?
- package ?com.esri.adf.web; ??
- ??
- import ?java.rmi.RemoteException; ??
- import ?java.util.Collection; ??
- import ?java.util.List; ??
- import ?java.util.Map; ??
- import ?com.esri.adf.web.ags.data.AGSLocalMapResource; ??
- import ?com.esri.adf.web.ags.data.AGSMapResource; ??
- import ?com.esri.adf.web.data.WebContext; ??
- import ?com.esri.adf.web.data.geometry.WebExtent; ??
- import ?com.esri.adf.web.data.query.IdentifyCriteria; ??
- import ?com.esri.adf.web.data.query.QueryResult; ??
- import ?com.esri.adf.web.data.query.TextCriteria; ??
- import ?com.esri.adf.web.data.query.WebQuery; ??
- import ?com.esri.adf.web.faces.event.MapEvent; ??
- import ?com.esri.adf.web.faces.event.MapToolAction; ??
- import ?com.esri.arcgis.carto.IFeatureLayer; ??
- import ?com.esri.arcgis.carto.IFeatureSelection; ??
- import ?com.esri.arcgis.carto.IMap; ??
- import ?com.esri.arcgis.carto.IMapServer; ??
- import ?com.esri.arcgis.carto.IMapServerObjects; ??
- import ?com.esri.arcgis.carto.esriSelectionResultEnum; ??
- import ?com.esri.arcgis.geodatabase.Field; ??
- import ?com.esri.arcgis.geodatabase.ICursor; ??
- import ?com.esri.arcgis.geodatabase.IField; ??
- import ?com.esri.arcgis.geodatabase.IFields; ??
- import ?com.esri.arcgis.geodatabase.IRecordSet; ??
- import ?com.esri.arcgis.geodatabase.IRow; ??
- import ?com.esri.arcgis.geodatabase.ISelectionSet; ??
- import ?com.esri.arcgis.geodatabase.SpatialFilter; ??
- import ?com.esri.arcgis.geodatabase.esriSpatialRelEnum; ??
- import ?com.esri.arcgis.geometry.Envelope; ??
- import ?com.esri.arcgis.server.IServerContext; ??
- import ?com.esri.arcgisws.EnvelopeN; ??
- import ?com.esri.arcgisws.EsriSearchOrder; ??
- import ?com.esri.arcgisws.EsriSpatialRelEnum; ??
- import ?com.esri.arcgisws.MapServerPort; ??
- import ?com.esri.arcgisws.Record; ??
- import ?com.esri.arcgisws.RecordSet; ??
- ??
- public ? class ?ADFQuery? implements ?MapToolAction?{ ??
- ???? ??
- ???? private ? static ? final ? long ?serialVersionUID?=?713600076584099585L; ??
- ????WebContext?context?=? null ; ??
- ???? int ?c?=? 0 ; ??
- ??
- ???? /* ?
- ?????*?WebQuery ?
- ?????*/ ??
- ???? private ? void ?test1(WebExtent?extent)?{ ??
- ????????AGSMapResource?res?=?(AGSMapResource)?context.getResources().get( "ags0" ); ??
- ????????IdentifyCriteria?ic?=? new ?IdentifyCriteria(extent); ??
- ????????WebQuery?query?=?(WebQuery)?context.getAttribute( "query" ); ??
- ????????List?layer?=?context.getWebQuery().getQueryLayers(); ??
- ????????List?results?=?query.query(ic,?layer); ??
- ???????? for ?( int ?i?=? 0 ;?i?<?results.size();?i++)?{ ??
- ????????????QueryResult?result?=?(QueryResult)?results.get(i); ??
- ??
- ????????????result.highlight(); ??
- ????????????Map?map?=?result.getDetails(); ??
- ????????????map.size(); ??
- ????????????Collection?col?=?map.values(); ??
- ????????????Object[]?obj?=?col.toArray(); ??
- ???????????? for ?( int ?j?=? 0 ;?j?<?obj.length;?j++)?{ ??
