本文將通過一下幾個方面來一一進行解決
????? 1、程序的主要功能
????? 2、實現過程
????? 3、類的定義
????? 4、用生成器generator動態更新每個對象并返回對象
????? 5、使用strip 去除不必要的字符
????? 6、rematch匹配字符串
????? 7、使用timestrptime提取字符串轉化為時間對象
????? 8、完整代碼
程序的主要功能
現在有個存儲用戶信息的像表格一樣的文檔:第一行是屬性,各個屬性用逗號(,)分隔,從第二行開始每行是各個屬性對應的值,每行代表一個用戶。如何實現讀入這個文檔,每行輸出一個用戶對象呢?
另外還有4個小要求:
每個文檔都很大,如果一次性把所有行生成的那么多對象存成列表返回,內存會崩潰。程序中每次只能存一個行生成的對象。
用逗號隔開的每個字符串,前后可能有雙引號(”)或者單引號('),例如”張三“,要把引號去掉;如果是數字,有+000000001.24這樣的,要把前面的+和0都去掉,提取出1.24
文檔中有時間,形式可能是2013-10-29,也可能是2013/10/29 2:23:56 這樣的形式,要把這樣的字符串轉成時間類型
這樣的文檔有好多個,每個的屬性都不一樣,例如這個是用戶的信息,那個是通話紀錄。所以類中的具體屬性有哪些要根據文檔的第一行動態生成
實現過程
1.類的定義
由于屬性是動態添加的,屬性-值 對也是動態添加的,類中要含有
updateAttributes()
和
updatePairs()
兩個成員函數即可,此外用列表
attributes
存儲屬性,詞典
attrilist
存儲映射。其中
init()
函數為構造函數。
__attributes
前有下劃線表示私有變量,不能在外面直接調用。實例化時只需
a=UserInfo()
即可,無需任何參數。
class UserInfo(object): 'Class to restore UserInformation' def __init__ (self): self.attrilist={} self.__attributes=[] def updateAttributes(self,attributes): self.__attributes=attributes def updatePairs(self,values): for i in range(len(values)): self.attrilist[self.__attributes[i]]=values[i]
2.用生成器(generator)動態更新每個對象并返回對象
生成器相當于一個只需要初始化一次,就可自動運行多次的函數,每次循環返回一個結果。不過函數用
return
返回結果,而生成器用
yield
返回結果。每次運行都在
yield
返回,下一次運行從
yield
之后開始。例如,我們實現斐波拉契數列,分別用函數和生成器實現:
def fib(max): n, a, b = 0, 0, 1 while n < max: print(b) a, b = b, a + b n = n + 1 return 'done'
我們計算數列的前6個數:
>>> fib(6) 1 1 2 3 5 8 'done'
如果用生成器的話,只要把
print
改成
yield
就可以了。如下:
def fib(max): n, a, b = 0, 0, 1 while n < max: yield b a, b = b, a + b n = n + 1
使用方法:
>>> f = fib(6) >>> f>>> for i in f: ... print(i) ... 1 1 2 3 5 8 >>>
可以看到,生成器fib本身是個對象,每次執行到yield會中斷返回一個結果,下次又繼續從
yield
的下一行代碼繼續執行。生成器還可以用
generator.next()
執行。
在我的程序中,生成器部分代碼如下:
def ObjectGenerator(maxlinenum): filename='/home/thinkit/Documents/usr_info/USER.csv' attributes=[] linenum=1 a=UserInfo() file=open(filename) while linenum < maxlinenum: values=[] line=str.decode(file.readline(),'gb2312')#linecache.getline(filename, linenum,'gb2312') if line=='': print'reading fail! Please check filename!' break str_list=line.split(',') for item in str_list: item=item.strip() item=item.strip('\"') item=item.strip('\'') item=item.strip('+0*') item=catchTime(item) if linenum==1: attributes.append(item) else: values.append(item) if linenum==1: a.updateAttributes(attributes) else: a.updatePairs(values) yield a.attrilist #change to ' a ' to use linenum = linenum +1
其中,
a=UserInfo()
為類
UserInfo
的實例化.因為文檔是gb2312編碼的,上面使用了對應的解碼方法。由于第一行是屬性,有個函數將屬性列表存入
UserInfo
中,即
updateAttributes();
后面的行則要將 屬性-值 對讀入一個字典中存儲。
p.s.python
中的字典相當于映射(map).
