本節講述加密算法和加密算法的使用研究
如果有疑問請看源代碼:
輕松一刻哦
o(∩_∩)o...哈哈
徘徊在牛A和牛B之間的人
o(∩_∩)o...哈哈
package cn.com.huawei.opensource.common.codecs;
import java.util.logging.Logger;
import org.apache.commons.codec.DecoderException;
import org.apache.commons.codec.binary.Base64;
import org.apache.commons.codec.binary.Hex;
import org.apache.commons.codec.language.Metaphone;
import org.apache.commons.codec.language.RefinedSoundex;
import org.apache.commons.codec.language.Soundex;
import org.apache.commons.codec.digest.DigestUtils;
/**
*Commons-codec組件設置各種編碼是算法的信息
* @author bailonggang
* 2009-2-8
* 下午03:58:14
*/
public class CodeCUtil {
?
private static Logger logger=Logger.getLogger(CodeCUtil.class.getName());??
/**
? * MD5加密的過程的應用
? * @param crbyte
? * @return
? */
public static String encodeMD5(String crbyte)
{
byte[] bytes=crbyte.getBytes();
return DigestUtils.md5Hex(bytes);
}
/**
? * MD5加密的過程的應用
? * @param crbyte
? * @return
? */
public static String encodeSHA1(String crbyte)
{
byte[] bytes=crbyte.getBytes();
return DigestUtils.shaHex(bytes);
}
/**
? * 字符串的解碼過程
? * @param enbytes
? * @return
? */
?? public? static Object? decodeBase64(String enbytes)
?? {
?? Base64 base64 = new Base64();
?????? Object obj=null;
try {
obj = base64.decode(enbytes);
} catch (DecoderException e) {
????? logger.info("base64 decode ["+enbytes+"] error:"+e.getMessage());
}
??????? return obj;
?? }
?? /**
??? * base64編碼的過程的信息
??? * @param crbty
??? * @return
??? */
?? public? static String encodeBase64(String crbty)
?? {
?????? Base64 base64 = new Base64();
?????? byte[] enbytes =base64.encode(crbty.getBytes());
?????? return new String(enbytes);
?? }
?? /**
??? * 十六進制加密的過程
??? * @param enbyte
??? * @return
??? * @throws DecoderException
??? */
?? public static String encodeHex(String enbyte) throws DecoderException
?? {
?????? char[] enbytes = Hex.encodeHex(enbyte.getBytes());
?????????? return new String(enbytes);
?? }
?? /**
??? * 十六進制解密的過程
??? * @param enbytes
??? * @return
??? * @throws DecoderException
??? */
?? public static String decodeHex(String enbytes) throws DecoderException
?? {
?????? byte[] bytes=Hex.decodeHex(enbytes.toCharArray());
?????????? return new String(bytes);
?? }
?? /**
??? * Metaphone 建立出相同的key給發音相似的單字, 比 Soundex 還要準確, 但是 Metaphone
??? * 沒有固定長度, Soundex 則是固定第一個英文字加上3個數字. 這通常是用在類似音比對,
??? * 也可以用在 MP3 的軟件開發.
??? *
??? */
?? public static void languageEncoding()
?? {
?? Metaphone metaphone = new Metaphone();
?????????? RefinedSoundex refinedSoundex = new RefinedSoundex();
?????????? Soundex soundex = new Soundex();
?????????? for (int i = 0; i < 2; i++) {
?????????????? String str = (i == 0) ? "resume" : "resin";
?????????????? String mString = null;
?????????????? String rString = null;
?????????????? String sString = null;
?????????????? try {
?????????????????? mString = metaphone.encode(str);
?????????????????? rString = refinedSoundex.encode(str);
?????????????????? sString = soundex.encode(str);
?????????????? } catch (Exception ex){
?????????????????? ;
?????????????? }
?????????????? System.out.println("Original:" + str);
?????????????? System.out.println("Metaphone:" + mString);
?????????????? System.out.println("RefinedSoundex:" + rString);
?????????????? System.out.println("Soundex:" + sString + "\n");
?????????? }
?? }
}
如果有疑問請看源代碼:
輕松一刻哦
o(∩_∩)o...哈哈
徘徊在牛A和牛B之間的人
o(∩_∩)o...哈哈
package cn.com.huawei.opensource.common.codecs;
import java.util.logging.Logger;
import org.apache.commons.codec.DecoderException;
import org.apache.commons.codec.binary.Base64;
import org.apache.commons.codec.binary.Hex;
import org.apache.commons.codec.language.Metaphone;
import org.apache.commons.codec.language.RefinedSoundex;
import org.apache.commons.codec.language.Soundex;
import org.apache.commons.codec.digest.DigestUtils;
/**
*Commons-codec組件設置各種編碼是算法的信息
* @author bailonggang
* 2009-2-8
* 下午03:58:14
*/
public class CodeCUtil {
?
