在項目中我們會經常遇到這種圓角效果,因為直角的看起來確實不那么雅觀,可能大家會想到用圖片實現,試想上中下要分別做三張圖片,這樣既會是自己的項目增大也會增加內存使用量,所以使用shape來實現不失為一種更好的實現方式。在這里先看一下shape的使用:
- <? xml version = "1.0" encoding = "utf-8" ?>
- < shape xmlns:android = "http://schemas.android.com/apk/res/android"
- android:shape = "rectangle"
- >
- <!--漸變-->
- < gradient
- android:startColor = "#B5E7B8"
- android:endColor = "#76D37B"
- android:angle = "270"
- />
- <!--描邊-->
- <!-- < stroke android:width = "1dip"
- android:color = "@color/blue"
- /> -- >
- <!--實心-->
- <!-- < solid android:color = "#FFeaeaea"
- /> -- >
- <!--圓角-->
- < corners
- android:bottomRightRadius = "4dip"
- android:bottomLeftRadius = "4dip"
- android:topLeftRadius = "4dip"
- android:topRightRadius = "4dip"
- />
- </ shape >
solid:實心,就是填充的意思
android:color指定填充的顏色
gradient:漸變
android:startColor和android:endColor分別為起始和結束顏色,ndroid:angle是漸變角度,必須為45的整數倍。
另外漸變默認的模式為android:type="linear",即線性漸變,可以指定漸變為徑向漸變,android:type="radial",徑向漸變需要指定半徑android:gradientRadius="50"。
stroke:描邊
android:width="2dp" 描邊的寬度,android:color 描邊的顏色。
我們還可以把描邊弄成虛線的形式,設置方式為:
android:dashWidth="5dp"
android:dashGap="3dp"
其中android:dashWidth表示'-'這樣一個橫線的寬度,android:dashGap表示之間隔開的距離。
corners:圓角
android:radius為角的弧度,值越大角越圓。
OK,下面開始自定義listview實現圓角的例子:
首先在drawable下定義只有一項的選擇器app_list_corner_round.xml:
- <? xml version = "1.0" encoding = "utf-8" ?>
- < shape xmlns:android = "http://schemas.android.com/apk/res/android"
- android:shape = "rectangle"
- >
- <!--漸變-->
- < gradient
- android:startColor = "#B5E7B8"
- android:endColor = "#76D37B"
- android:angle = "270"
- />
- <!--描邊-->
- <!-- < stroke android:width = "1dip"
- android:color = "@color/blue"
- /> -- >
- <!--實心-->
- <!-- < solid android:color = "#FFeaeaea"
- /> -- >
- <!--圓角-->
- < corners
- android:bottomRightRadius = "4dip"
- android:bottomLeftRadius = "4dip"
- android:topLeftRadius = "4dip"
- android:topRightRadius = "4dip"
- />
- </ shape >
- <? xml version = "1.0" encoding = "utf-8" ?>
- < shape xmlns:android = "http://schemas.android.com/apk/res/android"
- android:shape = "rectangle"
- >
- < gradient
- android:startColor = "#B5E7B8"
- android:endColor = "#76D37B"
- android:angle = "270"
- />
- <!-- < stroke android:width = "1dip"
- android:color = "@color/blue"
- /> -- >
- <!-- < solid android:color = "#FFeaeaea"
- /> -- >
- < corners
- android:topLeftRadius = "4dip"
- android:topRightRadius = "4dip"
- />
- </ shape >
如果是底部最后一項,則下面兩個角為圓角,app_list_corner_round_bottom.xml:
- <? xml version = "1.0" encoding = "utf-8" ?>
- < shape xmlns:android = "http://schemas.android.com/apk/res/android"
- android:shape = "rectangle"
- >
- < gradient
- android:startColor = "#B5E7B8"
- android:endColor = "#76D37B"
- android:angle = "270"
- />
- <!-- < stroke android:width = "1dip"
- android:color = "@color/blue"
- /> -- >
- <!-- < solid android:color = "#FFeaeaea"
- /> -- >
- < corners
- android:bottomRightRadius = "4dip"
- android:bottomLeftRadius = "4dip"
- />
- </ shape >
如果是中間項,則應該不需要圓角, app_list_corner_round_center.xml:
- <? xml version = "1.0" encoding = "utf-8" ?>
- < shape xmlns:android = "http://schemas.android.com/apk/res/android"
- android:shape = "rectangle"
- >
- < gradient
- android:startColor = "#B5E7B8"
- android:endColor = "#76D37B"
- android:angle = "270"
- />
- <!-- < stroke android:width = "1dip"
- android:color = "@color/blue"
- /> -- >
- <!-- < solid android:color = "#FFeaeaea"
- /> -- >
- <!-- < corners
- android:bottomRightRadius = "4dip"
- android:bottomLeftRadius = "4dip"
- /> -- >
- </ shape >
listview的背景圖片大家可以使用stroke描述,這里我使用了一張9PNG的圖片,因為9PNG圖片拉伸不失真。
定義好了圓角的shape接下來是自定義listview的實現:
- packagecn.com.karl.view;
- importcn.com.karl.test.R;
- importandroid.content.Context;
- importandroid.util.AttributeSet;
- importandroid.view.MotionEvent;
- importandroid.widget.AdapterView;
- importandroid.widget.ListView;
- /**
- *圓角ListView
- */
- publicclassCornerListViewextendsListView{
- publicCornerListView(Contextcontext){
- super(context);
- }
- publicCornerListView(Contextcontext,AttributeSetattrs,intdefStyle){
- super(context,attrs,defStyle);
- }
- publicCornerListView(Contextcontext,AttributeSetattrs){
- super(context,attrs);
- }
- @Override
- publicbooleanonInterceptTouchEvent(MotionEventev){