- ????????????????System.out.println(obj[j]); ??
- ????????????} ??
- ????????} ??
- ????} ??
- ??
- ???? /* ?
- ?????*?arcgisws.SpatialFilter ?
- ?????*/ ??
- ???? private ? void ?test2(WebExtent?extent)?{ ??
- ????????AGSMapResource?res?=?(AGSMapResource)?context.getResources().get( "ags0" ); ??
- ????????MapServerPort?mapServer?=?res.getMapServer(); ??
- ????????EnvelopeN?env?=? new ?EnvelopeN(extent.getMinX(),?extent.getMinY(), ??
- ????????????????extent.getMaxX(),?extent.getMaxY(),? null ,? null ,? null ,? null , ??
- ???????????????? null ); ??
- ????????com.esri.arcgisws.SpatialFilter?filter?=? new ?com.esri.arcgisws.SpatialFilter(); ??
- ????????filter.setSpatialRel(EsriSpatialRelEnum.esriSpatialRelIntersects); ??
- ????????filter.setWhereClause( "" ); ??
- ????????filter.setSearchOrder(EsriSearchOrder.esriSearchOrderSpatial); ??
- ????????filter.setSpatialRelDescription( "" ); ??
- ????????filter.setGeometryFieldName( "" ); ??
- ????????filter.setFilterGeometry(env); ??
- ???????? int ?layerId?=? 1 ; ??
- ???????? try ?{ ??
- ????????????RecordSet?rs?=?mapServer.queryFeatureData(mapServer.getDefaultMapName(),?layerId,?filter); ??
- ????????????Record[]?rd?=?rs.getRecords(); ??
- ???????????? for ?( int ?i?=? 0 ;?i?<?rd.length;?i++)?{ ??
- ????????????????Object[]?obj?=?rd[i].getValues(); ??
- ???????????????? for ?( int ?j?=? 0 ;?j?<?obj.length;?j++)?{ ??
- ????????????????????String?s?=?obj[j].toString(); ??
- ????????????????} ??
- ????????????} ??
- ????????}? catch ?(RemoteException?e)?{ ??
- ????????????e.printStackTrace(); ??
- ????????} ??
- ????} ??
- ??
- ???? /* ?
- ?????*?geodatabase.SpatialFilter ?
- ?????*/ ??
- ???? private ? void ?test3(WebExtent?extent){ ??
- ???????? try ?{ ??
- ????????????AGSLocalMapResource?res?=?(AGSLocalMapResource)?context.getResources().get( "ags0" ); ??
- ????????????IMapServer?mapServer?=?res.getLocalMapServer(); ??
- ????????????IServerContext?sc?=?res.getServerContext(); ??
- ????????????Envelope?env?=?(Envelope)?sc.createObject(Envelope.getClsid()); ??
- ????????????env.putCoords(extent.getMinX(),?extent.getMinY(),?extent.getMaxX(), ??
- ????????????????????extent.getMaxY()); ??
- ????????????com.esri.arcgis.geodatabase.SpatialFilter?filter?=?(SpatialFilter)?sc ??
- ????????????????????.createObject(com.esri.arcgis.geodatabase.SpatialFilter ??
- ????????????????????????????.getClsid()); ??
- ????????????filter.setGeometryByRef(env); ??
- ????????????filter.setSpatialRel(esriSpatialRelEnum.esriSpatialRelIndexIntersects); ??
- ????????????System.out.println( "---1---?" ); ??
- ???????????? int ?layerId?=? 1 ; ??
- ????????????IRecordSet?rs?=?mapServer.queryFeatureData(mapServer.getDefaultMapName(),?layerId,?filter); ??
- ????????????IFields?fds?=?rs.getFields(); ??
- ????????????System.out.println( "---cnt2---?" ?+?fds.getFieldCount()); ??
- ???????????? for ?( int ?i?=? 0 ;?i?<?fds.getFieldCount();?i++)?{ ??
