由于工作需要,改功能已測試OK!
#region 導(dǎo)入word到編輯器
?? ? ? ?//Html文件名
?? ? ? ?private string _htmlFileName;
?? ? ? ?/// <summary>
?? ? ? ?/// 上傳Word文檔
?? ? ? ?/// </summary>
?? ? ? ?/// <param name="inputFile"></param>
?? ? ? ?/// <param name="filePath"></param>
?? ? ? ?private string UpLoadFile(HtmlInputFile inputFile)
?? ? ? ?{
?? ? ? ? ? ?string fileName, fileExtension;
?? ? ? ? ? ?UploadedFile file = RadUploadContext.Current.UploadedFiles[0];
?? ? ? ? ? ?//string Path = Server.MapPath(@"Uploads");
?? ? ? ? ? ?////如果路徑不存在,則創(chuàng)建
?? ? ? ? ? ?//if (System.IO.Directory.Exists(Path) == false)
?? ? ? ? ? ?//{
?? ? ? ? ? ?// ? ?System.IO.Directory.CreateDirectory(Path);
?? ? ? ? ? ?//}
?? ? ? ? ? ?////組合路徑,file.GetName()取得文件名
?? ? ? ? ? ?//Path = Path + "/" + file.GetName().ToString();
?? ? ? ? ? ?//file.SaveAs(Path, true);
?? ? ? ? ? ?fileName = file.GetName();
?? ? ? ? ? ?//建立上傳對象
?? ? ? ? ? ?//HttpPostedFile postedFile = inputFile.PostedFile;
?? ? ? ? ? ?//fileName = System.IO.Path.GetFileName(postedFile.FileName);
?? ? ? ? ? ?fileExtension = System.IO.Path.GetExtension(fileName);
?? ? ? ? ? ?string phyPath = Server.MapPath("~/") + "Portals\\0\\WordImport\\";
?? ? ? ? ? ?//判斷路徑是否存在,若不存在則創(chuàng)建路徑
?? ? ? ? ? ?DirectoryInfo upDir = new DirectoryInfo(phyPath);
?? ? ? ? ? ?if (!upDir.Exists)
?? ? ? ? ? ?{
?? ? ? ? ? ? ? ?upDir.Create();
?? ? ? ? ? ?}
?? ? ? ? ? ?//保存文件
?? ? ? ? ? ?try
?? ? ? ? ? ?{
?? ? ? ? ? ? ? ?file.SaveAs(phyPath + fileName);
?? ? ? ? ? ?}
?? ? ? ? ? ?catch
?? ? ? ? ? ?{
?? ? ? ? ? ?}
?? ? ? ? ? ?return phyPath + fileName;
?? ? ? ?}
?? ? ? ?/// <summary>
?? ? ? ?/// word轉(zhuǎn)成html
?? ? ? ?/// </summary>
?? ? ? ?/// <param name="wordFileName"></param>
?? ? ? ?private string WordToHtml(object wordFileName)
?? ? ? ?{
?? ? ? ? ? ?//在此處放置用戶代碼以初始化頁面
?? ? ? ? ? ?ApplicationClass word = new ApplicationClass();
?? ? ? ? ? ?Type wordType = word.GetType();
?? ? ? ? ? ?Documents docs = word.Documents;
?? ? ? ? ? ?//打開文件
?? ? ? ? ? ?Type docsType = docs.GetType();
?? ? ? ? ? ?Document doc = (Document)docsType.InvokeMember("Open",
?? ? ? ? ? ?System.Reflection.BindingFlags.InvokeMethod, null, docs, new Object[] { wordFileName, true, true });
?? ? ? ? ? ?//轉(zhuǎn)換格式,另存為
?? ? ? ? ? ?Type docType = doc.GetType();
?? ? ? ? ? ?string wordSaveFileName = wordFileName.ToString();
