response. response.write(temp.getvalue()) return response
其它的可能性
使用 Python 可以生成許多其它類型的內容,下面介紹的是一些其它的想法和一些可以用以實現它們的庫。 Here are a few more ideas and some pointers to libraries you could use to implement them:
??? ZIP 文件 :Python 標準庫中包含有 zipfile 模塊,它可以讀和寫壓縮的 ZIP 文件。 它可以用于按需生成一些文件的壓縮包,或者在需要時壓縮大的文檔。 如果是 TAR 文件則可以使用標準庫 tarfile 模塊。
??? 動態圖片 : Python 圖片處理庫 (PIL; http://www.pythonware.com/products/pil/) 是極好的生成圖片(PNG, JPEG, GIF 以及其它許多格式)的工具。 它可以用于自動為圖片生成縮略圖,將多張圖片壓縮到單獨的框架中,或者是做基于 Web 的圖片處理。
??? 圖表 : Python 有許多出色并且強大的圖表庫用以繪制圖表,按需地圖,表格等。 我們不可能將它們全部列出,所以下面列出的是個中的翹楚。
??????? matplotlib (http://matplotlib.sourceforge.net/) 可以用于生成通常是由 matlab 或者 Mathematica 生成的高質量圖表。
??????? pygraphviz (https://networkx.lanl.gov/wiki/pygraphviz) 是一個 Graphviz 圖形布局的工具 (http://graphviz.org/) 的 Python 接口,可以用于生成結構化的圖表和網絡。
總之,所有可以寫文件的庫都可以與 Django 同時使用。 The possibilities are immense.
我們已經了解了生成“非HTML”內容的基本知識,讓我們進一步總結一下。 Django擁有很多用以生成各類“非HTML”內容的內置工具。
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
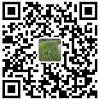
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
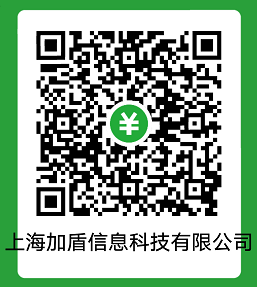