private static Logger logger=Logger.getLogger(CodeCUtil.class.getName());??
/**
? * MD5加密的過程的應用
? * @param crbyte
? * @return
? */
public static String encodeMD5(String crbyte)
{
byte[] bytes=crbyte.getBytes();
return DigestUtils.md5Hex(bytes);
}
/**
? * MD5加密的過程的應用
? * @param crbyte
? * @return
? */
public static String encodeSHA1(String crbyte)
{
byte[] bytes=crbyte.getBytes();
return DigestUtils.shaHex(bytes);
}
/**
? * 字符串的解碼過程
? * @param enbytes
? * @return
? */
?? public? static Object? decodeBase64(String enbytes)
?? {
?? Base64 base64 = new Base64();
?????? Object obj=null;
try {
obj = base64.decode(enbytes);
} catch (DecoderException e) {
????? logger.info("base64 decode ["+enbytes+"] error:"+e.getMessage());
}
??????? return obj;
?? }
?? /**
??? * base64編碼的過程的信息
??? * @param crbty
??? * @return
??? */
?? public? static String encodeBase64(String crbty)
?? {
?????? Base64 base64 = new Base64();
?????? byte[] enbytes =base64.encode(crbty.getBytes());
?????? return new String(enbytes);
?? }
?? /**
??? * 十六進制加密的過程
??? * @param enbyte
??? * @return
??? * @throws DecoderException
??? */
?? public static String encodeHex(String enbyte) throws DecoderException
?? {
?????? char[] enbytes = Hex.encodeHex(enbyte.getBytes());
?????????? return new String(enbytes);
?? }
?? /**
??? * 十六進制解密的過程
??? * @param enbytes
??? * @return
??? * @throws DecoderException
??? */
?? public static String decodeHex(String enbytes) throws DecoderException
?? {
?????? byte[] bytes=Hex.decodeHex(enbytes.toCharArray());
?????????? return new String(bytes);
?? }
?? /**
??? * Metaphone 建立出相同的key給發音相似的單字, 比 Soundex 還要準確, 但是 Metaphone
??? * 沒有固定長度, Soundex 則是固定第一個英文字加上3個數字. 這通常是用在類似音比對,
??? * 也可以用在 MP3 的軟件開發.
??? *
??? */
?? public static void languageEncoding()
?? {
?? Metaphone metaphone = new Metaphone();
?????????? RefinedSoundex refinedSoundex = new RefinedSoundex();
?????????? Soundex soundex = new Soundex();
?????????? for (int i = 0; i < 2; i++) {
?????????????? String str = (i == 0) ? "resume" : "resin";
?????????????? String mString = null;
?????????????? String rString = null;
?????????????? String sString = null;
?????????????? try {
?????????????????? mString = metaphone.encode(str);
?????????????????? rString = refinedSoundex.encode(str);
?????????????????? sString = soundex.encode(str);
?????????????? } catch (Exception ex){
?????????????????? ;
?????????????? }
?????????????? System.out.println("Original:" + str);
?????????????? System.out.println("Metaphone:" + mString);
?????????????? System.out.println("RefinedSoundex:" + rString);
?????????????? System.out.println("Soundex:" + sString + "\n");
?????????? }
?? }
}

更多文章、技術交流、商務合作、聯系博主
微信掃碼或搜索:z360901061
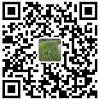
微信掃一掃加我為好友
QQ號聯系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
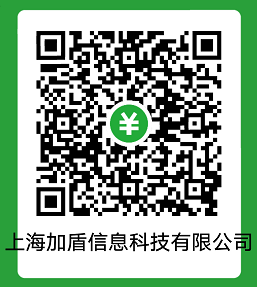