- ????????????????IField?fd?=?(Field)?fds.getField(i); ??
- ????????????????System.out.println(fd.getName()); ??
- ????????????} ??
- ????????????ICursor?cursor?=?rs.getCursor( true ); ??
- ????????????IRow?row?=?cursor.nextRow(); ??
- ???????????? while ?(row?!=? null )?{ ??
- ????????????????System.out.println( "------?" ?+?row.getValue( 4 )); ??
- ????????????????row?=?cursor.nextRow(); ??
- ????????????} ??
- ????????}? catch ?(Exception?e)?{ ??
- ????????????e.printStackTrace(); ??
- ????????} ??
- ????} ??
- ??
- ???? /* ?
- ?????*?IFeatureSelection ?
- ?????*/ ??
- ???? private ? void ?test4(WebExtent?extent){ ??
- ????????AGSLocalMapResource?res?=?(AGSLocalMapResource)?context.getResources().get( "ags0" ); ??
- ????????IServerContext?sc?=?res.getServerContext(); ??
- ????????IMapServerObjects?mso?=?(IMapServerObjects)?res.getLocalMapServer(); ??
- ????????IMap?map; ??
- ???????? try ?{ ??
- ????????????map?=?mso.getMap(res.getLocalMapServer().getDefaultMapName()); ??
- ????????????IFeatureLayer?fl?=?(IFeatureLayer)?map.getLayer( 1 ); ??
- ????????????Envelope?env?=?(Envelope)?sc.createObject(Envelope.getClsid()); ??
- ????????????env.putCoords(extent.getMinX(),?extent.getMinY(),?extent.getMaxX(), ??
- ????????????????????extent.getMaxY()); ??
- ????????????com.esri.arcgis.geodatabase.SpatialFilter?filter?=?(SpatialFilter)?sc ??
- ????????????????????.createObject(com.esri.arcgis.geodatabase.SpatialFilter ??
- ????????????????????????????.getClsid()); ??
- ????????????filter.setGeometryByRef(env); ??
- ????????????filter.setSpatialRel(esriSpatialRelEnum.esriSpatialRelIndexIntersects); ??
- ??
- ????????????IFeatureSelection?fs?=?(IFeatureSelection)?fl; ??
- ????????????fs.selectFeatures(filter,esriSelectionResultEnum.esriSelectionResultNew,? false ); ??
- ????????????ISelectionSet?ss?=?fs.getSelectionSet(); ??
- ????????}? catch ?(Exception?e)?{ ??
- ????????????e.printStackTrace(); ??
- ????????} ??
- ????} ??
- ??
- ???? /* ?
- ?????*?TextCriteria ?
- ?????*/ ??
- ???? private ? void ?test5()?{ ??
- ????????AGSMapResource?res?=?(AGSMapResource)?context.getResources().get( "ags0" ); ??
- ????????TextCriteria?tc?=? new ?TextCriteria(); ??
- ????????tc.setSearchText( "北京市" ); ??
- ????????WebQuery?query?=?(WebQuery)?context.getAttribute( "query" ); ??
- ????????List?layer?=?context.getWebQuery().getQueryLayers(); ??
- ????????List?results?=?query.query(tc,?layer); ??
- ???????? for ?( int ?i?=? 0 ;?i?<?results.size();?i++)?{ ??
- ????????????QueryResult?result?=?(QueryResult)?results.get(i); ??
- ????????????result.highlight(); ??
- ????????????Map?map?=?result.getDetails(); ??
- ????????????map.size(); ??
- ????????????Collection?col?=?map.values(); ??
- ????????????Object[]?obj?=?col.toArray(); ??
- ???????????? for ?( int ?j?=? 0 ;?j?<?obj.length;?j++)?{ ??
- ????????????????System.out.println(obj[j]); ??
- ????????????} ??
- ????????} ??
- ????} ??
- ??