- switch(ev.getAction()){
- caseMotionEvent.ACTION_DOWN:
- int x =(int)ev.getX();
- int y =(int)ev.getY();
- int itemnum = pointToPosition (x,y);
- if( itemnum ==AdapterView.INVALID_POSITION)
- break;
- else{
- if( itemnum ==0){
- if( itemnum ==(getAdapter().getCount()-1)){
- //只有一項
- setSelector(R.drawable.app_list_corner_round);
- }else{
- //第一項
- setSelector(R.drawable.app_list_corner_round_top);
- }
- }elseif( itemnum ==(getAdapter().getCount()-1))
- //最后一項
- setSelector(R.drawable.app_list_corner_round_bottom);
- else{
- //中間項
- setSelector(R.drawable.app_list_corner_round_center);
- }
- }
- break;
- caseMotionEvent.ACTION_UP:
- break;
- }
- returnsuper.onInterceptTouchEvent(ev);
- }
- }
下面看一下列表布局文件setting。xml:
- <? xml version = "1.0" encoding = "utf-8" ?>
- < LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
- android:layout_width = "fill_parent"
- android:layout_height = "fill_parent"
- android:orientation = "vertical" >
- < include layout = "@layout/head" />
- < cn.com.karl.view.CornerListView
- android:id = "@+id/setting_list"
- android:layout_width = "fill_parent"
- android:layout_height = "wrap_content"
- android:layout_margin = "10dip"
- android:background = "@drawable/corner_list_bg"
- android:cacheColorHint = "#00000000" />
- </ LinearLayout >
自定義Listview對應的item文件 main_tab_setting_list_item.xml
- <? xml version = "1.0" encoding = "utf-8" ?>
- < LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android"
- android:layout_width = "fill_parent"
- android:layout_height = "wrap_content" >
- < RelativeLayout
- android:layout_width = "fill_parent"
- android:layout_height = "50dp"
- android:gravity = "center_horizontal" >
- < TextView
- android:id = "@+id/tv_system_title"
- android:layout_width = "wrap_content"
- android:layout_height = "wrap_content"
- android:layout_alignParentLeft = "true"
- android:layout_centerVertical = "true"
- android:layout_marginLeft = "10dp"
- android:text = "分享"
- android:textColor = "@color/black" />
- < ImageView
- android:id = "@+id/iv_system_right"
- android:layout_width = "wrap_content"
- android:layout_height = "wrap_content"
- android:layout_alignParentRight = "true"
- android:layout_centerVertical = "true"
- android:layout_marginRight = "10dp"
- android:src = "@drawable/arrow1" />
- </ RelativeLayout >
- </ LinearLayout >
最后是在activity中填充適配器:
- packagecn.com.karl.test;
- importjava.util.ArrayList;
- importjava.util.HashMap;
- importjava.util.List;
- importjava.util.Map;
- importcn.com.karl.view.CornerListView;
- importandroid.os.Bundle;
- importandroid.view.View;
- importandroid.widget.SimpleAdapter;
- publicclassSettingActivityextendsBaseActivity{
- privateCornerListView cornerListView = null ;
- privateList < Map < String ,String > > listData = null ;
- privateSimpleAdapter adapter = null ;
- @Override
- protectedvoidonCreate(BundlesavedInstanceState){
- //TODOAuto-generatedmethodstub
- super.onCreate(savedInstanceState);
- setContentView(R.layout.setting);
- cornerListView =(CornerListView)findViewById(R.id.setting_list);
- setListData();
- adapter = new SimpleAdapter(getApplicationContext(),listData,R.layout.main_tab_setting_list_item,
- newString[]{"text"},newint[]{R.id.tv_system_title});
- cornerListView.setAdapter(adapter);
- initHead();
- btn_leftTop.setVisibility(View.INVISIBLE);
- tv_head.setText("設置");
- }
- /**
- *設置列表數據
- */
- privatevoidsetListData(){
- listData = new ArrayList < Map < String ,String > > ();
- Map < String ,String > map = new HashMap < String ,String > ();
- map.put("text","分享");
- listData.add(map);
- map = new HashMap < String ,String > ();
- map.put("text","檢查新版本");
- listData.add(map);
- map = new HashMap < String ,String > ();
- map.put("text","反饋意見");
- listData.add(map);
- map = new HashMap < String ,String > ();
- map.put("text","關于我們");
- listData.add(map);
- map = new HashMap < String ,String > ();
- map.put("text","支持我們,請點擊這里的廣告");
- listData.add(map);
- }
- }
這樣就完成了,雖然過程較繁雜,但是當做一個好的模板以后使用會方便很多,最后看一下實現效果和我們用圖片實現的一樣嗎
更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
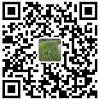
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
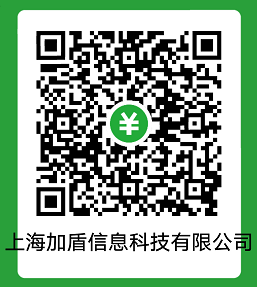