3.使用strip 去除不必要的字符
從上面代碼中,可以看到使用
str.strip(somechar)
即可去除str前后的
somechar
字符。
somechar
可以是符號,也可以是正則表達式,如上:
item=item.strip()#除去字符串前后的所有轉義字符,如\t,\n等 item=item.strip('\"')#除去前后的" item=item.strip('\'') item=item.strip('+0*')#除去前后的+00...00,*表示0的個數可以任意多,也可以沒有
4.re.match匹配字符串
函數語法:
re.match(pattern, string, flags=0)
函數參數說明:
參數?????????? 描述
pattern??? ?? 匹配的正則表達式
string??????? ?要匹配的字符串。
flags????????? 標志位,用于控制正則表達式的匹配方式,如:是否區分大小寫,多行匹配等等。
若匹配成功re.match方法返回一個匹配的對象,否則返回None。`
>>> s='2015-09-18'
>>> matchObj=re.match(r'\d{4}-\d{2}-\d{2}',s, flags= 0)
>>> print matchObj
<_sre.SRE_Match object at 0x7f3525480f38>
1
2
3
4
5
5.使用time.strptime提取字符串轉化為時間對象
在
time
模塊中,
time.strptime(str,format)
可以把
str
按照
format
格式轉化為時間對象,
format
中的常用格式有:
???? %y 兩位數的年份表示(00-99)
???? %Y 四位數的年份表示(000-9999)
???? %m 月份(01-12)
?????%d 月內中的一天(0-31)
???? %H 24小時制小時數(0-23)
???? %I 12小時制小時數(01-12)
???? %M 分鐘數(00=59)
???? %S 秒(00-59)
此外,還需要使用
re
模塊,用正則表達式,對字符串進行匹配,看是否是一般時間的格式,如
YYYY/MM/DD H:M:S, YYYY-MM-DD
等
在上面的代碼中,函數catchTime就是判斷item是否為時間對象,是的話轉化為時間對象。
代碼如下:
import time import re def catchTime(item): # check if it's time matchObj=re.match(r'\d{4}-\d{2}-\d{2}',item, flags= 0) if matchObj!= None : item =time.strptime(item,'%Y-%m-%d') #print "returned time: %s " %item return item else: matchObj=re.match(r'\d{4}/\d{2}/\d{2}\s\d+:\d+:\d+',item,flags=0 ) if matchObj!= None : item =time.strptime(item,'%Y/%m/%d %H:%M:%S') #print "returned time: %s " %item return item
完整代碼:
import collections import time import re class UserInfo(object): 'Class to restore UserInformation' def __init__ (self): self.attrilist=collections.OrderedDict()# ordered self.__attributes=[] def updateAttributes(self,attributes): self.__attributes=attributes def updatePairs(self,values): for i in range(len(values)): self.attrilist[self.__attributes[i]]=values[i] def catchTime(item): # check if it's time matchObj=re.match(r'\d{4}-\d{2}-\d{2}',item, flags= 0) if matchObj!= None : item =time.strptime(item,'%Y-%m-%d') #print "returned time: %s " %item return item else: matchObj=re.match(r'\d{4}/\d{2}/\d{2}\s\d+:\d+:\d+',item,flags=0 ) if matchObj!= None : item =time.strptime(item,'%Y/%m/%d %H:%M:%S') #print "returned time: %s " %item return item def ObjectGenerator(maxlinenum): filename='/home/thinkit/Documents/usr_info/USER.csv' attributes=[] linenum=1 a=UserInfo() file=open(filename) while linenum < maxlinenum: values=[] line=str.decode(file.readline(),'gb2312')#linecache.getline(filename, linenum,'gb2312') if line=='': print'reading fail! Please check filename!' break str_list=line.split(',') for item in str_list: item=item.strip() item=item.strip('\"') item=item.strip('\'') item=item.strip('+0*') item=catchTime(item) if linenum==1: attributes.append(item) else: values.append(item) if linenum==1: a.updateAttributes(attributes) else: a.updatePairs(values) yield a.attrilist #change to ' a ' to use linenum = linenum +1 if __name__ == '__main__': for n in ObjectGenerator(10): print n #輸出字典,看是否正確
總結
以上就是這篇文章的全部內容,希望能對大家的學習或者工作帶來一定幫助,如果有疑問大家可以留言交流,謝謝大家對腳本之家的支持。
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
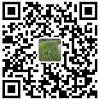
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
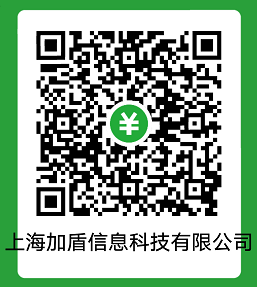