- ???? public ? void ?execute(MapEvent?arg0)? throws ?Exception?{ ??
- ????????context?=?arg0.getWebContext(); ??
- ????????WebExtent?ex?=?(WebExtent)?arg0.getWebGeometry(); ??
- ????????ex?=?(WebExtent)?ex.toMapGeometry(arg0.getWebContext().getWebMap()); ??
- ????????test5(); ??
- ????????System.out.println( "---ok---" ); ??
- ????} ??
- ???? ??
- }??
package com.esri.adf.web; import java.rmi.RemoteException; import java.util.Collection; import java.util.List; import java.util.Map; import com.esri.adf.web.ags.data.AGSLocalMapResource; import com.esri.adf.web.ags.data.AGSMapResource; import com.esri.adf.web.data.WebContext; import com.esri.adf.web.data.geometry.WebExtent; import com.esri.adf.web.data.query.IdentifyCriteria; import com.esri.adf.web.data.query.QueryResult; import com.esri.adf.web.data.query.TextCriteria; import com.esri.adf.web.data.query.WebQuery; import com.esri.adf.web.faces.event.MapEvent; import com.esri.adf.web.faces.event.MapToolAction; import com.esri.arcgis.carto.IFeatureLayer; import com.esri.arcgis.carto.IFeatureSelection; import com.esri.arcgis.carto.IMap; import com.esri.arcgis.carto.IMapServer; import com.esri.arcgis.carto.IMapServerObjects; import com.esri.arcgis.carto.esriSelectionResultEnum; import com.esri.arcgis.geodatabase.Field; import com.esri.arcgis.geodatabase.ICursor; import com.esri.arcgis.geodatabase.IField; import com.esri.arcgis.geodatabase.IFields; import com.esri.arcgis.geodatabase.IRecordSet; import com.esri.arcgis.geodatabase.IRow; import com.esri.arcgis.geodatabase.ISelectionSet; import com.esri.arcgis.geodatabase.SpatialFilter; import com.esri.arcgis.geodatabase.esriSpatialRelEnum; import com.esri.arcgis.geometry.Envelope; import com.esri.arcgis.server.IServerContext; import com.esri.arcgisws.EnvelopeN; import com.esri.arcgisws.EsriSearchOrder; import com.esri.arcgisws.EsriSpatialRelEnum; import com.esri.arcgisws.MapServerPort; import com.esri.arcgisws.Record; import com.esri.arcgisws.RecordSet; public class ADFQuery implements MapToolAction { private static final long serialVersionUID = 713600076584099585L; WebContext context = null; int c = 0; /* * WebQuery */ private void test1(WebExtent extent) { AGSMapResource res = (AGSMapResource) context.getResources().get("ags0"); IdentifyCriteria ic = new IdentifyCriteria(extent); WebQuery query = (WebQuery) context.getAttribute("query"); List layer = context.getWebQuery().getQueryLayers(); List results = query.query(ic, layer); for (int i = 0; i < results.size(); i++) { QueryResult result = (QueryResult) results.get(i); result.highlight(); Map map = result.getDetails(); map.size(); Collection col = map.values(); Object[] obj = col.toArray(); for (int j = 0; j < obj.length; j++) { System.out.println(obj[j]); } } } /* * arcgisws.SpatialFilter */ private void test2(WebExtent extent) { AGSMapResource res = (AGSMapResource) context.getResources().get("ags0"); MapServerPort mapServer = res.getMapServer(); EnvelopeN env = new EnvelopeN(extent.getMinX(), extent.getMinY(), extent.getMaxX(), extent.getMaxY(), null, null, null, null, null); com.esri.arcgisws.SpatialFilter filter = new com.esri.arcgisws.SpatialFilter(); filter.setSpatialRel(EsriSpatialRelEnum.esriSpatialRelIntersects); filter.setWhereClause(""); filter.setSearchOrder(EsriSearchOrder.esriSearchOrderSpatial); filter.setSpatialRelDescription(""); filter.setGeometryFieldName(""); filter.setFilterGeometry(env); int layerId = 1; try { RecordSet rs = mapServer.queryFeatureData(mapServer.getDefaultMapName(), layerId, filter); Record[] rd = rs.getRecords(); for (int i = 0; i < rd.length; i++) { Object[] obj = rd[i].getValues(); for (int j = 0; j < obj.length; j++) { String s = obj[j].toString(); } } } catch (RemoteException e) { e.printStackTrace(); } } /* * geodatabase.SpatialFilter */ private void test3(WebExtent extent){ try { AGSLocalMapResource res = (AGSLocalMapResource) context.getResources().get("ags0"); IMapServer mapServer = res.getLocalMapServer(); IServerContext sc = res.getServerContext(); Envelope env = (Envelope) sc.createObject(Envelope.getClsid()); env.putCoords(extent.getMinX(), extent.getMinY(), extent.getMaxX(), extent.getMaxY()); com.esri.arcgis.geodatabase.SpatialFilter filter = (SpatialFilter) sc .createObject(com.esri.arcgis.geodatabase.SpatialFilter .getClsid()); filter.setGeometryByRef(env); filter.setSpatialRel(esriSpatialRelEnum.esriSpatialRelIndexIntersects); System.out.println("---1--- "); int layerId = 1; IRecordSet rs = mapServer.queryFeatureData(mapServer.getDefaultMapName(), layerId, filter); IFields fds = rs.getFields(); System.out.println("---cnt2--- " + fds.getFieldCount()); for (int i = 0; i < fds.getFieldCount(); i++) { IField fd = (Field) fds.getField(i); System.out.println(fd.getName()); } ICursor cursor = rs.getCursor(true); IRow row = cursor.nextRow(); while (row != null) { System.out.println("------ " + row.getValue(4)); row = cursor.nextRow(); } } catch (Exception e) { e.printStackTrace(); } } /* * IFeatureSelection */ private void test4(WebExtent extent){ AGSLocalMapResource res = (AGSLocalMapResource) context.getResources().get("ags0"); IServerContext sc = res.getServerContext(); IMapServerObjects mso = (IMapServerObjects) res.getLocalMapServer(); IMap map; try { map = mso.getMap(res.getLocalMapServer().getDefaultMapName()); IFeatureLayer fl = (IFeatureLayer) map.getLayer(1); Envelope env = (Envelope) sc.createObject(Envelope.getClsid()); env.putCoords(extent.getMinX(), extent.getMinY(), extent.getMaxX(), extent.getMaxY()); com.esri.arcgis.geodatabase.SpatialFilter filter = (SpatialFilter) sc .createObject(com.esri.arcgis.geodatabase.SpatialFilter .getClsid()); filter.setGeometryByRef(env); filter.setSpatialRel(esriSpatialRelEnum.esriSpatialRelIndexIntersects); IFeatureSelection fs = (IFeatureSelection) fl; fs.selectFeatures(filter,esriSelectionResultEnum.esriSelectionResultNew, false); ISelectionSet ss = fs.getSelectionSet(); } catch (Exception e) { e.printStackTrace(); } } /* * TextCriteria */ private void test5() { AGSMapResource res = (AGSMapResource) context.getResources().get("ags0"); TextCriteria tc = new TextCriteria(); tc.setSearchText("北京市"); WebQuery query = (WebQuery) context.getAttribute("query"); List layer = context.getWebQuery().getQueryLayers(); List results = query.query(tc, layer); for (int i = 0; i < results.size(); i++) { QueryResult result = (QueryResult) results.get(i); result.highlight(); Map map = result.getDetails(); map.size(); Collection col = map.values(); Object[] obj = col.toArray(); for (int j = 0; j < obj.length; j++) { System.out.println(obj[j]); } } } public void execute(MapEvent arg0) throws Exception { context = arg0.getWebContext(); WebExtent ex = (WebExtent) arg0.getWebGeometry(); ex = (WebExtent) ex.toMapGeometry(arg0.getWebContext().getWebMap()); test5(); System.out.println("---ok---"); } }
內(nèi)容摘要
使用ArcGIS Server java ADF開發(fā),如果需要空間關(guān)系查詢,可以有兩種辦法,
一種是通過com.esri.arcgisws.SpatialFilter這個包來實(shí)現(xiàn),是一種SOAP的方式,
另外一種是使用傳統(tǒng)的AO的方式實(shí)現(xiàn):com.esri.arcgis.geodatabase.SpatialFilter注意兩個包的命名一樣,很容易混淆。
過程描述
第一種方式的代碼如下:
?
