Python實現模擬時鐘代碼推薦
# coding=utf8 import sys, pygame, math, random from pygame.locals import * from datetime import datetime, date, time def print_text(font, x, y, text, color=(255,255,255)): imgtext = font.render(text, True, color) screen.blit(imgtext, (x,y)) def wrap_angle(angle): return abs(angle%360) # main pygame.init() screen = pygame.display.set_mode((600,500)) pygame.display.set_caption("CLOCK") font = pygame.font.Font(None, 36) orange = 220,180,0 white = 255,255,255 yellow = 255,255,0 pink = 255,100,100 pos_x = 300 pos_y = 250 radius = 250 angle = 360 # repeating loop while True: for event in pygame.event.get(): if event.type == QUIT: sys.exit() keys = pygame.key.get_pressed() if keys[K_ESCAPE]: sys.exit() screen.fill((0,0,100)) # draw circle pygame.draw.circle(screen, white, (pos_x,pos_y), radius, 6) # draw the clock number 1-12 for n in range(1,13): angle = math.radians(n*(360/12)-90) x = math.cos(angle)*(radius-20)-10 y = math.sin(angle)*(radius-20)-10 print_text(font, pos_x+x, pos_y+y, str(n)) # get the time of day today = datetime.today() hours = today.hour%12 minutes = today.minute seconds = today.second # draw the hours hand hour_angle = wrap_angle(hours*(360/12)-90) hour_angle = math.radians(hour_angle) hour_x = math.cos(hour_angle)*(radius-80) hour_y = math.sin(hour_angle)*(radius-80) target = (pos_x+hour_x, pos_y+hour_y) pygame.draw.line(screen, pink, (pos_x,pos_y), target, 12) # draw the minutes hand min_angle = wrap_angle(minutes*(360/60)-90) min_angle = math.radians(min_angle) min_x = math.cos(min_angle)*(radius-60) min_y = math.sin(min_angle)*(radius-60) target = (pos_x+min_x, pos_y+min_y) pygame.draw.line(screen, orange, (pos_x,pos_y), target, 12) # draw the seconds hand sec_angle = wrap_angle(seconds*(360/60)-90) sec_angle = math.radians(sec_angle) sec_x = math.cos(sec_angle)*(radius-40) sec_y = math.sin(sec_angle)*(radius-40) target = (pos_x+sec_x, pos_y+sec_y) pygame.draw.line(screen, yellow, (pos_x,pos_y), target, 12) # draw the center pygame.draw.circle(screen, white, (pos_x,pos_y), 20) print_text(font, 0, 0, str(hours) + ":" + str(minutes) + ":" + str(seconds)) pygame.display.update()
再來一個例子
import sys from PyQt4 import QtGui, QtCore from PyQt4.QtCore import Qt from PyQt4.QtCore import QPoint from PyQt4.QtCore import QTimer from PyQt4.QtCore import QTime from PyQt4.QtGui import QPainter from PyQt4.QtGui import QColor from PyQt4.QtGui import QPolygon from PyQt4.QtCore import SIGNAL as signal class Clock(QtGui.QWidget): ''' classdocs ''' def __init__(self): ''' Constructor ''' super(Clock, self).__init__() self.hourColor=QColor(127, 0, 127); self.minuteColor=QColor(0, 127, 127, 191) self.secondColor=QColor(127, 127,0,120) self.initUI() self.timer = QTimer() self.timer.timeout.connect(self.update) self.timer.start(30) self.show() def handChange(self): self.side = min(self.width(), self.height()) self.hand=(max(self.side/200,4), max(self.side/100,8), max(self.side/40,30)) self.hourHand=QPolygon([QPoint(self.hand[0],self.hand[1]),QPoint(-self.hand[0],self.hand[1]),QPoint(0,-self.hand[2])]) self.minuteHand=QPolygon([QPoint(self.hand[0],self.hand[1]),QPoint(-self.hand[0],self.hand[1]),QPoint(0,-self.hand[2]*2)]) self.secondHand=QPolygon([QPoint(self.hand[0],self.hand[1]),QPoint(-self.hand[0],self.hand[1]),QPoint(0,-self.hand[2]*3)]) def set_transparency(self, enabled): if enabled: self.setAutoFillBackground(False) else: self.setAttribute(Qt.WA_NoSystemBackground, False) #下面這種方式好像不行 # pal=QtGui.QPalette() # pal.setColor(QtGui.QPalette.Background, QColor(127, 127,10,120)) # self.setPalette(pal) self.setAttribute(Qt.WA_TranslucentBackground, enabled) self.repaint() def initUI(self): self.setGeometry(300, 300, 300, 200) self.setWindowTitle('Clock') self.handChange() self.rightButton=False # 下面兩個配合實現窗體透明和置頂 sizeGrip=QtGui.QSizeGrip(self) self.setWindowFlags(Qt.FramelessWindowHint|Qt.WindowStaysOnTopHint|Qt.SubWindow ) #self.setMouseTracking(True); self.trans=True self.set_transparency(True) quitAction = QtGui.QAction(QtGui.QIcon('quit.png'), '&Quit', self) self.connect(quitAction,signal("triggered()"),QtGui.qApp.quit) backAction = QtGui.QAction( '&Back', self) self.connect(backAction,signal("triggered()"),self.backClicked) self.popMenu= QtGui.QMenu() self.popMenu.addAction(quitAction) self.popMenu.addAction(backAction) def resizeEvent(self, e): self.handChange() def backClicked(self): if self.trans == True : self.trans = False self.set_transparency(False) else: self.trans = True self.set_transparency(True) def mouseReleaseEvent(self,e): if self.rightButton == True: self.rightButton=False self.popMenu.popup(e.globalPos()) def mouseMoveEvent(self, e): if e.buttons() & Qt.LeftButton: self.move(e.globalPos()-self.dragPos) e.accept() def mousePressEvent(self, e): if e.button() == Qt.LeftButton: self.dragPos=e.globalPos()-self.frameGeometry().topLeft() e.accept() if e.button() == Qt.RightButton and self.rightButton == False: self.rightButton=True def paintEvent(self, e): time = QTime.currentTime() qp = QPainter() qp.begin(self) qp.setRenderHint(QPainter.Antialiasing) # 開啟這個抗鋸齒,會很占cpu的! qp.translate(self.width() / 2, self.height() / 2) qp.scale(self.side / 200.0, self.side / 200.0) qp.setPen(QtCore.Qt.NoPen) qp.setBrush(self.hourColor) qp.save() qp.rotate(30.0 * ((time.hour() + time.minute()/ 60.0))) qp.drawConvexPolygon(self.hourHand) qp.restore() qp.setPen(self.hourColor) for i in range(12): qp.drawLine(88, 0, 96, 0) qp.rotate(30.0) qp.setPen(QtCore.Qt.NoPen) qp.setBrush(self.minuteColor) qp.save() qp.rotate(6.0 * ((time.minute() + (time.second()+time.msec()/1000.0) / 60.0))) qp.drawConvexPolygon(self.minuteHand) qp.restore() qp.setPen(self.minuteColor) for i in range(60): if (i % 5) is not 0: qp.drawLine(92, 0, 96, 0) qp.rotate(6.0) qp.setPen(QtCore.Qt.NoPen) qp.setBrush(self.secondColor) qp.save() qp.rotate(6.0*(time.second()+time.msec()/1000.0)) qp.drawConvexPolygon(self.secondHand) qp.restore() qp.end() if __name__ == '__main__': app = QtGui.QApplication(sys.argv) clock = Clock() sys.exit(app.exec_())
例三:
主要是模仿了qt自帶的clock例子,然后 通過 python的語法 進行實現,
同時添加了秒針 的實現
用到的工具 有qt 4.8;python 2.7 ;pyqt
import sys from PyQt4.QtCore import * from PyQt4.QtGui import * class clock (QWidget): def __init__(self): QWidget.__init__(self,windowTitle="python clock") timer = QTimer(self) self.connect(timer, SIGNAL("timeout()"),self,SLOT("update()")) timer.start(1000) self.resize(200,200) def paintEvent(self,e): hourColorHand = QPolygon([QPoint(7,8),QPoint(-7,8),QPoint(0,-30)]) minuteColorHand = QPolygon([QPoint(7,8),QPoint(-7,8),QPoint(0,-70)]) secondColorHand = QPolygon([QPoint(3,8),QPoint(-3,8),QPoint(0,-90)]) hourColor = QColor(127,0,127) minuteColor = QColor(0,127,127,191) secondColor = QColor(0,100,100,100) painter = QPainter(self); side = min(self.width(),self.height()) atime =QTime.currentTime() painter.setRenderHint(QPainter.Antialiasing) painter.translate(self.width()/2,self.height()/2) painter.scale(side/200,side/200) painter.setPen(Qt.NoPen) painter.setBrush(hourColor) painter.save() painter.rotate(30.0*(atime.hour() + atime.minute()/60.0)) painter.drawConvexPolygon(hourColorHand) painter.restore() painter.setPen(hourColor) for i in range(0,12): painter.drawLine(88,0,96,0) painter.rotate(30.0) painter.setPen(Qt.NoPen) painter.setBrush(minuteColor) painter.save() painter.rotate(6.0*(atime.minute()+atime.second()/60.0)) painter.drawConvexPolygon(minuteColorHand) painter.restore() painter.setPen(minuteColor) for i in range(0,60) : if (i%5)!=0 : painter.drawLine(92,0,96,0) painter.rotate(6.0) painter.setPen(Qt.NoPen) painter.setBrush(secondColor) painter.save() painter.rotate(6.0 * atime.second()) painter.drawConvexPolygon(secondColorHand) painter.restore() if __name__ == "__main__" : q = QApplication(sys.argv) s = clock() s.show() q.exec_()
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
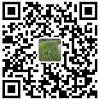
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
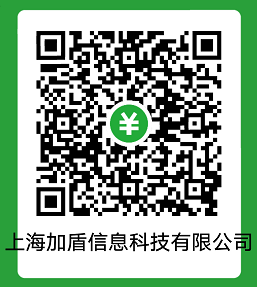