?? ? ? ? ? ?string strSaveFileName = wordSaveFileName.Substring(0, wordSaveFileName.Length - 3) + "html";
?? ? ? ? ? ?object saveFileName = (object)strSaveFileName;
?? ? ? ? ? ?//下面是Microsoft Word 9 Object Library的寫法,如果是10,可能寫成:
?? ? ? ? ? ?/*
?? ? ? ? ? ?docType.InvokeMember("SaveAs", System.Reflection.BindingFlags.InvokeMethod,
?? ? ? ? ? ? null, doc, new object[]{saveFileName, Microsoft.Office.Interop.Word.WdSaveFormat.wdFormatFilteredHTML});
?? ? ? ? ? ?*/
?? ? ? ? ? ?///其它格式:
?? ? ? ? ? ?///wdFormatHTML
?? ? ? ? ? ?///wdFormatDocument
?? ? ? ? ? ?///wdFormatDOSText
?? ? ? ? ? ?///wdFormatDOSTextLineBreaks
?? ? ? ? ? ?///wdFormatEncodedText
?? ? ? ? ? ?///wdFormatRTF
?? ? ? ? ? ?///wdFormatTemplate
?? ? ? ? ? ?///wdFormatText
?? ? ? ? ? ?///wdFormatTextLineBreaks
?? ? ? ? ? ?///wdFormatUnicodeText
?? ? ? ? ? ?docType.InvokeMember("SaveAs", System.Reflection.BindingFlags.InvokeMethod,
?? ? ? ? ? ? null, doc, new object[] { saveFileName, WdSaveFormat.wdFormatHTML });
?? ? ? ? ? ?docType.InvokeMember("Close", System.Reflection.BindingFlags.InvokeMethod,
?? ? ? ? ? ? null, doc, null);
?? ? ? ? ? ?//退出 Word
?? ? ? ? ? ?wordType.InvokeMember("Quit", System.Reflection.BindingFlags.InvokeMethod,
?? ? ? ? ? ? null, word, null);
?? ? ? ? ? ?return saveFileName.ToString();
?? ? ? ?}
?? ? ? ?/// <summary>
?? ? ? ?/// 讀取html文件,返回字符串
?? ? ? ?/// </summary>
?? ? ? ?/// <param name="strHtmlFileName"></param>
?? ? ? ?/// <returns></returns>
?? ? ? ?private string getHtml(string strHtmlFileName)
?? ? ? ?{
?? ? ? ? ? ?System.Text.Encoding encoding = System.Text.Encoding.GetEncoding("gb2312");
?? ? ? ? ? ?StreamReader sr = new StreamReader(strHtmlFileName, encoding);
?? ? ? ? ? ?string str = sr.ReadToEnd();
?? ? ? ? ? ?sr.Close();
?? ? ? ? ? ?return str;
?? ? ? ?}
?? ? ? ?/// <summary>
?? ? ? ?///?
?? ? ? ?/// </summary>
?? ? ? ?/// <param name="strHtml"></param>
?? ? ? ?/// <returns></returns>
?? ? ? ?private void findUsedFromHtml(string strHtml, string strFileName)
?? ? ? ?{
?? ? ? ? ? ?string strStyle;
?? ? ? ? ? ?string strBody;
?? ? ? ? ? ?// stytle 部分
?? ? ? ? ? ?int index = 0;
?? ? ? ? ? ?int intStyleStart = 0;
?? ? ? ? ? ?int intStyleEnd = 0;
?? ? ? ? ? ?while (index < strHtml.Length)
?? ? ? ? ? ?{
?? ? ? ? ? ? ? ?int intStyleStartTmp = strHtml.IndexOf("<style>", index);
?? ? ? ? ? ? ? ?if (intStyleStartTmp == -1)