- SpatialFilter?filter?=? new ?SpatialFilter();? ??
- ??String?mapName=functionality.getMapDescription().getName(); ??
- ?? ??
- ?? this .getWebContext().getWebGraphics().clearGraphics(); ??
- ??WebPoint?wPoint=(WebPoint)event.getWebGeometry().toMapGeometry(event.getWebContext().getWebMap()); ??
- ?? ??
- ?? ??
- ???Geometry?geom?=?AGSUtil.toAGSGeometry(wPoint); ??
- ???com.esri.arcgisws.PointN?pontN?=?(com.esri.arcgisws.PointN)geom; ??
- ???Point?aoPont?=?(Point)AGSUtil.createArcObjectFromStub(pontN,?serverContext); ??
- ??? ??
- ???filter.setFilterGeometry(geom);? ??
- ???filter.setSpatialRel(com.esri.arcgisws.EsriSpatialRelEnum.esriSpatialRelRelation);? ??
- ???filter.setWhereClause( "" );? ??
- ???filter.setSearchOrder(com.esri.arcgisws.EsriSearchOrder.esriSearchOrderSpatial);? ??
- ???filter.setGeometryFieldName( "" );? ??
- ???filter.setSpatialReferenceFieldName( "" );? ??
- ???filter.setSpatialRelDescription(spatialType);? ??
- ??? ??
- ???MapLayerInfo?layerInfos[]?=?functionality.getLayerInfos();? ??
- ??? for ( int ?layerIndex= 0 ;layerIndex<layerInfos.length;layerIndex++){ ??
- ????MapLayerInfo?mapLayerInfo?=?(MapLayerInfo)?layerInfos[layerIndex];? ??
- ????Field[]?fields=mapLayerInfo.getFields().getFieldArray(); ??
- ????RecordSet?recordSet?=?mapServer.queryFeatureData(mapName,?layerIndex,?filter);? ??
- ????... ??
- ????}??
SpatialFilter filter = new SpatialFilter(); String mapName=functionality.getMapDescription().getName(); this.getWebContext().getWebGraphics().clearGraphics(); WebPoint wPoint=(WebPoint)event.getWebGeometry().toMapGeometry(event.getWebContext().getWebMap()); Geometry geom = AGSUtil.toAGSGeometry(wPoint); com.esri.arcgisws.PointN pontN = (com.esri.arcgisws.PointN)geom; Point aoPont = (Point)AGSUtil.createArcObjectFromStub(pontN, serverContext); filter.setFilterGeometry(geom); filter.setSpatialRel(com.esri.arcgisws.EsriSpatialRelEnum.esriSpatialRelRelation); filter.setWhereClause(""); filter.setSearchOrder(com.esri.arcgisws.EsriSearchOrder.esriSearchOrderSpatial); filter.setGeometryFieldName(""); filter.setSpatialReferenceFieldName(""); filter.setSpatialRelDescription(spatialType); MapLayerInfo layerInfos[] = functionality.getLayerInfos(); for(int layerIndex=0;layerIndex<layerInfos.length;layerIndex++){ MapLayerInfo mapLayerInfo = (MapLayerInfo) layerInfos[layerIndex]; Field[] fields=mapLayerInfo.getFields().getFieldArray(); RecordSet recordSet = mapServer.queryFeatureData(mapName, layerIndex, filter); ... }
第二種方式代碼如下:
?
- SpatialFilter?spatialFilter=?(SpatialFilter)serverContext.createObject(SpatialFilter.getClsid()); ??
- ?? ??
- ??WebPolygon?wPgon=(WebPolygon)event.getWebGeometry().toMapGeometry(event.getWebContext().getWebMap()); ??
- ??GraphicElement?ge?=? new ?GraphicElement(); ??
- ??ge.setGeometry(wPgon); ??
- ???ge.setSymbol( this .getWebContext().getWebQuery().getPolygonGraphicSymbol()); ??
- ??? this .getWebContext().getWebGraphics().addGraphics(ge); ??
- ?? ??
- ???Geometry?geom?=?AGSUtil.toAGSGeometry(wPgon); ??
- ???com.esri.arcgisws.PolygonN?pgonN?=?(com.esri.arcgisws.PolygonN)geom; ??
- ???Polygon?aoPgon?=?(Polygon)AGSUtil.createArcObjectFromStub(pgonN,?serverContext); ??
- ??? ??
- ??? for ( int ?layerIndex= 0 ;layerIndex<pMap.getLayerCount();layerIndex++){ ??
- ????ILayer?layer=pMap.getLayer(layerIndex); ??
- ????FeatureLayer?featureLayer=(FeatureLayer)layer; ??
- ????IFeatureClass?featureClass=featureLayer.getFeatureClass(); ??
- ????spatialFilter.setGeometryByRef(aoPgon); ??
- ????spatialFilter.setSpatialRel(esriSpatialRelEnum.esriSpatialRelWithin); ??
- ????spatialFilter.setGeometryField(featureClass.getShapeFieldName()); ??
- ????IFeatureCursor?featureCursor?=?featureClass.search(spatialFilter,? true ); ??
- ????IFeature?feature?=?featureCursor.nextFeature(); ??
- ????... ??
- ????}??
SpatialFilter spatialFilter= (SpatialFilter)serverContext.createObject(SpatialFilter.getClsid()); WebPolygon wPgon=(WebPolygon)event.getWebGeometry().toMapGeometry(event.getWebContext().getWebMap()); GraphicElement ge = new GraphicElement(); ge.setGeometry(wPgon); ge.setSymbol(this.getWebContext().getWebQuery().getPolygonGraphicSymbol()); this.getWebContext().getWebGraphics().addGraphics(ge); Geometry geom = AGSUtil.toAGSGeometry(wPgon); com.esri.arcgisws.PolygonN pgonN = (com.esri.arcgisws.PolygonN)geom; Polygon aoPgon = (Polygon)AGSUtil.createArcObjectFromStub(pgonN, serverContext); for(int layerIndex=0;layerIndex<pMap.getLayerCount();layerIndex++){ ILayer layer=pMap.getLayer(layerIndex); FeatureLayer featureLayer=(FeatureLayer)layer; IFeatureClass featureClass=featureLayer.getFeatureClass(); spatialFilter.setGeometryByRef(aoPgon); spatialFilter.setSpatialRel(esriSpatialRelEnum.esriSpatialRelWithin); spatialFilter.setGeometryField(featureClass.getShapeFieldName()); IFeatureCursor featureCursor = featureClass.search(spatialFilter, true); IFeature feature = featureCursor.nextFeature(); ... }
在一般的情況下,建議使用第一種方式空間查詢


更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
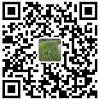
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點(diǎn)擊下面給點(diǎn)支持吧,站長非常感激您!手機(jī)微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點(diǎn)擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
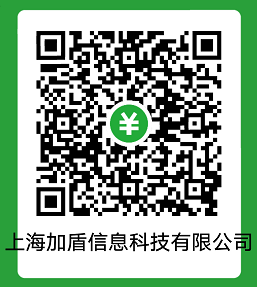