?? ? ? ? ? ? ? ?{
?? ? ? ? ? ? ? ? ? ?break;
?? ? ? ? ? ? ? ?}
?? ? ? ? ? ? ? ?int intContentStart = strHtml.IndexOf("<!--", intStyleStartTmp);
?? ? ? ? ? ? ? ?if (intContentStart - intStyleStartTmp == 9)
?? ? ? ? ? ? ? ?{
?? ? ? ? ? ? ? ? ? ?intStyleStart = intStyleStartTmp;
?? ? ? ? ? ? ? ? ? ?break;
?? ? ? ? ? ? ? ?}
?? ? ? ? ? ? ? ?else
?? ? ? ? ? ? ? ?{
?? ? ? ? ? ? ? ? ? ?index = intStyleStartTmp + 7;
?? ? ? ? ? ? ? ?}
?? ? ? ? ? ?}
?? ? ? ? ? ?index = 0;
?? ? ? ? ? ?while (index < strHtml.Length)
?? ? ? ? ? ?{
?? ? ? ? ? ? ? ?int intContentEndTmp = strHtml.IndexOf("-->", index);
?? ? ? ? ? ? ? ?if (intContentEndTmp == -1)
?? ? ? ? ? ? ? ?{
?? ? ? ? ? ? ? ? ? ?break;
?? ? ? ? ? ? ? ?}
?? ? ? ? ? ? ? ?int intStyleEndTmp = strHtml.IndexOf("</style>", intContentEndTmp);
?? ? ? ? ? ? ? ?if (intStyleEndTmp - intContentEndTmp == 5)
?? ? ? ? ? ? ? ?{
?? ? ? ? ? ? ? ? ? ?intStyleEnd = intStyleEndTmp;
?? ? ? ? ? ? ? ? ? ?break;
?? ? ? ? ? ? ? ?}
?? ? ? ? ? ? ? ?else
?? ? ? ? ? ? ? ?{
?? ? ? ? ? ? ? ? ? ?index = intContentEndTmp + 4;
?? ? ? ? ? ? ? ?}
?? ? ? ? ? ?}
?? ? ? ? ? ?strStyle = strHtml.Substring(intStyleStart, intStyleEnd - intStyleStart + 8);
?? ? ? ? ? ?// Body部分
?? ? ? ? ? ?int bodyStart = strHtml.IndexOf("<body");
?? ? ? ? ? ?int bodyEnd = strHtml.IndexOf("</body>");
?? ? ? ? ? ?strBody = strHtml.Substring(bodyStart, bodyEnd - bodyStart + 7);
?? ? ? ? ? ?//替換圖片地址
?? ? ? ? ? ?string fullName = strFileName.Substring(strFileName.LastIndexOf("\\") + 1);
?? ? ? ? ? ?string strOld = fullName.Replace("doc", "files");
?? ? ? ? ? ?string strNew = Page.Request.ApplicationPath + "/Portals/0/WordImport/" + strOld;
?? ? ? ? ? ?strBody = strBody.Replace(strOld, strNew);
?? ? ? ? ? ?strBody = strBody.Replace("v:imagedata", "img");
?? ? ? ? ? ?strBody = strBody.Replace("</v:imagedata>", "");
?? ? ? ? ? ?//this.TextBox1.Text = strBody;
?? ? ? ? ? ?// ?this.TextArea1.InnerText = strStyle;
?? ? ? ? ? ?this.content.Value = strStyle + strBody;
?? ? ? ?}
?? ? ? ?#endregion
更多文章、技術(shù)交流、商務(wù)合作、聯(lián)系博主
微信掃碼或搜索:z360901061
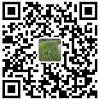
微信掃一掃加我為好友
QQ號聯(lián)系: 360901061
您的支持是博主寫作最大的動力,如果您喜歡我的文章,感覺我的文章對您有幫助,請用微信掃描下面二維碼支持博主2元、5元、10元、20元等您想捐的金額吧,狠狠點擊下面給點支持吧,站長非常感激您!手機微信長按不能支付解決辦法:請將微信支付二維碼保存到相冊,切換到微信,然后點擊微信右上角掃一掃功能,選擇支付二維碼完成支付。
【本文對您有幫助就好】元
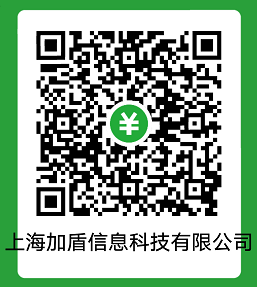